Mastering Algorithm Challenges: Techniques and Insights
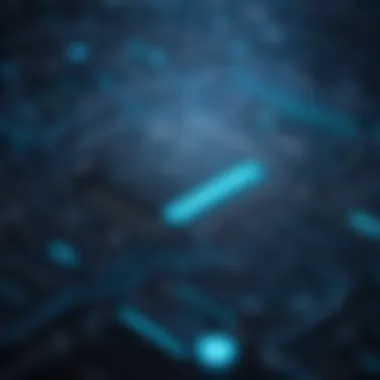
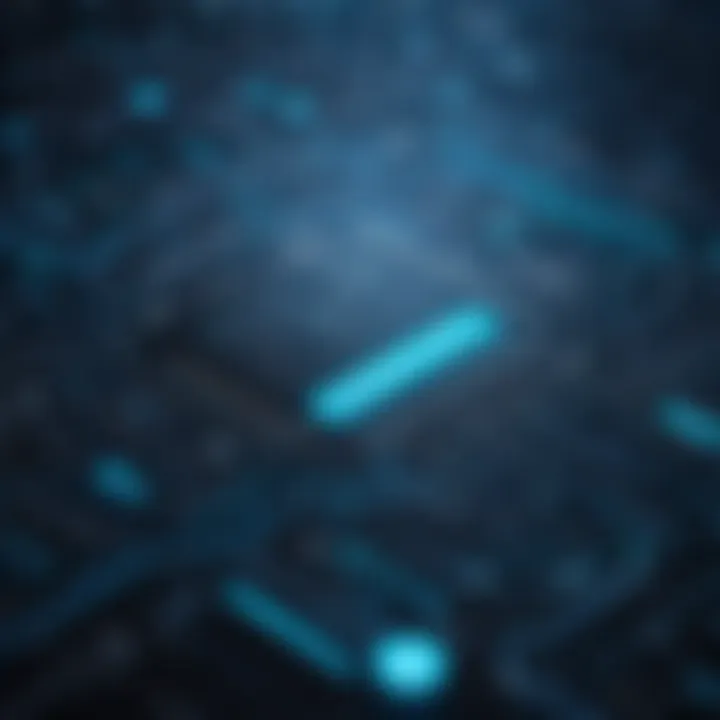
Intro
In the rapidly evolving field of computer science, algorithm problems remain a cornerstone of both academic study and practical application. Understanding these problems is crucial not only for IT professionals but also for students who aspire to navigate the intricate challenges that come with software development and data management. Algorithmic thinking enhances problem-solving capabilities, fueling innovation and efficiency in tech-related disciplines.
This article aims to peel back the layers of algorithmic challenges, focusing on key themes such as types of problems one might encounter, the various strategies for solving them, and the skills that are essential for success in the field. Whether you are a seasoned developer or a student in the making, grasping these concepts will significantly improve your proficiency in tackling algorithm-related tasks and expanding your technical repertoire.
As we get into it, remember that every algorithm problem can lead to invaluable insights. With the right approach, what seems unsolvable at first can open doors to effortless solutions. Let’s dive into the significant aspects that make up this complex yet fascinating area of study.
Prelude to Algorithm Problems
Understanding algorithm problems is akin to deciphering the code of the digital universe. Algorithm problems are not just dry exercises in logic; rather, they serve as the foundational bedrock of computer science and software engineering. They are the puzzles and challenges that programmers face daily, involving the manipulation of data, optimization of processes, and implementation of effective solutions. Grasping these problems can lead to a significant leap in one’s capabilities, setting one apart in a competitive landscape.
The ability to navigate algorithmic challenges efficiently enhances a developer's skillset considerably. It encourages critical thinking and fosters problem-solving prowess, ultimately sharpening one’s coding skills. In a world where time is money, mastering algorithms means delivering solutions that are not just correct, but also optimized for performance.
"It's not enough to just solve the problem; one has to solve it in a way that is efficient and scalable."
Defining Algorithm Problems
Algorithm problems can be defined as a set of tasks or challenges that require a systematic approach to find an optimal solution. These tasks often involve data structures, number manipulation, and pattern recognition. To put it plainly, an algorithm problem asks you to find a methodical process for resolving a question or challenge.
For example, consider the problem of determining the shortest path between two locations on a map. This isn't merely a matter of guessing; rather, it involves complex calculations, understandings such as Dijkstra's algorithm, which helps identify the most efficient routes based on various parameters like distance or time.
Importance in Software Development
In the realm of software development, algorithm problems hold immense importance and serve various crucial functions:
- Efficiency: Many applications require the handling of vast amounts of data. Efficient algorithms minimize processing time, which is vital in creating responsive software.
- Scalability: Solutions must be scalable, allowing the software to handle increased data loads without significant performance drops. Mastering algorithms is pivotal in designing systems that grow with user needs.
- Innovative Solutions: Tackling algorithm problems nurtures creativity by forcing developers to think outside the box. Finding a solution to a seemingly unsolvable challenge can lead to breakthroughs in various sectors.
