Mastering Pseudocode for Effective Programming Skills
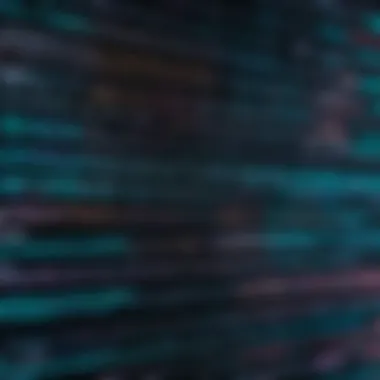
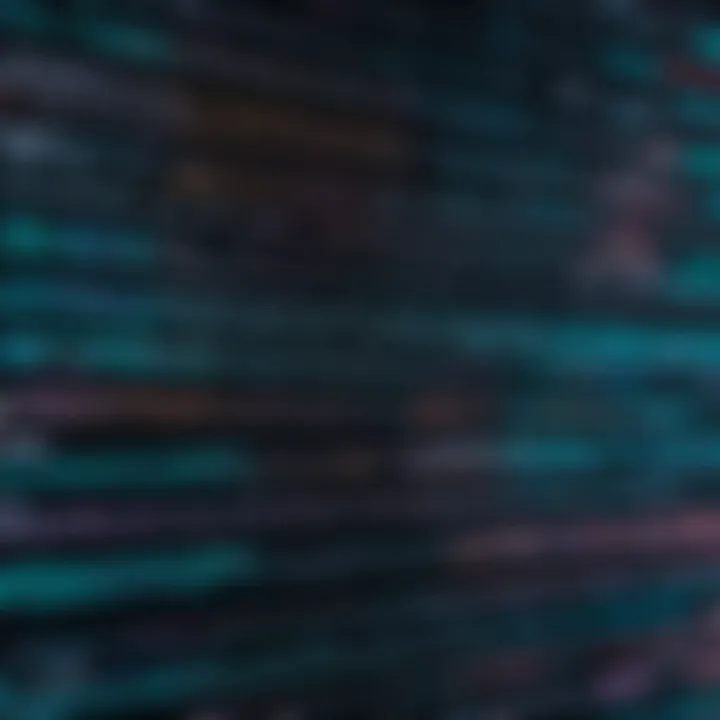
Intro
Pseudocode serves as the backbone of programming by providing a structured format to communicate algorithms clearly. It is intended to bridge the gap between raw code and human understanding. When you're learning programming or tackling complex algorithms, developing a solid grasp of pseudocode becomes essential. This article explores how mastering pseudocode sets the foundation for proficient coding practices, applicable across various programming languages.
The journey into the realm of pseudocode can seem daunting at first. However, with a well-structured approach and practical insights, anyone can navigate through its complexities. In this piece, we dissect the fundamental concepts, practical applications, and effective techniques associated with pseudocode.
Understanding Storage, Security, or Networking Concepts
Though the focus of this article remains on pseudocode, an awareness of storage, security, and networking concepts can enhance comprehension of where pseudocode fits into the broader programming landscape.
Prologue to the basics of storage, security, or networking
Knowledge of the basics of these concepts can provide context when designing algorithms that rely on these technologies. For instance, when you write pseudocode for an application, understanding how data is stored or secured impacts your algorithm's structure.
Key terminology and definitions in the field
Familiar terminology includes:
- Storage: Refers to the various types of data storage solutions, such as databases and file systems.
- Security: Involves methods to protect data integrity and confidentiality, including encryption and authentication.
- Networking: Encompasses the rules and technologies for data exchange over networks, focusing on protocols, routers, and switches.
Overview of important concepts and technologies
Each of these areas plays a significant role in programming. For example, consider that a solid understanding of networking can help a developer write more efficient algorithms for data transmission, while knowledge of security practices ensures that sensitive information remains protected.
Best Practices and Tips for Storage, Security, or Networking
Tips for optimizing storage solutions
When designing algorithms in pseudocode, consider how data will be stored and accessed:
- Opt for data structures that minimize redundancy.
- Implement caching strategies to speed up data retrieval.
Security best practices and measures
Incorporating security considerations into your algorithms early on is crucial. Best practices include:
- Always validate input to avoid vulnerabilities like SQL injections.
- Use encryption wherever sensitive data is involved.
Networking strategies for improved performance
Algorithms that involve networking should be mindful of latency and bandwidth. Here are key strategies:
- Design algorithms that optimize data processing to reduce network load.
- Use asynchronous calls where possible to enhance responsiveness.
Industry Trends and Updates
Staying updated with trends can significantly influence how pseudocode is utilized in current programming practices. Here are some noteworthy observations:
- Latest trends in storage technologies: Increasing adoption of cloud storage solutions that offer scalability and reduced costs.
- Cybersecurity threats and solutions: With the rising sophistication of cyber threats, the implementation of multi-factor authentication and end-to-end encryption becomes critical.
- Networking innovations and developments: Advancements such as software-defined networking are reshaping how networks are managed and optimized.
Case Studies and Success Stories
- Real-life examples of successful storage implementations: Companies leveraging NoSQL databases to handle big data efficiently showcase how pseudocode can articulate complex data interactions effectively.
- Cybersecurity incidents and lessons learned: Reviewing breaches in security can inform better practices in algorithm design focused on data protection.
- Networking case studies showcasing effective strategies: Real-world applications of networking protocols highlight the importance of pseudocode in planning scalable network solutions.
Reviews and Comparison of Tools and Products
Understanding tools available in the market—be it in storage or networking—can refine your approach:
- Analyzing different storage solutions, such as Amazon S3 versus Google Cloud Storage, informs optimal storage algorithms.
- Comparing cybersecurity tools, like Norton Security versus McAfee, helps understand protection algorithms.
