Programming Android Applications: A Comprehensive Guide
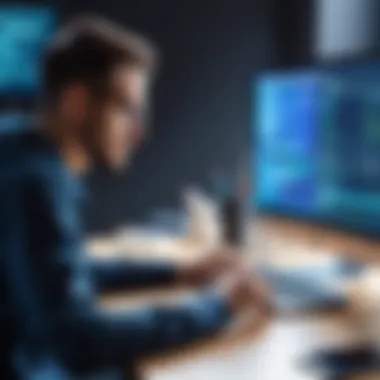
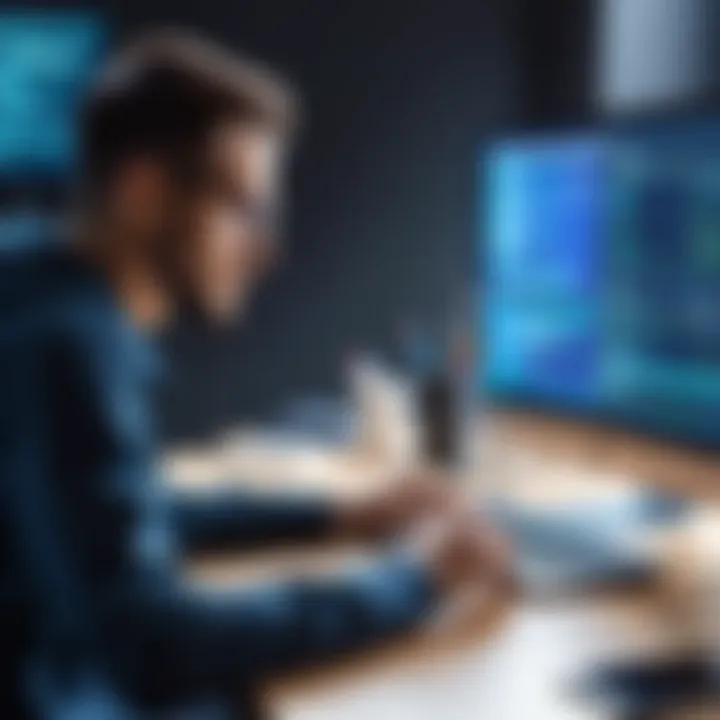
Intro
Creating applications for Android is more than just learning a programming language; it's about understanding the entire ecosystem. This guide dives deep into the nuances of bringing an Android app from concept to reality. Let's take this journey, exploring the essential tools, practices, and trends that shape this exciting field.
Understanding Storage, Security, and Networking Concepts
Foreword to the basics of storage, security, and networking
When stepping into the world of Android app development, the significance of efficient storage, robust security, and effective networking can't be overstated. These elements serve as the foundations upon which your applications are built. Understanding these concepts ensures not only smoother performance but also safeguards user data.
Key terminology and definitions in the field
To navigate through storage, security, and networking in Android programming, familiarity with the terminology is key. Here are some essential terms:
- API (Application Programming Interface): A set of tools that allows different software programs to communicate with one another.
- SQLite: A lightweight database engine commonly used on Android for local data storage.
- Encryption: The process of converting data into a coded format, making it accessible only to those with the right decryption key.
- HTTP/HTTPS: Protocols used for transferring data over the web, with HTTPS providing a secure connection.
- Bandwidth: The amount of data that can be transmitted in a given time frame over a network.
Overview of important concepts and technologies
Storage Technologies
Understanding the different storage options available for Android apps is vital. Whether it's using device storage, cloud solutions, or databases, each method serves different use cases. For instance, SQLite might suit smaller apps, while Firebase might be optimal for real-time data exchange.
Security Technologies
Android provides several layers of security, from application-level protections to hardware-backed security through Trusted Execution Environments (TEE). Developers must incorporate measures like Secure Sockets Layer (SSL) for data transmission and use secure coding practices.
Networking Technologies
In a connected world, effectively managing network resources becomes crucial. Techniques such as caching, lazy loading, and data throttling can greatly improve an app’s performance and decrease latency.
Best Practices and Tips for Storage, Security, and Networking
Tips for optimizing storage solutions
- Utilize the right data storage: Choose between internal and external storage based on the nature and sensitivity of the data.
- Implement data compression: Improve app performance and reduce storage needs by efficiently compressing data before saving it.