Engaging with algorithm problems equips software developers with the skills to approach complex problems systematically and deliver high-quality solutions that meet the demands of modern technology. The practice of continuously solving these problems builds resilience and enhances a developer’s capabilities, also preparing them for technical interviews, which often revolve around algorithmic thinking.
Types of Algorithm Problems
Understanding the various types of algorithm problems is essential in the realm of computer science. Each category not only serves a unique purpose but also has distinct characteristics that make them suitable for solving different kinds of challenges. Grasping the intricacies of these algorithms enhances a developer's toolkit, allowing them to tackle problems more efficiently.
Among the main classifications of algorithm problems are sorting algorithms, searching algorithms, graph algorithms, dynamic programming, and backtracking algorithms. Each of these types offers various methods that can lead to optimal or satisfactory solutions based on the context of the problem at hand.
Sorting Algorithms
Sorting algorithms are fundamental in organizing data efficiently. They take a collection of items and put them in a certain order, either ascending or descending. This practice isn’t just for aesthetic purposes; sorted data significantly boosts the performance of searches and can lay the groundwork for more complex operations.
Bubble Sort
Bubble Sort stands out for its simplicity, making it an excellent starting point for understanding sorting algorithms. The main aspect of Bubble Sort is its pairwise comparison of adjacent elements. If the elements are in the wrong order, they get swapped. This process repeats until the data becomes completely sorted.
One of the key characteristics of Bubble Sort is its O(n^2) time complexity, which can render it inefficient for large data sets. However, its straightforward approach and easy implementation make it a popular choice for teaching fundamental concepts. The unique feature of Bubble Sort is that it can be optimized to stop early if no swaps occur in a complete pass, saving unnecessary iterations. But its inefficiency in handling vast data sets limits its practical applications in professional environments.
Quick Sort
Quick Sort is renowned for its efficiency and recursive nature. It works by selecting a 'pivot' element from the array and partitioning the other elements into two sub-arrays: those less than the pivot and those greater. This approach significantly reduces the amount of data that needs sorting on each recursive call.
The primary characteristic of Quick Sort lies in its average time complexity of O(n log n), making it much faster in practice compared to simpler sorts like Bubble Sort. The unique feature of Quick Sort is its in-place sorting capability, meaning it requires less memory space. However, its worst-case performance can degrade to O(n^2) if not implemented correctly, especially when the pivot choices are poor.
Merge Sort
Merge Sort processes data in a divide-and-conquer manner. This algorithm splits the data into smaller chunks, sorts these chunks independently, and then combines them in a sorted order. This systematic breakdown of data can lead to much more efficient sorting processes.
Key to Merge Sort is its consistent O(n log n) time complexity across all scenarios. This reliability makes it a favorite in situations where predictability and performance are critical. The unique aspect of Merge Sort is that it is stable, meaning that the order of equal elements is maintained, which can be essential in certain applications. However, it does require additional space proportional to the number of elements being sorted, which may be a disadvantage in memory-constrained situations.
Searching Algorithms
Searching algorithms are instrumental in retrieving data efficiently from a structured dataset. Understanding the various searching methods can drastically reduce the time complexity in applications ranging from databases to real-time systems.
Binary Search
Binary Search is a classic example of an efficient searching technique, operating on sorted arrays. This algorithm repeatedly divides the search interval in half and continues until the target value is found or the interval is empty.
The hallmark of Binary Search is its O(log n) time complexity, making it much faster than linear search methods for large datasets. Its closed interval feature allows it to quickly narrow down possibilities, though it necessitates a pre-sorted list, which is a prerequisite before its application. However, if the data is not sorted, the benefits of Binary Search quickly dissipate.
Linear Search
Linear Search is the simplest form of search, working by checking each element in the dataset sequentially until the target value is found. Although straightforward, it offers an average time complexity of O(n), making it less efficient for extensive datasets.
The key characteristic of Linear Search is its versatility. It can be applied to unsorted collections without preprocessing. However, as datasets grow, the practicality of this search method diminishes, pushing it towards rare usage in performance-critical environments.
Depth-First Search
Depth-First Search (DFS) is primarily used on graph data structures. It explores as far as possible down one path before backing up and exploring other avenues. DFS can effectively find connected components or detect cycles in graphs.
The significant aspect of DFS is its low memory usage, operating effectively with a stack data structure. Moreover, it can be implemented both recursively and iteratively, which adds versatility. However, its tendency to get trapped in deep but unfruitful paths can lead to inefficiencies, particularly in selecting appropriate paths through large datasets.
Graph Algorithms
Graph algorithms are pivotal, especially in complex systems where connections and relations matter. These algorithms help visualize connections, facilitate routing, and map relationships like social interactions or web links.