- Evaluating networking equipment, like Cisco routers versus TP-Link, can influence networking algorithm designs.
"Mastering pseudocode not only aids in algorithm design but also empowers developers to communicate their ideas with clarity and precision."
By mastering pseudocode with a structured mindset, you become well-equipped to tackle the multifaceted programming challenges that lie ahead.
Understanding Pseudocode
Understanding pseudocode is a crucial stepping stone in the world of programming. It serves as a bridge between problem-solving concepts and the actual coding process. Think of it as the outline for a book, where the concrete details are filled in later. By grasping the basic principles of pseudocode, learners can streamline their thought processes and clarify their understanding of algorithms before they engage with more complex programming languages.
Definition and Purpose
Pseudocode can be defined as an informal language that combines the essence of programming logic with the readability of natural language. It doesn't enforce strict syntax rules like traditional programming languages. Instead, it focuses on the logic and structure behind algorithms. This flexibility allows beginners to draft their ideas without getting bogged down by syntax errors. For instance, when tasked with creating a sorting algorithm, a beginner might write:
This example clearly conveys the steps involved without the complexities introduced by Java or Python syntax.
The main purpose of pseudocode is to facilitate algorithm design and thinking. It streamlines the problem-solving process and enables programmers to focus on the logical flow of their ideas. By using pseudocode, one can formulate ideas coherently, making it an effective tool for communication among peers in the programming field. It becomes especially handy when explaining concepts to individuals who may not be familiar with specific programming languages, thus broadening accessibility.
Significance in Programming
The significance of pseudocode in programming cannot be overstated. It lays the groundwork for a smooth transition into actual coding. Here are a few key points that underline its importance:
- Simplicity: Pseudocode strips away the complexities of language syntax, making it easier to focus on logic.
- Collaborative Communication: It serves as a universal medium for coders from various backgrounds to discuss algorithms without the language barrier.
- Error Reduction: With pseudocode, one can identify logical flaws in the algorithm before implementing it in a programming language, reducing the chances of runtime errors.
"Pseudocode is like a map. It shows you where you're going without the fine print of road signs that can trip you up."
Ultimately, pseudocode is regarded as a best practice in the software development lifecycle. It’s not merely a stepping stone; it’s an essential compass guiding developers toward achieving their coding objectives effectively. Without a solid understanding of pseudocode, jumping directly into coding might lead to chaotic results and frustrating debugging sessions.
The Structure of Pseudocode
Understanding the structure of pseudocode is pivotal for anyone aspiring to become adept in programming. It acts as the skeletal framework that outlines the logical flow of a program in a way that is both general and detailed enough not to get lost in the weeds. The structure addresses what components are necessary for effective representation of algorithms, enabling clear communication of ideas before they are translated into actual code. An organized approach to pseudocode helps in reducing errors in programming, saving time and resources later in the development process.
Effective pseudocode possesses certain elements that define its clarity and functionality. These elements include basic components like variables, control structures, and data manipulations. These fundamental building blocks create a map that lays out how different parts of a coding problem interconnect, guiding you through towards the end goal. In this section, we will explore these components and conventions that elevate pseudocode from mere brainstorming to a useful tool for programming.
Basic Components
The basic components of pseudocode serve as the backbone of any pseudocode representation. Here are some key elements:
- Variables: In pseudocode, variables are placeholders that store values until they are needed. Defining them clearly is crucial since their names often convey meaning about what data they hold.
- Data Types: Although pseudocode is often abstract, recognizing data types such as integers, floats, and strings can help outline operations that can be performed.
- Operations: These often include arithmetic operations or concatenation operations that manipulate variables.
- Control Structures: Logical constructs such as loops and conditionals dictate the flow of execution within the algorithm. They are essential for building any functional program as they affect how data is processed in response to certain conditions.
When you combine these components thoughtfully, you create a structured approach that forms the foundation for deeper discussions around functionality and optimization.
Common Conventions
Common conventions in pseudocode help streamline communication and maintain consistency, particularly when multiple people are working on a project. Here are some established practices that contribute to clarity and uniformity:
- Indentation: Using indentation helps in visually organizing the code into blocks, mirroring the hierarchical nature of programming constructs like loops and conditions. It's not just for aesthetics; it clarifies logic flow.
- Keywords: Conventional keywords such as , , , , and help in denoting actions and thus standardizing the language used. Choosing the right keywords for operations simplifies the conversion from pseudocode to actual programming languages.
- Comments: A good practice in pseudocode is including comments to explain the thought process. This makes it easier for both you and others to understand the logic and intentions behind specific lines or blocks of code.
- Descriptive Naming: Using descriptive names for functions and variables enhances readability. For instance, instead of naming a variable , consider or . It paves the way for better collaboration and maintenance.
Ultimately, having these conventions in mind not only aids clarity and comprehension but also translates effectively to real-world programming.