- Cleanup unused data: Regularly clear out cookies, cache, and unused files to keep your app running smoothly.
Security best practices and measures
- Regularly update libraries: Ensure your app remains secure by using the latest versions of frameworks and libraries.
- User authentication: Implement strong authentication protocols, including OAuth or biometric verification.
- Secure data transmission: Always use HTTPS instead of HTTP to protect data in transit.
Networking strategies for improved performance
- Optimize API calls: Reduce the number of API calls and consolidate requests when possible to minimize server load.
- Use background services wisely: Take advantage of Android’s work manager to handle background tasks efficiently without draining system resources.
Industry Trends and Updates
Latest trends in storage technologies
The trend is shifting towards cloud computing, with tools like Google Cloud Platform leading the way. Developers are increasingly adopting NoSQL databases such as Firestore for their flexibility and scalability.
Cybersecurity threats and solutions
As the usage of mobile apps grows, so do the risks associated with cybersecurity. Utilizing machine learning algorithms for threat detection and adopting multi-factor authentication are becoming key strategies in safeguarding applications.
Networking innovations and developments
Technologies like 5G are revolutionizing the way apps connect and interact. With improved bandwidth and lower latency, apps can offer instantaneous responses, enhancing the user experience significantly.
Case Studies and Success Stories
Real-life examples of successful storage implementations
Businesses have turned to cloud solutions to manage user data effectively—one notable example is Spotify, which leverages cloud technology efficiently for its immense music library.
Cybersecurity incidents and lessons learned
The infamous breach of Equifax in 2017 serves as a stark reminder of the importance of fortifying cybersecurity measures. This incident pushed organizations to rethink their data handling practices and increase transparency with users.
Networking case studies showcasing effective strategies
Take the ride-sharing giant Uber as an example. Their innovative use of real-time data processing and adaptive networking capabilities enables them to connect drivers with riders seamlessly, optimizing service delivery.
Reviews and Comparison of Tools and Products
In-depth reviews of storage software and hardware
Options such as Firebase Realtime Database and MongoDB offer unique advantages for app developers, with Firebase focusing on real-time synchronizations and MongoDB offering flexibility in data structure.
Comparison of cybersecurity tools and solutions
Tools like OWASP ZAP and Nessus provide vital security assessment capabilities, enabling developers to scan and identify vulnerabilities early in the development cycle, therefore preventing potential breaches.
Evaluation of networking equipment and services
Emerging networking technologies like Mesh routers are being reviewed for their ability to provide seamless connectivity in homes and businesses alike, adapting to user demands effectively.
By understanding how storage, security, and networking intertwine, one can better navigate the complex landscape of Android app development.
Understanding Android Development
Understanding Android development is paramount in the realm of mobile technology. Given the ubiquity of smartphones in our daily lives, it becomes evident that mastering the principles of Android development is essential for anyone looking to create impactful mobile applications. This section aims to clarify key elements that make Android development not just a technical skill but a crucial part of our digital ecosystem.
What is Android?
Android is an open-source operating system primarily designed for mobile devices like smartphones and tablets. Developed by Google, it provides a platform for application developers to create software that runs seamlessly on a variety of hardware. The operating system is built on a modified version of the Linux kernel, featuring a substantial Java library. Its flexibility is one of the reasons Android has captured a significant share of the global mobile market.
The community around Android is vast and diverse, encompassing hobbyists and seasoned developers alike. Notably, applications can be developed in various programming languages, including Java and Kotlin, giving developers the freedom to choose tools they are comfortable with. Furthermore, the Android Software Development Kit (SDK) offers a rich set of tools to create various app functionalities, catering to an expansive audience.
Importance of Mobile Apps
Mobile apps are more than just conveniences; they have reshaped how businesses operate, how information is accessed, and how social interactions occur. Here are some key reasons why mobile applications are so vital:
- User Engagement: Mobile apps tend to foster greater engagement through notifications and personalized experiences. They allow companies to connect with customers directly on their devices, significantly enhancing user retention.
- Accessibility: With an intuitive interface designed for mobile users, apps make accessing services and products a breeze. Businesses that utilize mobile apps often find they can serve customers more effectively.
- Data Collection: Mobile applications help companies gather valuable data about user behavior, which can be utilized for improving services and targeting marketing efforts more precisely.