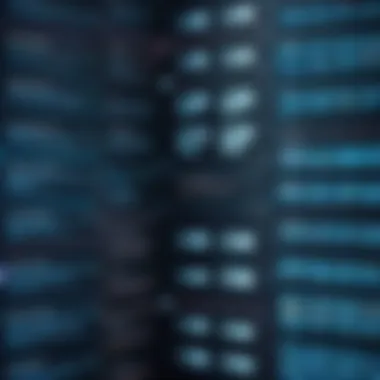
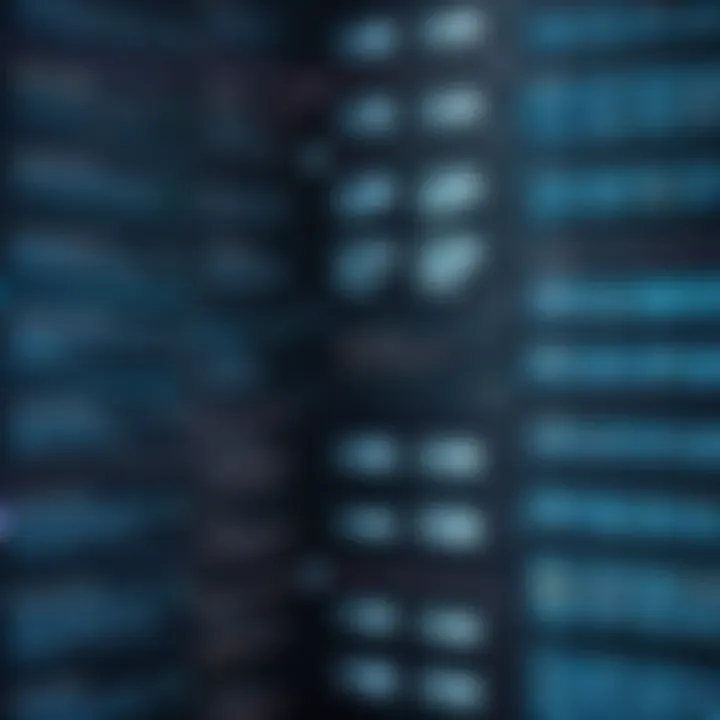
Dijkstra's Algorithm
Dijkstra's Algorithm finds the shortest path between nodes in a weighted graph. It starts from a source node and explores all connected nodes systematically, always opting for the current shortest path to the next node.
Its primary characteristic includes a time complexity of O(V^2) or better when using priority queues. The unique feature here is its ability to handle graphs with varying edge weights, making it ideal for applications like GPS navigation systems. However, it doesn’t work well with negative weights, which can be a significant downside in certain applications.
Bellman-Ford Algorithm
Bellman-Ford is another shortest-path algorithm that can handle graphs with negative weights. It operates by relaxing edges repeatedly until the optimum path to every vertex is established.
The advantage of this algorithm, particularly its O(VE) time complexity, allows it to work with a broader range of graphs compared to Dijkstra’s algorithm. A unique feature is its capability to detect negative cycles, making it quite useful in various theoretical computer science scenarios. Nevertheless, the performance disadvantage when juxtaposed against Dijkstra’s can constrain its practical application in speed-sensitive environments.
A Search
A* Search is a graph traversal and pathfinding algorithm that combines features of Dijkstra's Algorithm and heuristic-based search methods. A* evaluates paths using a cost function that includes the best-known distance from the starting node and an estimated distance to the finish.
Its major characteristic is its efficiency, providing faster pathfinding due to informed choices. The uniqueness of A* is its adaptability to various heuristics, such as the Manhattan or Euclidean distances, allowing users to tailor its use to diverse problems. However, if the heuristic function is not well-chosen, it can result in inefficient routes, affecting performance.
Dynamic Programming
Dynamic Programming (DP) deals with complex problems by breaking them down into simpler subproblems and storing their solutions, so they are not re-calculated multiple times. This technique is particularly useful where problems can be divided into overlapping subproblems, thus saving computation time.
Fibonacci Sequence
The Fibonacci Sequence is a classical illustration of dynamic programming in action. This sequence follows a specific pattern where each number is the sum of the two preceding numbers, making it a prime candidate for both recursive and iterative approaches.
The DP aspect emerges when you store previously computed Fibonacci numbers in a cache, drastically improving efficiency. The significance lies in showcasing how DP optimizes recursive solutions, turning an exponential time complexity into linear complexity through memoization. However, for smaller input sizes, the overhead may not justify the efficiency gains.
Knapsack Problem
The Knapsack Problem exemplifies optimization through dynamic programming, where various items with specific values and weights must be selected to maximize total value without breaching weight limits. This problem is notorious for its combinatorial nature but manageable through DP techniques.
By systematically exploring the decision to include or exclude each item while caching previous results, the algorithm finds an optimal solution efficiently. The main characteristic here is its ability to handle varying constraints, although large inputs can still present significant computational challenges due to growth in possible states.
Longest Common Subsequence
The Longest Common Subsequence (LCS) problem identifies the longest subsequence present in two sequences. It showcases dynamic programming’s power, breaking down the task into comparing individual elements and systematically working through all possible matches.