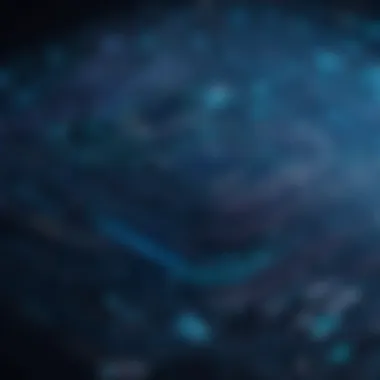
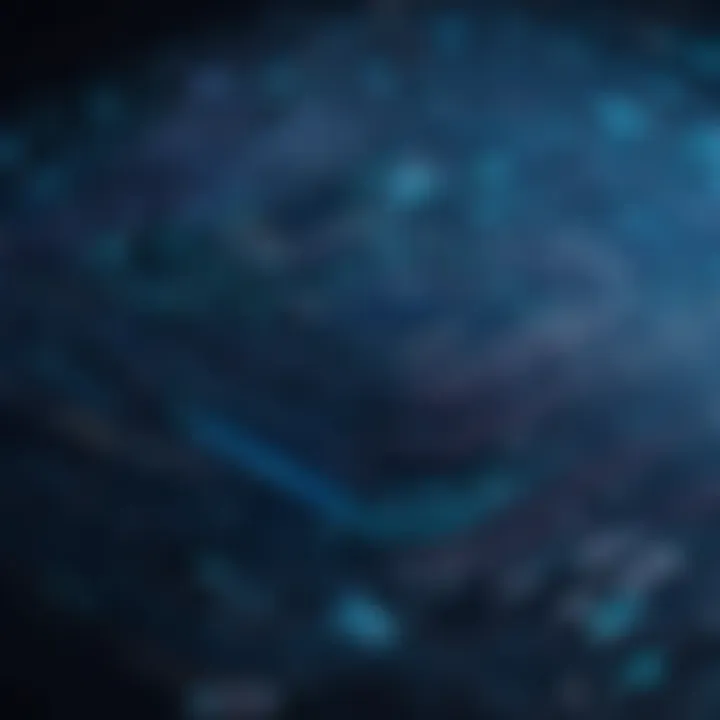
"A well-structured piece of pseudocode can save more time than a comprehensive debugging session later in coding."
Incorporating these basic components and common conventions sets a strong precedent for any pseudocode you write. Understanding these elements enhances the coding process, ensuring that when it's time to translate pseudocode into actual code, the journey is smoother and more intuitive.
Core Concepts to Grasp
Understanding core concepts is crucial when learning pseudocode, as they lay the groundwork for effective programming practices. These concepts not only help in structuring thoughts logically but also facilitate the transition to actual coding languages. It’s like having a toolbox: without the right tools, it’s difficult to build a strong framework.
Variables and Data Types
Variables are the building blocks of any programming project. They're placeholders for data that can change throughout the program's lifecycle. Knowing how to use them effectively is essential for creating any meaningful code. In pseudocode, variables are often labeled descriptively. Instead of using vague names like or , you would see something like or . This practice not only makes the code easier to read but also helps any developer understand the data's purpose at a glance.
Data types determine what kind of value can be stored in a variable. They can include integers, floats, strings, and booleans. Familiarity with data types allows the programmer to choose the right type for specific functions. For instance, if you’re storing someone’s age, it makes sense to use an integer, while text descriptions would require a string. By grasping these concepts, one builds a strong foundation that will be applicable across various programming languages.
Control Structures
Control structures give programmers the ability to dictate the flow of a program. They determine how the code will execute based on certain conditions or repeated tasks, thereby allowing for more dynamic programming.
Conditional Statements
Conditional statements, like ‘if’ statements, are vital for decision-making within the program. They allow one to execute different code based on whether a condition is true or false. This introduces flexibility. For example, a pseudocode statement might read:
The key characteristic here is its ability to branch the flow of the program. Because of this, they are favorable in many coding situations. A downside is that overly complex conditional branches can become difficult to manage and read. Keeping conditions simple is crucial to avoid confusion down the line.
Loops
Loops are another essential control structure that allows repeated execution of a block of code as long as a condition remains true. Consider a scenario where you need to process user inputs in a game. Instead of writing the input code repeatedly, a loop could easily manage this by iterating through the input requests:
The repetitive nature of loops can significantly reduce code length, making it easier to manage. The downside is that poorly defined termination conditions can lead to infinite loops, which can crash a program.
In summary, these core concepts provide the essential scaffolding upon which more advanced programming skills are built. By mastering variables, data types, and control structures like conditional statements and loops, learners can develop a more structured approach to problem-solving in programming.
Developing Problem-Solving Skills
In the realm of programming and algorithm development, honing problem-solving skills can often be the bridge between confusion and clarity. Developing these skills not only positions you to tackle complex coding challenges but also empowers you to think critically and analytically. Engaging with pseudocode actively trains your mind to dissect problems into manageable pieces and translates thoughts into logical sequences. This practice is invaluable as it prepares you to think like a programmer, an essential trait in today’s tech-driven world.
Breaking Down Problems
To effectively solve a problem, one needs to understand its components thoroughly. Breaking down problems into smaller, digestible segments is akin to slicing a hefty cake to serve at a party; it’s more palatable that way. This involves identifying the core issue and separating it from ancillary details that might cloud your judgment. By doing so, you create a clearer pathway to a solution.
For instance, if tasked with designing an application, begin by asking fundamental questions:
- What is the primary function of the app?
- Who is the intended user?
- What challenges does this app aim to solve?
By addressing these queries and segmenting the problem, you can apply pseudocode to outline a structured approach to tackle each element effectively.
Workflow Design
When it comes to workflow design, two primary approaches stand out: Top-Down and Bottom-Up. Each methodology serves its purpose depending on the complexity of the task at hand. Understanding the subtleties of these approaches can greatly influence your efficiency and effectiveness in creating solutions.
Top-Down Approach
The Top-Down Approach is characterized by breaking down a system into smaller components, starting from the broadest detail and progressively narrowing down into specifics. Imagine constructing a large building; one first creates the blueprint and lays down the foundation before detailing every room. This hierarchical model is beneficial as it allows programmers to focus on high-level functions before diving into intricate details.
A unique feature of the Top-Down Approach lies in its simplicity. It eases the initial phases of project planning, helping to minimize errors early on, and encourages understanding of the project’s overall vision. However, this process can sometimes lead to overlooking subtle details that may become crucial later in development.