- Brand Visibility: In a world where everyone has their phone glued to their hand, mobile apps increase brand visibility. An app on a user’s device acts as a constant reminder of the brand, even during off-hours.
- Revenue Generation: For businesses, mobile apps can serve as new revenue streams, whether through direct sales, subscriptions, or advertisements.
"In a digital age, understanding how to develop mobile applications is not merely an asset; it's a necessity."
As we delve deeper into the complexities of Android development, it will become clear how imperative it is to grasp these foundational concepts. The ability to create effective and engaging mobile applications can give you an edge in this competitive landscape. Thus, gaining a thorough understanding of Android development is crucial, not just as a learning opportunity but as a stepping stone into a thriving market.
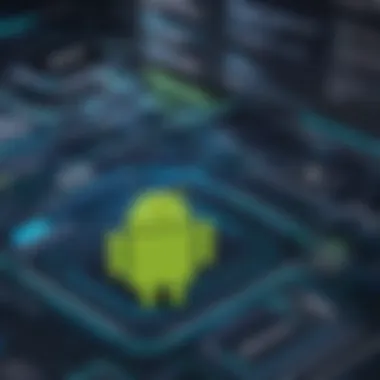
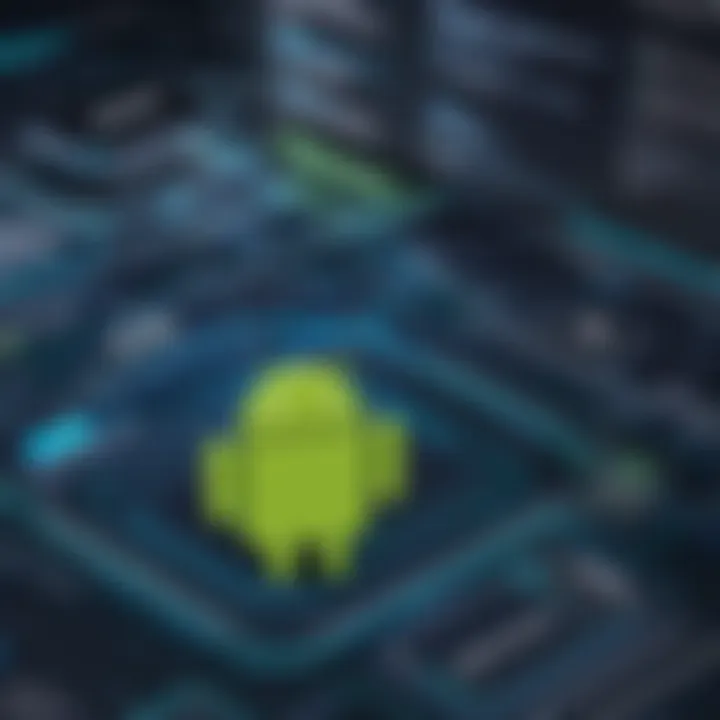
Setting Up the Development Environment
Setting up the development environment is an indispensable first step in the journey of developing Android applications. This phase forms the bedrock upon which every project stands or falls. A well-configured environment is crucial for smooth coding, debugging, and testing. Without the right tools and settings in place, developers may find themselves wrestling with issues that could have been avoided entirely.
An optimized setup allows for increased efficiency. Developers can focus on writing code rather than troubleshooting configuration problems. Furthermore, it fosters a conducive atmosphere, where creativity and technicality can merge seamlessly. Ensuring the development environment is tailored to individual preferences and project requirements can markedly enhance productivity.
Installing Android Studio
Installing Android Studio is like laying the foundation of a house. You wouldn't build on sand, would you? This Integrated Development Environment (IDE) is the official platform for Android development and is packed with features that cater to both rookie programmers and seasoned experts. To get started, you'll need to download the IDE from the official Android Developers website.
Once you get the installer, it’s essential to follow these steps meticulously:
- Run the Installer: After downloading, double-click the installer file. The setup wizard will guide you through a series of configurations.
- Choose Installation Type: You can go with the standard setup, which installs essential components or opt for a custom installation if you have specific preferences.
- Set SDK Location: The Software Development Kit (SDK) location is critical as it provides necessary tools to develop apps. Make sure to note this path.
- Complete the Installation: After setting everything, hit the finish button, and voila! You're good to go.
Once Android Studio is up and running, take a moment to explore its layout. Familiarize yourself with the different menus and tools. This will save you precious time down the line.