Highlighting its O(mn)* time complexity, LCS optimizes checks, caching interim results to facilitate future comparisons. The unique aspect, being able to find a match in unrelated sequences, opens paths in bioinformatics or text comparison, but the storage requirements can swell with larger input sequences.
Backtracking Algorithms
Backtracking is a technique that builds incrementally towards a solution by trying partial solutions and abandoning those that fail to satisfy the constraints. This method is quite powerful when navigating complex combinations and permutations.
N-Queens Problem
The N-Queens Problem is a classic case of backtracking, wherein the goal is to place N queens on an N by N chessboard without threats. The remarkable aspect of this problem is how elegantly it illustrates backtracking principles through systematic placement and backtracking once a conflict arises.
Its contributions extend beyond pure algorithmics; it offers insights into combinatorial problem solving and constraint satisfaction. However, for large N, the search space expands exponentially, leading to efficiency drawbacks.
Sudoku Solver
Solving Sudoku puzzles through backtracking is another practical application. The solver fills in numbers incrementally, ensuring compliance with Sudoku rules and retreating upon conflicts.
This problem-solving characteristic demonstrates the flexibility of backtracking techniques in a familiar context. The main limitation lies in the requirement of multiple backtrack attempts, which may not be efficient at the higher difficulty levels.
Permutations
Generating permutations of a set uses backtracking to explore all possible arrangements of the elements. The brilliance of this approach lies in its structured method of traversing through combinations without repetitions.
The unique characteristic of this problem is its capability to generate results in a time-efficient manner, presenting clear and manipulable structures. This versatility makes it relevant in computational problems involving combinations. Nevertheless, its factorial time complexity can become impractical with larger sets, leading to limited use in high-scale applications.
Understanding the various types of algorithm problems is crucial for anyone looking to navigate the complex landscape of algorithm design and implementation. With the right approach and grasp on these fundamental categories, a developer can adeptly conquer challenges that arise in software development and data analysis.
Approaches to Solve Algorithm Problems
When tackling algorithm problems, the approach taken can significantly influence both the solution's efficiency and its effectiveness. Understanding various techniques provides insight into how to navigate complex challenges. Each method offers distinct advantages and disadvantages, and professionals need to grasp these to select the best possible strategy for a given problem.
For instance, some problems lend themselves well to brute force strategies, where one exhaustively searches through all possibilities. Others may be resolved more efficiently through greedy algorithms, which make the locally optimal choice at each stage. Knowing when to apply each approach can save time and resources, enabling faster development cycles and more robust solutions.
Having a toolkit of diverse strategies fosters adaptability; software developers and data scientists can shift gears seamlessly as problem parameters change. This is especially important in fast-paced environments where requirements evolve rapidly.
Brute Force Techniques
Brute force techniques stand as the most straightforward approach to problem-solving. They involve systematically exploring all possible solutions to determine the correct one. Think of it as trying every combination of a lock until it opens. While this approach guarantees a solution, it often comes at a steep cost in terms of time and computational resources.
The main advantage here is simplicity. Implementing a brute force solution is usually quick and does not require an in-depth understanding of the problem's underlying complexities. For many problems, especially those with small input sizes, this method can yield satisfactory results.
However, the challenge becomes evident with larger data sets. As the size grows, the number of possible combinations can skyrocket, leading to impractical execution times.
Greedy Algorithms
Next up are greedy algorithms. This approach involves making a series of choices, each locally optimal with the hope that those choices will lead to a globally optimal solution. The essence of greedy algorithms is in their decision-making strategy, which often emphasizes narrow-focused efficiency over total accuracy.
For example, in tasks like Huffman coding or minimum spanning tree problems, greedy algorithms can dramatically reduce execution time while still generating suitable solutions. One could say that greedy algorithms are like a microwave oven: they provide quick results but may not always deliver the best results compared to traditional cooking methods.