Bottom-Up Approach
In contrast, the Bottom-Up Approach starts with the nitty-gritty components and builds up to a complete system. This methodology focuses on individual tasks or modules, assembling them stepwise into a larger framework. Think of it as piecing together a puzzle, where each piece is crucial to reveal the entire image.
The key characteristic of this approach lies in its thoroughness. By ensuring that each module works seamlessly, programmers can rest assured that the final product is built on a solid foundation. However, this can also pose challenges, such as potential integration issues if components do not align perfectly. Therefore, while the Bottom-Up Approach promotes detail-oriented coding, it could lead to time-consuming fixes during later integration stages.
Understanding when to employ the Top-Down or Bottom-Up Approach is vital in problem-solving through pseudocode, laying a structured path to find active coding solutions.
In summary, both the Top-Down and Bottom-Up methodologies present valuable frameworks for tackling programming challenges. By integrating these approaches within a workflow design, you can sharpen your problem-solving skills effectively, making you a more adept programmer.
Learning through Examples
Learning through examples serves as a bridge to understanding complex ideas by transforming abstract concepts into concrete scenarios. When learners dive into the world of pseudocode, the journey can become daunting without practical illustrations. Examples provide context, making the theoretical components more accessible and relatable. The experience gained through practical application can bolster a learner's confidence and the clarity of their understanding.
Using real-world scenarios in pseudocode helps clarify the relationship between syntax and logic. Here are some specific elements to consider:
- Clarity in Functionality: Examples can demonstrate how algorithms operate in practice. They clarify how a series of steps in pseudocode translates to actions in a programming language.
- Incremental Learning: Breaking down problems through smaller, simpler examples allows learners to grasp steps individually before tackling more complex problems.
- Reinforcement of Concepts: Repeated exposure to examples cements concepts in memory. By working through multiple examples, one can recognize patterns and common techniques used in various situations.
Simple Algorithms
Starting with simple algorithms is crucial for novices. These algorithms introduce key programming principles without overwhelming the learner with complexity. For instance, consider a basic algorithm to find the sum of all integers from 1 to N:
This algorithm illustrates important concepts:
- Initialization: Ensuring variables are set before use.
- Iteration: The concept of looping through a set of values.
- Accumulative Operations: Understanding how to modify a variable based on conditions.
By working through such examples, learners can identify common pitfalls, like failing to initialize a variable or incorrectly defining loop boundaries.
Real-World Applications
Real-world contexts further enrich the learning experience by connecting classroom knowledge to practical environments. For instance, consider a pseudocode designed for a retail management system, managing inventory. Here’s how a basic inventory check might look:
This application emphasizes:
- Decision-Making: Conditional statements play a major role in determining the behavior of the system based on the data available.
- Resource Management: Learning to apply logic that affects real-world resources teaches students accountability in programming.
By applying pseudocode to relevant scenarios, students can appreciate the vital role programming plays in everyday operations across various fields. As participants engage with each example, they emerge equipped not just with code but a mindset prepared for efficient problem-solving and strategic thinking in their future careers.
Tools and Resources for Learning
When it comes to mastering pseudocode, having the right tools and resources can make a world of difference. Just like a chef needs sharp knives and quality ingredients, budding programmers require effective materials to sharpen their skills. With the ever-changing landscape of technology, it is crucial to choose resources that not only provide foundational knowledge but also keep pace with current trends in programming.
Consider the resources available to you: there are numerous books and tutorials that offer structured paths to understanding pseudocode. These writings often exhibit a level of depth and rigor, guiding the learner step by step through the subtleties of syntax and logic. Additionally, online courses and communities have burgeoned, creating platforms for shared learning and collaborative growth. The blending of traditional education with digital avenues allows learners to soak in knowledge from various perspectives, which is invaluable in a field where paradigms shift rapidly.
- Benefits of Choosing the Right Tools
- Enhanced comprehension of core concepts
- Access to diverse viewpoints and techniques
- Opportunities for practical application through community engagement
Before diving into specific resources, it’s worth noting that not all materials are created equal. Learners should evaluate their own needs, whether they are starting from scratch or seeking to refine existing skills. Tailoring your learning journey with suitable resources increases motivation and effectiveness, which is vital as you navigate the complexities of pseudocode.
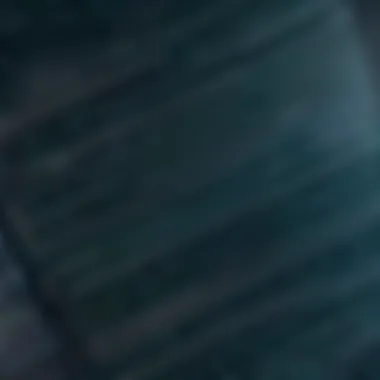
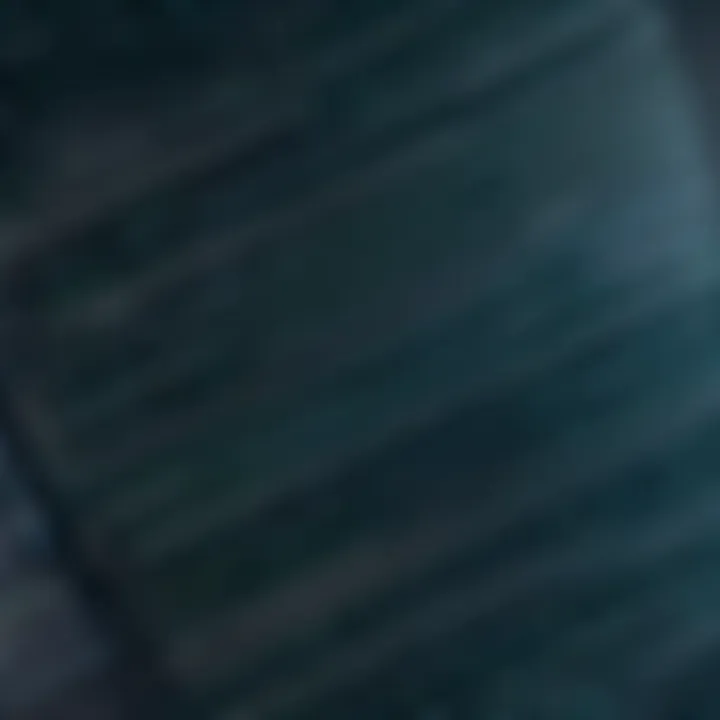
Books and Tutorials
Books and tutorials are often the bread and butter for anyone serious about learning pseudocode. They provide a structured approach to the subject, breaking down complex ideas into digestible chunks.