Choosing the Right Emulator
While a physical device can give a tactile testing experience, not everyone has access to multiple phones or tablets. Here’s where the emulator comes into play. Choosing the right emulator is like picking the right vehicle for a road trip; it can make or break your experience.
The Android Emulator is bundled with Android Studio, allowing developers to run apps on various Android devices virtualized on their computer. Things to consider include:
- Performance: Some emulators are resource hogs, impacting the overall performance of your system. Look for lightweight options that won't bog down your development process.
- Device Variety: Opt for emulators that offer a wide range of devices to test different screen sizes, resolutions, and Android versions.
- Features: Advanced features like GPS simulation, camera integration, or network latency can help in thorough testing.
Here are a few popular emulator choices:
- Genymotion: Known for its speed and multiple device support, this option is favored by many developers.
- Android Virtual Device: Built within Android Studio, it's simple to set up and offers extensive device configurations.
- Bluestacks: More geared towards gaming, but still a solid choice for general app testing.
Selecting the right emulator can save time in the testing process, leading to a smoother development experience overall.
"Your development environment can either bring synergy to your programming experience or hinder it significantly. Choose wisely."
In summary, laying a solid groundwork by setting up your development environment correctly is pivotal. It is the first tangible step toward building your Android application and sets the tone for the development process.
Programming Languages for Android
When it comes to developing Android applications, the choice of programming language plays a crucial role. It's not just about syntax; it's about compatibility, efficiency, and the ability to leverage native features of the Android platform. The popularity of Android as a mobile operating system is directly tied to the programming languages utilized to create applications. Therefore, understanding these languages is fundamental for developers at any level.
The two primary languages for Android development are Java and Kotlin. Each has its distinct characteristics, advantages, and drawbacks that can affect the development process. Let’s break down these languages and highlight their importance.
Java in Android Development
Java has been the cornerstone of Android development since the platform's inception. It's a well-established language, known for its robustness and performance. Developers often recognize its comprehensive libraries and tools that facilitate everything from basic application structure to complex functionalities.
Advantages of Java in Android Development:
- Stability: The extensive use of Java ensures that many resources, forums, and troubleshooting options are available, helping developers solve issues quickly.
- Memory Management: Java's garbage collection feature is a boon for managing memory without requiring developers to constantly track allocations and deallocations.
- Community Support: As a mature language, Java has a vast community. There's a plethora of documentation, tutorials, and third-party libraries that make development more streamlined.
However, it’s worth noting that Java can be somewhat verbose compared to newer languages. This verbosity can lead to increased development time and complexity in coding, especially for tasks that require frequent code updates.
Kotlin: The Modern Language
Introduced officially for Android development in 2017, Kotlin has rapidly gained traction. Many developers appreciate its modern approach, as it addresses some of Java’s limitations while maintaining full interoperability with Java code. Kotlin's syntax is more concise, which often translates to fewer lines of code and lower chances for bugs.
Highlights of Kotlin:
- Conciseness: One of Kotlin's strong points is its ability to express ideas clearly in fewer lines compared to Java, helping to improve code readability.
- Null Safety: Kotlin’s design incorporates null safety into the language—a feature that significantly reduces the risk of null pointer exceptions, a common headache for Java developers.
- Functional Programming Features: Kotlin supports many functional programming concepts, allowing developers to write clean and efficient code that is often easier to maintain.
Both languages hold their weight in Android development, but if you aim for rapid development cycles and cleaner code, Kotlin often becomes the preferred choice.
"Choosing the right programming language can make all the difference between a smooth development experience and one filled with roadblocks."
In the end, the effective use of either Java or Kotlin hinges on project requirements, team expertise, and future scalability considerations. As Android continues to evolve, staying updated with these programming languages becomes essential for any developer aiming to succeed in the fast-paced world of mobile applications.
Core Components of Android Applications
The core components of Android applications play a pivotal role in defining how apps are structured and how they interact with users and each other. Understanding these components is crucial for any developer looking to create efficient and effective applications. These components serve as building blocks, each with its specific function and life cycle. Knowing how to leverage them properly can markedly enhance the functionality and user experience of an app. Whether you are building a simple task manager or a sophisticated e-commerce platform, getting a grasp on these core players is non-negotiable. They not only improve application performance but also ensure that apps are reliable and user-centric.