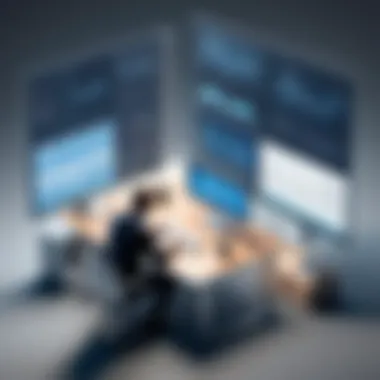
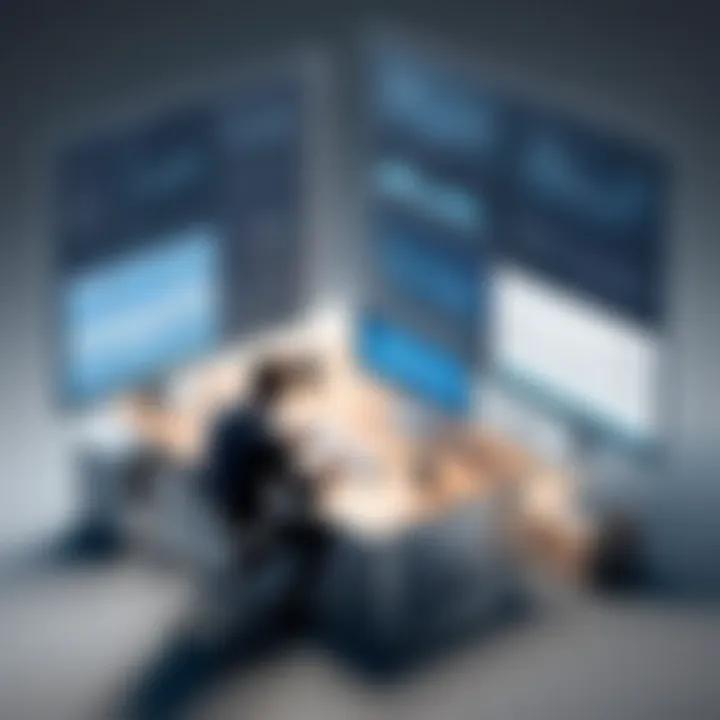
However, greedy algorithms come with their own pitfalls. Not all problems can be solved effectively with this approach—where local optimality does not equate to global optimality, greedy choices can lead to sub-par results. This is where critical analysis is necessary to determine if a greedy approach is appropriate for a specific problem.
Divide and Conquer
The divide and conquer approach involves breaking a problem into smaller subproblems, solving each one independently, and then combining their results to form a solution. Imagine cutting a large pizza into slices to make it easier to eat—it's a method designed to simplify complex issues.
This strategy shines when problems exhibit recursive properties. Classic examples include sorting algorithms such as merge sort and quicksort. First, the input is divided into smaller segments. Then, each part is processed separately, and the results are combined. This method often leads to efficient solution times, particularly for problems that can be solved in logarithmic time compared to their brute force counterparts.
That said, implementing divide and conquer solutions might require more advanced understanding, particularly when determining the merging process. It’s not always the simplest route to take, but when executed correctly, it can yield robust results.
Recursion vs Iteration
Finally, we touch on important concepts: recursion and iteration. While both terms refer to ways of organizing code to repeat processes, they each have significant implications for algorithm design.
Recursion involves a function calling itself to break down problems into manageable parts, akin to a person asking for help to climb a mountain by breaking the climb into smaller, manageable sections. This can lead to cleaner code and simpler logic, particularly for certain types of problems such as tree traversal.
However, recursion often comes with added overhead due to function call stacks, and it may not perform well for problems that require extensive iterations or very deep recursion. In contrast, iteration uses loop structures to repeat actions until a condition is met, typically leading to lower memory usage but potentially more complex code.
Ultimately, the choice of recursion or iteration should depend on the problem type and the performance requirements. Both approaches hold useful strategies for a programmer’s toolkit.
"Choosing the correct approach for an algorithm problem can differentiate between a swift solution and a drawn-out debugging session. It often pays off to understand the nuances at play."
In sum, effective problem-solving strategies in algorithm challenges necessitate a thorough understanding of various approaches. Equipped with the right techniques, IT professionals and students can devise efficient, robust solutions in tackling algorithm problems.
Skills Required for Mastering Algorithm Problems
Mastering algorithm problems is not just about understanding theoretical concepts; it’s about developing a suite of skills that work in concert to tackle complex challenges. With algorithms playing a fundamental role in software development, honing these skills becomes both essential and rewarding. It sets the stage for the successful design, implementation, and optimization of algorithms. This section will delve into core competencies that readers need to navigate the intricate world of algorithms.
Analytical Thinking
Analytical thinking serves as the backbone of problem-solving in algorithms. It’s the ability to dissect a problem into smaller, manageable parts, examine each piece, and understand how they interconnect to form the whole. This skill encourages a systematic approach, allowing one to avoid getting lost in the weeds.
A key aspect is fostering a mindset of curiosity and questioning. Why does a certain algorithm work? What assumptions are being made? By embracing this line of inquiry, individuals can develop innovative solutions that are not just correct but efficient.
"The essence of problem-solving is not merely finding answers but understanding the question fully."
Mathematical Foundations
A sharp grasp of mathematics underpins much of the work involved in algorithm problem-solving. Two notable branches are particularly important: discrete mathematics and probability theory.
Discrete Mathematics
Discrete mathematics deals with countable, distinct structures. It lays down the groundwork for various algorithms, particularly in areas like graph theory and combinatorics. The beauty of discrete math is it offers definite answers rather than shades of grey. This characteristic makes it invaluable for IT professionals designin algorithms, as clarity in logic and structure is paramount.
One unique feature of discrete mathematics is its focus on finite systems. This can be incredibly beneficial when creating algorithms for tasks that involve bounded resources or limited data sets. However, it’s worth mentioning that its applicability may wane when dealing with continuous data or complexities that require calculus or real analysis.