Some recommended texts offer guided exercises that encourage active participation. For instance, Cracking the Coding Interview by Gayle Laakmann McDowell covers many bots and algorithms, but it also emphasizes building a solid knowledge base through pseudocode. Another staple is Automate the Boring Stuff with Python by Al Sweigart, which, while centered around Python, showcases how pseudocode can simplify processes and clarify programming logic.
Here’s how to maximize the benefits from books and tutorials:
- Identify your learning style—do you prefer visual aids, extensive text, or hands-on coding examples?
- Make use of online platforms like en.wikipedia.org, which can provide supplementary information on related topics.
- Set aside regular time for reading to gradually build familiarity with terms and structures.
Practical Exercises to Hone Skills
When diving into the world of pseudocode, it's not merely about absorbing concepts and theory; it’s about rolling up your sleeves and getting your hands dirty. Practical exercises serve as the cornerstone of genuine understanding, shaping your ability to apply what you've learned in a meaningful way. Engaging in these activities not only reinforces knowledge but also cultivates problem-solving capabilities crucial in programming.
Creating Your Own Problems
One of the most effective ways to deepen your mastery of pseudocode is to design your own problems. This exercise compels you to think critically about the algorithms you want to write. You might consider a scenario, a puzzle, or even a real-life task that needs solving.
For instance, think about how to organize your day or schedule appointments. Expressing this in pseudocode can look something like:
In crafting problems from scratch, you not only get to flex your creative muscles, but you also confront challenges typical in coding. You’ll find that as you create problems, you understand nuances like edge cases and conditional logic much better.
Collaborative Projects
Another invaluable approach to enhance your understanding is to engage in collaborative projects. Working with peers exposes you to different thinking patterns and problem-solving techniques. Whether you're tackling a group assignment in class or contributing to an open-source project online, collaboration brings a wealth of knowledge to the table.
This setting allows for the sharing of ideas, which can sometimes lead to the birth of better solutions. You'll have to communicate your pseudocode and clarify your thought process to your teammates. For instance, imagine organizing a small project about developing a simple inventory management system. The pseudocode might look like:
In these collaborative environments, the feedback loop becomes essential. Someone might spot a flaw in your logic or offer a more efficient approach, enriching your learning experience. In the end, being part of a team not only enhances technical skills but also fosters soft skills like communication and teamwork.
"Learning is not a spectator sport." — D. P. Smith
Essentially, practical exercises are your best bet to transition from theory to application. Being proactive in creating problems and collaborating with others will arm you with the tools necessary to succeed in programming and beyond. Whether you are gearing up for career opportunities or tackling academic challenges, embracing these practical avenues will undoubtedly set you apart.
Avoiding Common Pitfalls
When diving into the world of pseudocode, it's all too easy to stumble into some common traps that can hinder your progress as you learn. Recognizing these pitfalls is crucial for anyone eager to master pseudocode and elevate their programming skills. Funny, how the simplest mistakes can often lead to the most significant setbacks, right? But fear not, as avoiding these missteps can save you a world of headaches down the line.
Overcomplicating Solutions
One of the primary hazards in pseudocode writing is the tendency to overcomplicate. When tackling a problem, it can be tempting to draw up a labyrinthine solution with each step intricately detailed. This might give the illusion of thoroughness, yet it often leads you straight to confusion instead of clarity.
To illustrate, let’s consider an essential task like sorting a list of numbers. A novice might delve into sorting algorithms and enumerate each step extensively, injecting unnecessary complexity. Instead, keeping it straightforward is often much more effective.
Here are key benefits of simplicity in your pseudocode:
- Clarity: Simple pseudocode is easier to read and understand.
- Focus: Stripping down to the fundamentals allows you to concentrate on the core logic without distraction.
- Efficiency: The less clutter in your solution, the easier it is to debug and adapt.
Instead of writing a complex sorting mechanism, simply stating "Sort the list of numbers in ascending order" captures the essence without overwhelming someone new to coding. Embracing simplicity doesn’t mean cutting corners; it’s abouthonesty, clarity, and respect for your future self trying to unravel the code after a month’s time.
Neglecting Testing Methods
Another glaring pitfall is failing to incorporate rigorous testing methodologies into your pseudocode development. Ideally, every algorithm should be put through its paces before being deemed fit for real-world application. The irony here is that many aspiring coders overlook testing until it's too late. They end up wrestling with bugs in actual code implementation, which could have been rectified long before.
Picture yourself writing an algorithm for a user input. If you neglect to consider edge cases—like what happens if no input is given or if it receives unexpected characters—you're setting yourself up for failure. This not only creates frustrations for end-users but also leads to a tarnished reputation as a developer.
To effectively implement testing, consider:
- Unit Tests: Write simple tests for each part of your pseudocode to ensure functionality.
- Boundary Cases: Always test the limits of your program to identify potential faults.
- Peer Review: Having another set of eyes can illuminate issues you might have missed.
Incorporating testing into your pseudocode establishes a more robust framework as you transition into real programming. It sets a solid precedent for best practices, paving the way for continued growth and resilience in a world rife with challenges.
Remember, good pseudocode not only anticipates functionality but also embraces thorough testing—it's the compass that keeps you on the right path.
Transitioning from Pseudocode to Real Programming Languages
Transitioning from pseudocode to actual programming languages is a monumental step in the journey of mastering code. It’s like taking the first leap off a diving board into a pool of endless coding possibilities. This section gears readers to grasp why this transition is crucial, not just for learning but for applying programming concepts in real-world scenarios.
When you think about pseudocode, it’s a bridge—a simplified version of an algorithm that helps in structuring thoughts without getting lost in syntax. But once you seal the deal with a solid pseudocode algorithm, it's time to get down to brass tacks and implement it in a real programming language, whether it be Python, Java, or C++. Here, understanding the mapping of pseudocode to syntax kicks in. The skills you've honed in pseudocode create a robust foundation that can boost your confidence as you navigate through different programming languages.
Mapping Pseudocode to Syntax
Mapping pseudocode to the syntax of a chosen programming language highlights the similarities and differences that exist between them. The key lies in identifying how variables, control structures, functions, and data types in pseudocode correspond to their respective counterparts in programming languages. Each language has its own quirks, but the underlying concepts mostly remain consistent.
For example, consider the following pseudocode:
In Python, you might express it as:
Knocking out the syntax of these languages takes practice, but once you familiarizze yourself with translating your pseudocode to actual code, it becomes second nature. Here are important facets to consider:
- Control Structures: Learn how conditional statements and loops function in your language of choice by mirroring your pseudocode.
- Data Types: Understand how pseudocode’s simple types translate into more complex structures in programming languages.
- Function Implementation: Note how functional definitions in pseudocode morph into actual function syntax in code.
Understanding these mappings not only minimizes the learning curve but also enhances your coding efficiency. The more proficient you become at translating your ideas, the faster you can implement complex algorithms.
Best Practices in Code Implementation
Once you've mapped out your pseudocode into a programming language, there are best practices to follow that can mean the difference between a clean, maintainable codebase and a tangled mess that you'd rather forget about. Here’s where discipline and foresight play a huge part.
- Keep It DRY (Don’t Repeat Yourself): Aim to write code that isn’t repetitive. By modularizing your code into functions, you enhance readability and maintainability.
- Consistent Naming Conventions: Use meaningful variable names that reflect the content they hold. For instance, is far clearer than .
- Commenting Wisely: Document your code with comments judiciously. Don’t bombard readers with excessive comments; use them wherever the logic requires clarity. Only explain why something is done, rather than what it does as that should be evident from well-structured code.
- Testing Your Code: Develop a habit of testing snippets as you write them, instead of waiting until the end. This helps in catching errors early.
- Version Control: Learning how to use tools like Git can elevate your programming process. You’ll gain the ability to track changes and collaborate efficiently.
By adhering to these best practices, not only will your code be more effective but also cleaner and easier to expand or debug. The transition from pseudocode to real coding signifies not just learning a language, but cultivating a professional mindset.
"An optimal implementation not only adheres to the rules of syntax but also showcases an understanding of core logic."
The journey from pseudocode to real programming languages shapes you into a thoughtful coder. So, roll up your sleeves and get ready to bring your pseudocode to life!
Evaluating Your Progress
Evaluating your progress is a cornerstone in mastering pseudocode. It’s not only about writing pseudo-instructions; it’s about refining your thought process and logical reasoning. Regular evaluation allows you to identify strengths and weaknesses, adapt your learning strategies, and ultimately become a more effective coder.
Self-Assessment Techniques
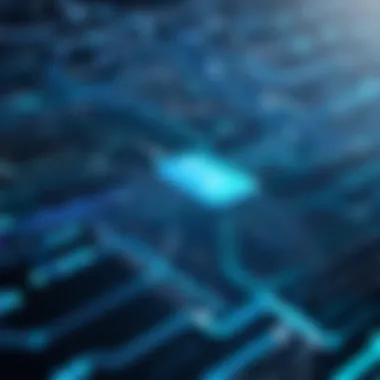
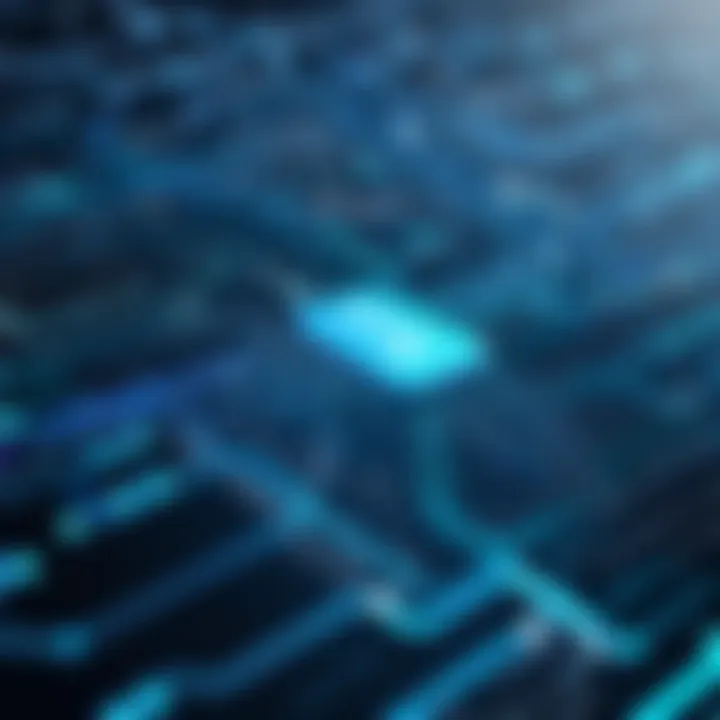
Self-assessment techniques serve as a mirror to your understanding. When you engage in self-evaluation, you take the reins of your own learning, figuring out where you stand in your journey. Here are some practical methods:
- Coding Challenges: Regularly tackle programming challenges that require pseudocode before coding. This helps you articulate your logic clearly.