Probability Theory
Probability theory introduces a layer of uncertainty into mathematical reasoning. It’s essential in algorithms where outcomes are not deterministic, especially in machine learning and data analysis. Understanding probability enables developers to create algorithms that can make informed guesses rather than absolute decisions.
A standout aspect of probability theory is its ability to help in decision-making processes under uncertainty. This makes it a popular choice for fields like cybersecurity, where risks and threats are dynamically changing. The trade-off? One must balance mathematical rigor with the inherent unpredictability of this area, which can add complexity to the algorithm from a design perspective.
Programming Proficiency
Once the analytical and mathematical bases are covered, the next step is programming proficiency. This is about more than just knowing how to code; it's about understanding how to translate algorithmic ideas into functional code. Thus, selecting the appropriate programming language tailored for the task at hand becomes crucial.
Language Selection
Choosing the right programming language can significantly impact the development and performance of algorithms. Each language offers distinct advantages aligned with specific types of problems. For example, Python is favoured for its readability and extensive libraries, making it excellent for rapid prototyping and data science.
The unique characteristic of language selection lies in its trade-offs; while one language might be faster in execution, another could be more versatile with libraries. A practical understanding of which language best suits a particular scenario can save time and enhance productivity while minimizing learning curves.
Code Optimization Techniques
Once initial code is written, optimization becomes necessary. It’s about refining code and algorithms to run more efficiently, consuming fewer resources, and enhancing performance. Basic techniques include reducing complexity through better algorithms or employing data structures that improve runtime.
The standout feature of code optimization is its dual nature; while it can significantly enhance efficiency, over-optimization might lead to convoluted code that's harder to maintain. Striking a balance is essential. Ideally, having a well-optimized piece of code is a win, but maintaining clarity should not be sacrificed in the name of performance.
Overall, the skills required for mastering algorithm problems serve as pillars that uphold effective problem-solving. From analytical prowess to mathematical comprehension, coupled with solid programming capabilities, these proficiencies equip individuals to tackle challenges head-on in an evolving tech landscape.
Common Mistakes in Algorithm Problem-Solving
Identifying and recognizing common mistakes in algorithm problem-solving is essential for anyone venturing into the world of programming and computer science. The road to crafting efficient algorithms can be riddled with pitfalls that can complicate the process and lead to ineffective solutions. By understanding these missteps, programmers can not only enhance their problem-solving skills but also elevate the quality of their code. Here, we delve into three typical blunders: underestimating problem complexity, ignoring edge cases, and overcomplicating solutions.
Underestimating Problem Complexity
When faced with a new algorithm problem, one of the most frequent errors is underestimating its complexity. This often stems from a thin understanding of the underlying principles that dictate algorithm behavior and efficiency. Those who rush headlong into coding without fully grasping the requirements can find themselves in a bind, battling performance issues down the line.
For instance, consider algorithms that involve nested loops. A common oversight is assuming that these loops will execute in a linear time frame. However, if you have an algorithm with two nested loops, the time complexity jumps from O(n) to O(n²). This explosive escalation in complexity can lead to dramatically increased run times, which may render your solution inefficient for larger data sets.
"The devil is in the details" still rings true—taking a moment to analyze the problem can save a whole lot of headaches.
Ignoring Edge Cases
Ignoring edge cases is another notorious mistake that can undermine the robustness of an algorithm. Edge cases are those atypical scenarios that sit at the fringe of expected input values, often representing extremes in data. For example, when working with a sorting algorithm, it is crucial to consider what happens with an empty array or an array with just one item. Failure to account for these situations can yield unexpected results, like throwing exceptions or returning incorrect outputs.
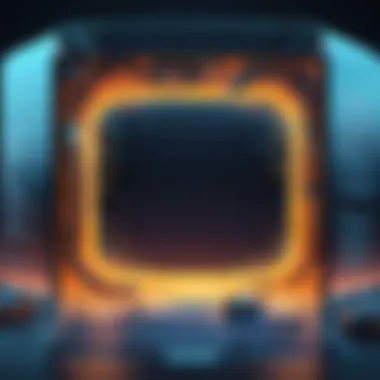
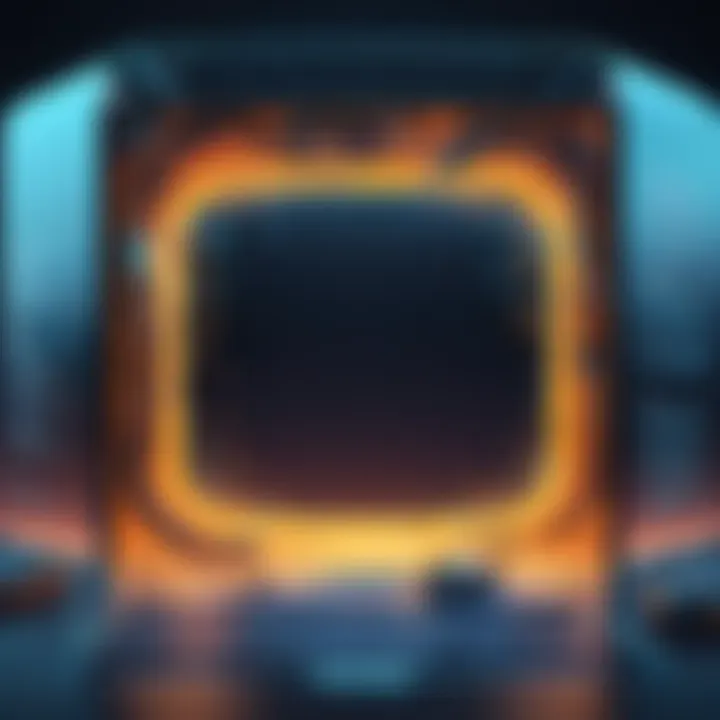
Thus, preparing for these unusual inputs is key. Ensuring that your solutions gracefully handle edge cases is not just a good practice; it is a necessity. This meticulous attention to detail can make a world of difference in real-world applications.