- Journaling: Keep a log of problems you faced while writing pseudocode. Reflect on them, noting solutions you found and how you arrived at them.
- Concept Mapping: Create diagrams that link various pseudocode concepts. It visually represents your understanding and interconnections.
- Practice with Examples: Rewrite existing algorithms in your own pseudocode style. This exercise reinforces how well you grasp the concepts.
Utilizing these techniques isn’t merely a tick on a to-do list; it provides insight into your cognitive pathways and how effectively you can translate ideas into structured logic.
Feedback from Peers
Feedback from peers plays a vital role in honing your skills. When you share your pseudocode with others, you open the door to diverse perspectives and constructive criticism. Engaging with peers offers several benefits:
- Different Approaches: Friends may offer alternative methods you hadn't considered. This can broaden your understanding of a specific problem or concept.
- Constructive Critique: Having others review your work helps identify errors in logic or unwieldy solutions that you might overlook.
- Supportive Learning Environment: Working alongside others fosters motivation. It can be encouraging to see your peers tackle similar challenges and share their learning experiences.
- Building a Network: Interacting with others in the field can lead to valuable connections that might assist you in your future endeavors.
Getting feedback is about fostering a collaborative spirit. Engaging in discussion encourages not only personal growth but also contributes to collective knowledge.
"The greatest teacher, failure is."
Together, self-assessment and peer feedback create an ecosystem of continuous learning, pushing you to evolve beyond mere basics into the realm of deeper understanding and application.
Applying Pseudocode in Industry
Pseudocode plays a pivotal role in bridging the gap between abstract programming concepts and practical application in industry settings. Its clarity and straightforwardness make it an essential tool for IT professionals, cybersecurity experts, and students alike. By using pseudocode, teams can effectively communicate complex ideas and algorithms, ensuring everyone is on the same page before diving into actual code. This approach reduces miscommunication, enhances collaboration, and ultimately helps in the delivery of robust software solutions.
The ability to translate concepts into a readable format is invaluable for various industry practices. For instance, it aids in documentation, allowing for a shared understanding among team members of different technical backgrounds. To drive home its significance, let's explore a couple of key aspects in detail.
Case Studies
In many successful tech projects, applying pseudocode has proven to streamline and enhance decision-making processes. Consider the case of a financial institution developing an algorithm for fraud detection. The team first created pseudocode to outline the flow of operations, detailing each step involved in evaluating transaction patterns. This enabled them to spot potential inefficiencies or logical gaps early in the development lifecycle.
Similarly, a health tech startup designed a telemedicine platform using pseudocode to map out user interactions. By visualizing the user journey and interactions, they managed to create a seamless experience for patients. Such case studies highlight not only the practical applications of pseudocode but also its ability to foresee challenges which may arise later in the coding phase.
Integration in Software Development Lifecycles
Integrating pseudocode into the software development lifecycle (SDLC) is essential for the success of modern development practices. Whether employing Agile, Waterfall, or DevOps methodologies, pseudocode acts as a foundational element in several phases:
- Planning: Teams can specify project requirements and functionalities through pseudocode, delineating the expected outcomes intuitively.
- Design: During the design phase, pseudocode helps to develop relevant algorithms, providing a guideline for system architecture and component interaction.
- Implementation: As developers translate pseudocode into programming languages, they often discover inconsistencies that require addressing beforehand. This step minimizes alignment issues later on.
- Testing: Pseudocode can also serve in developing test cases, ensuring that all designated scenarios are addressed.
In summary, applying pseudocode within the industry is more than just a learning tool; it is a catalyst that promotes clarity and efficiency throughout the entire SDLC. By adopting this structured approach, organizations can streamline their development processes, foster effective communication among teams, and enhance the overall quality of their software solutions.
"By leveraging pseudocode, teams not only bridge communication gaps but also set a sturdy framework for successful software development."
Expanding Knowledge Beyond Pseudocode
In the realm of programming, mastering pseudocode serves as a vital stepping stone. However, it is crucial to realize that this knowledge shouldn't stop there. Breaching the boundaries of pseudocode can significantly enhance your coding prowess and overall understanding of algorithms.
By delving deeper into the interconnected world of algorithm analysis and data structures, you position yourself to tackle more complex challenges effectively. In this part, we will explore both algorithm analysis techniques and the understanding of data structures. Together, these areas form the backbone of programming competency, enabling a more complete grasp of how to translate ideas into working code.
Algorithm Analysis Techniques
Algorithm analysis is like a compass for programmers, guiding them through the vast sea of potential solutions. When you're knee-deep in code, evaluating its efficiency can mean the difference between an application that runs in seconds versus one that takes ages. Understanding the time complexity and space complexity of algorithms aids in determining the feasibility and performance.
Several techniques are employed in algorithm analysis:
- Big O Notation: This is the most common method to express the upper bound for an algorithm's execution time. Grasping Big O gives insight into how the runtime increases with input size.
- Best, Worst, and Average Cases: Learning to differentiate between these cases can help in estimating how algorithms will perform under various conditions.
- Amortized Analysis: This technique provides a way to analyze the average time per operation over a sequence of operations, focusing on long-term performance rather than isolated instances.
Considering these techniques can reveal subtle efficiencies and drawbacks in your algorithms, leading to smarter programming solutions.
Understanding Data Structures
Data structures are the containers of your data management efforts. To handle programming problems efficiently, knowledge of different types of data structures—from arrays and lists to trees and graphs—becomes imperatively important. Each structure has its pros and cons; knowing them can shape your problem-solving techniques.
A few fundamental data structures to consider include:
- Arrays: Simple and fast for indexed access but inflexible due to fixed size.
- Linked Lists: These provide flexibility in size but lack the speed of arrays for indexed access.
- Trees: Useful for hierarchical data, trees facilitate organized searching and retrieval.
- Graphs: Essential for representing networks, understanding graphs is critical in fields such as cybersecurity and artificial intelligence.
By grasping these concepts, you can analyze the most effective approach to store and manipulate data, thus sharpening your code performance.
"Understanding both algorithm analysis and data structures is paramount as it empowers a coder to create elegant solutions, effectively merging creativity with logic."
In the end, expanding your knowledge beyond pseudocode allows you to become a more versatile programmer. Each layer of understanding not only builds on the previous one but also opens doors to innovative solutions in software development, enhancing your chances for success in this evolving field.
Building a Personal Learning Path
Creating a personal learning path is a crucial step for anyone setting out to master pseudocode. This concept isn’t just a roadmap; it’s a customizable journey tailored to individual needs, learning styles, and professional aspirations. By outlining specific learning objectives and continuously evolving these goals, learners can navigate the complexities of coding more effectively.
Setting Objectives
When establishing a personal learning path, the first thing to do is set clear, achievable objectives. Think about where you want to land in the world of programming. Are you aiming to crack the nuts and bolts behind web development or delve deeper into data science? Identifying your interests can help channel your efforts in the right direction.
- Be Specific: Instead of saying, "I want to learn programming," fine-tune your goals. Something like, "I want to understand loops and algorithms by the end of this month" gives you a clear target.
- Measurable Outcomes: Consider keeping track of what you learn. After working on algorithm exercises for a week, you might say, "I can now write pseudocode for a binary search."
- Realistic Expectations: Steer clear from overly ambitious goals. It’s better to start small and gradually tackle more complex concepts. This will not only keep you motivated but will also reinforce your understanding.
Bringing structure to your learning objectives can translate into a more fruitful experience. As a learner, making adjustments along the way is inevitable. Don't hesitate to revise your goals based on the progress you make.
Continuous Learning Strategies
The tech world is like a fast-moving train, and for this reason, continuous learning strategies must be part of your personal learning path. Here’s how to ensure you keep up with that relentless pace.
- Stay Curious: Treat every new concept as an opportunity to grow. If you learn about conditional statements, for instance, challenge yourself to implement them in a real-life scenario. This active engagement helps cement your understanding.
- Join Communities: Participating in forums like reddit.com or engaging in discussions on platforms such as Facebook can expose you to different perspectives and problem-solving approaches. Connecting with fellow learners often uncovers nuggets of knowledge that literature alone may not provide.
- Utilize Online Resources: Websites like en.wikipedia.org and britannica.com can offer foundational insights as you supplement your learning with hands-on coding.
- Reflect on Your Learning: Regularly take time to reflect on what you’ve learned. Journaling or blogging about your journey can deepen your understanding and help in identifying areas where you need improvement.
The goal isn’t to become the fastest coder, but the most effective thinker.
In summary, building a personal learning path in pseudocode is about being deliberate and strategic. With set objectives and ongoing learning strategies, you’re not just preparing yourself for today's challenges but also laying a solid foundation for the future of your coding career.
Closure: The Road Ahead
As we reach the conclusion of this exploration into pseudocode, it’s vital to reflect on the journey we've taken and the pathways that lie ahead. Pseudocode is not just a pedagogical tool; it’s a bridge to understanding complex programming concepts. As you have seen, it enables the breakdown of intricate algorithms into manageable chunks. Mastering pseudocode equips you with unique skills that will not only enhance your programming abilities but can also bolster your problem-solving approaches across diverse fields.
Reflections on Learning Pseudocode
Learning pseudocode instills a certain confidence in your coding abilities. When programming languages can feel as convoluted as ancient script, pseudocode offers clarity. It’s like having a roadmap before embarking on a long journey. You get to see the landscape of your logic without the distractions of syntax errors or language-specific rules.
Reflecting on the strategies discussed, such as breaking down problems or utilizing structured designs, one can appreciate how these methods foster logical thinking. Start small - perhaps with elementary algorithms - and gradually build complexity as your understanding deepens. The beauty of this method lies in its adaptability; whether you are a computer science student or an experienced developer, there’s always more to learn. Remember:
- Practice frequently with various algorithms
- Engage closely with communities for feedback and insights
- Be persistent in your learning endeavor
Connecting Theory to Practice
Linking theoretical knowledge to practical applications is where many learners often struggle. In our context, understanding pseudocode sets the foundation for realizing its real-world usage. Applications in software development, data analysis, and even cybersecurity are plentiful. When facing a task, envision it in pseudocode before diving into actual programming. This method clarifies your thought process and reduces the cognitive load when transitioning into a specific language syntax.
In managing projects, consider how pseudocode can outline workflow efficiently. This could range from algorithm creation to debugging existing code.
"The ability to see the bigger picture while working through details is what turns a good coder into a great one."
Moreover, as industries evolve, ongoing practice in converting ideas into pseudocode becomes increasingly invaluable. Tools like GitHub and collaborative forums provide a platform to engage with others’ projects, test your skills, and reinforce your learning.
Lastly, always be open to feedback, both to your pseudocode and your eventual programming. Surrounding yourself with a network of peers helps in reinforcing your learning path. This iterative process will bring about growth as a coder capable of tackling real-world challenges efficiently.
In essence, the road ahead involves enduring commitment and the continuous integration of pseudocode into your programming repertoire. Keep that curiosity alive, and remember—every accomplished coder started where you are right now.