Overcomplicating Solutions
Sometimes, the desire to craft the most optimized or clever solution can lead to overcomplicating matters. Programmers may deploy convoluted methods that, although they may produce the correct result, can be difficult to read and maintain. Clear code is often prioritized in professional environments, as readability directly influences collaboration and future code modifications.
For instance, employing a highly intricate recursive approach for a simple problem can lead to confusion for others who may work on the code later. A fresh perspective can be aided by simpler iterations or even basic algorithmic patterns. Simple often trumps cleverness; there’s beauty in straightforward solutions that are easy for anyone to grasp.
By keeping an eye on these common mistakes, programmers can sharpen their algorithmic prowess. Instead of succumbing to the pitfalls scattered along the path, practitioners can maintain focus, ensuring they cultivate robust, efficient, and maintainable solutions.
Best Practices for Solving Algorithm Problems
When it comes to algorithm problem-solving, knowing some best practices can be the ace up your sleeve. These practices not only streamline the process but also enhance understanding and effectiveness in tackling challenges. By adhering to these practices, you’ll find that you can decode even the toughest problems, ultimately making you a more proficient coder. This section will explore three essential practices: breaking down problems, utilizing pseudocode, and engaging in iterative testing and debugging.
Breaking Down Problems
It’s easy to feel overwhelmed by an algorithm problem, especially when it seems like a tangled web of requirements and constraints. Breaking down problems into manageable parts isn’t just a nice notion—it’s a necessity. This approach allows you to focus on one piece of the puzzle at a time, taking away the pressure that often clouds thinking.
Here’s why this practice is important:
- Clarity of thought: By dissecting a problem, you clarify what’s being asked. It becomes less like a daunting monster and more like a series of friendly puzzles waiting to be solved.
- Identifying sub-problems: Many complex problems can be divided into smaller, familiar sub-problems. For instance, to sort an array, you might first need to divide the array into two halves. Recognizing these connections provides paths to known solutions.
- Incremental solutions: Working on sub-problems allows for incremental development. Once a piece works, you can integrate it with the others. Gradual building often results in fewer errors.
Utilizing Pseudocode
Pseudocode functions as a bridge between human thought and machine logic. Writing pseudocode can simplify the process of translating ideas into a formal programming language. You won't need to stuck in syntax; you can focus on the algorithm’s logic instead.
Here are some benefits of using pseudocode:
- Enhanced focus on logic: This practice helps you to flesh out the steps of your algorithm without getting bogged down in the specifics of programming syntax. The clarity gained can lead to more effective coding.
- Easier communication: If you're collaborating with others, pseudocode can be a universal language. Team members can grasp concepts without needing to know every programming language detail.
- Refinement and iteration: Writing pseudocode permits flexibility. You can easily revise steps or introduce new ideas without reworking complex code. It serves as a preliminary blueprint, saving headache later on.
Iterative Testing and Debugging
Once you’ve written your algorithm, it's time to put it through its paces. Testing iteratively is the mantra here. Rather than waiting until the very end to debug your code, a piecemeal approach can catch errors sooner, when they’re easier to fix.
Consider these factors:
- Catch issues early: Regularly testing small sections of your code can help catch errors before they snowball into bigger problems. It’s much easier to tackle a bug in a few lines of code than to sift through pages.
- Reinforced learning: By fixing individual pieces of code iteratively, the reason behind an error becomes clearer. This forms stronger connections to the underlying logic of the algorithm.
- Confidence boost: A continuous feedback loop enhances confidence. Whenever a test passes, it affirms that you’re progressing, encouraging you to keep at it.
In short, mastering algorithmic challenges hinges on adequate practices that improve efficiency and comprehension. By breaking problems down, utilizing pseudocode, and engaging in iterative testing and debugging, you’re setting yourself up for success.
Remember, each problem you solve adds to your toolkit, preparing you for the next challenge ahead.
Real-World Applications of Algorithm Problems
The integration of algorithm problems into real-world scenarios is not just an academic exercise but a fundamental element that drives various technological advancements. These problems manifest in everyday applications, affecting website performance, data integrity, and communication security. Understanding these applications aids IT professionals, cybersecurity experts, and students in grasping the significance of algorithms beyond theoretical confines.
Web Development
In the realm of web development, algorithms are the backbone of efficient coding practices. From ensuring optimal loading times to managing user interactions, algorithms like sorting and searching algorithms can greatly enhance a website's responsiveness. For instance, on e-commerce platforms, efficient sorting and filtering algorithms help users find products quickly, ultimately leading to higher sales and user satisfaction.
- Load Balancing: Algorithms distribute user requests across multiple servers, preventing overload and ensuring reliability.
- Content Delivery Networks (CDNs): They utilize algorithms to choose the best server for delivering content to users, optimizing speed and accessibility.
- SEO Optimization: Search engines use algorithms to rank web pages, impacting visibility and engagement.
A clear understanding of these algorithmic processes equips developers to make informed choices in their work, enhancing their ability to create user-centric designs.
Data Analysis
Algorithm problems play a pivotal role in data analysis, enabling the extraction of meaningful insights from vast data sets. Techniques like clustering and regression analysis make use of tailored algorithms to uncover patterns and predict trends.
Consider the following examples:
- Machine Learning: Algorithms improve decision-making processes by learning from data. This is evident in recommendation systems used by platforms like Netflix and Amazon, where user behavior dictates future suggestions.
- Predictive Analytics: Businesses utilize algorithms to forecast sales trends and consumer behavior, allowing them to adapt their strategies proactively.
- Big Data: Tools that handle large volumes of data rely heavily on algorithms for processing and analyzing information in real-time.
Understanding the algorithms behind these innovations means data analysts can refine their techniques, ensuring accuracy and relevance in their findings.
Cryptography
Cryptography is where algorithms truly prove their worth, especially in ensuring data security. With the increasing threat of cyber attacks, robust algorithms are essential for encrypting and decrypting sensitive information. The choice of algorithm can impact the security effectiveness immensely.
- Symmetric Encryption: Algorithms such as AES (Advanced Encryption Standard) enable fast and secure data encryption using a single key for both encryption and decryption.
- Asymmetric Encryption: Algorithms like RSA utilize a pair of keys, enhancing security while allowing secure communication over open channels.
- Hash Functions: These algorithms transform data into fixed-length strings, protecting integrity and preventing unauthorized data alteration.
Understanding these algorithms offers insights into safeguarding personal and organizational information, a necessity in today’s digital world.
"The application of algorithmic thinking in these contexts leads to innovations that reshape industries and improve societal function."
Finale: Advancing in Algorithmic Problem Solving
In the ever-evolving landscape of technology, the importance of advancing in algorithmic problem solving cannot be overstated. Algorithms are the backbone of efficient software solutions across multiple domains, from web development to data science and cybersecurity. This article has aimed to equip readers with a comprehensive understanding of not just the functional aspects of algorithms, but also the conceptual foundations that underlie them.
Understanding the diversity and classification of algorithm problems is crucial. Each type, whether it be sorting, searching, or dynamic programming, has specific applications and requires distinct approaches. Consequently, by grasping these principles, professionals can choose the right tools for their tasks, enhancing both productivity and creativity.
Additionally, the skills necessary for mastering these challenges have been identified. From analytical thinking to an understanding of mathematical principles, building a strong foundation paves the way for tackling complex problems effectively. This growth in skill is complemented by awareness of common pitfalls, helping individuals avoid the traps that can derail progress.
To encapsulate the journey we undertook:
Summary of Key Points
- Types of Algorithm Problems: Each algorithm serves a unique purpose. From sorting data efficiently with Quick Sort to finding the shortest path using Dijkstra's Algorithm, knowing the various types helps in selecting the appropriate method.
- Approaches to Solving Problems: Different problem-solving strategies, such as brute force or divide and conquer, provide various lenses through which to analyze and resolve algorithmic challenges.
- Skills Required: Analytical skills, a sound understanding of discrete mathematics, and programming knowledge form the trifecta essential for success in algorithm development.
- Common Mistakes: Recognizing issues such as underestimating complexity or failing to consider edge cases is vital for improving solution quality.
- Best Practices: Breaking down problems, utilizing pseudocode, and engaging in iterative testing contribute to robust solutions.
As we look toward the horizon, several Future Directions in Algorithm Research emerge:
- Machine Learning Algorithms: The integration of algorithmic processes with machine learning is gaining momentum. Researchers are focusing on refining algorithms that allow machines to learn from data more efficiently.
- Quantum Algorithms: The field of quantum computing is drawing attention. As more quantum computers become available, the need for algorithms that leverage quantum mechanics will be imperative and continues to be a rich area for exploration.
- Real-Time Problem Solving: With the growth of live data applications, algorithms that provide real-time analytics and decision-making capabilities are becoming essential. Future research may explore enhancing the speed and efficiency of such algorithms.