Activities and Intents
Activities are essential for presenting a user interface in Android applications. Think of an Activity as a single screen with a user interface that allows users to interact with the app. Each time a user interacts with an app, they’re typically dealing with an Activity. For instance, when you open a messaging app and view a conversation, the screen you see is an Activity.
Each Activity has its own life cycle. A developer must navigate through the activity's life cycle, such as onCreate(), onStart(), onResume(), and so on. Understanding these methods ensures that resources are efficiently managed and the user’s experience is fluid. Activities can also launch other Activities through Intents, which are messages that facilitate communication between different components in an Android device. For example, an Intent can be triggered to open a gallery Activity when a user wants to attach an image in a chat.
Services: Running Operations in the Background
Services are another crucial component aimed at running long-lasting operations in the background without a user interface. They are particularly handy for tasks like keeping music playing while a user navigates through different screens or handling network operations without crashing the app’s graphical user interface.
In essence, there are three types of services: Started Services, Bound Services, and Intent Services. For example, a Started Service could download a file while the user continues to use the app for other tasks. These services remain active even if the user moves to a different Activity. It's essential to handle services properly, especially when managing resources, to prevent battery drain and improve performance.
Broadcast Receivers: Listening for Events
Broadcast Receivers serve as a communication mechanism within the Android operating system. They allow an application to listen for specific events that occur in the system or another app, such as incoming SMS messages, changes in network connectivity, or battery status updates.
When a specific event happens, the Broadcast Receiver gets activated and can execute a corresponding action. For instance, let's say a user receives a text message; the app may display a notification to alert them. This process is particularly helpful for responding to intents that do not require user interaction. To make effective use of Broadcast Receivers, developers should manifest them in the app’s manifest file, which registers the receiver to specific events.
Content Providers: Sharing Data
Content Providers are the backbone of data management in Android applications. They allow different applications to access and share data securely. For example, a social media app might leverage a Content Provider to access the phone's contact list. This isn’t just about accessing data; it’s about structuring how data is shared across different applications while maintaining security and privacy.
They often work with a standard URI scheme, allowing other applications to gain access to the content provider’s data. For example, if an app needs to retrieve a list of images or contacts, it will use a Content Provider rather than directly accessing the data. It's not just cleaner but also minimizes the risks related to data interoperability and security issues.
In summary, the core components of Android applications—Activities, Services, Broadcast Receivers, and Content Providers—are essential for building robust and efficient apps. They work together to ensure that applications function smoothly and provide a seamless user experience.
UI Design Principles in Android
User Interface (UI) design is the linchpin of any successful Android application. A well-designed UI not only enhances user engagement but also significantly bolsters the app's overall functionality. It serves as the bridge between the user and the application, which means a deep understanding of UI design principles is crucial for creating intuitive and visually appealing interfaces. This section will delve into its key aspects, addressing why it’s essential for both user satisfaction and the app's operational efficiency.
A coherent UI design fosters clarity and user flow, reducing frustration and downtime during interactions. Moreover, as the mobile market becomes saturated, distinguishing your app relies heavily on its interface. Users naturally gravitate towards applications that are not just functional but also pleasant to the eye. Therefore, mastering UI design principles can provide an undeniable edge in a competitive landscape.
Understanding Layouts
The layout is the backbone of an Android application's interface. It dictates how elements are arranged on the screen, significantly impacting usability and aesthetics. A solid layout can make navigation intuitive, enabling users to find what they need swiftly without falling into the trap of excessive scrolling or confusion.
Android offers various layout types, such as LinearLayout, RelativeLayout, ConstraintLayout, and FrameLayout, each serving different purposes. Here’s a brief overview:
- LinearLayout: Displays child elements in a single row or column, akin to stacking blocks. It’s simple but can create clutter if overused.
- RelativeLayout: Positions elements based on relationships with sibling elements or the parent layout, allowing for a more flexible arrangement.
- ConstraintLayout: Provides a more advanced framework for creating complex layouts while managing performance effectively.
- FrameLayout: Primarily designed to display a single item, making it useful for overlaying views.
Effective layout design considerations include responsiveness to different screen sizes and orientations. Utilizing tools like the Layout Inspector in Android Studio can help developers test and optimize layouts for various devices. This way, regardless of whether users are on a smartphone or tablet, the experience remains consistent and enjoyable.
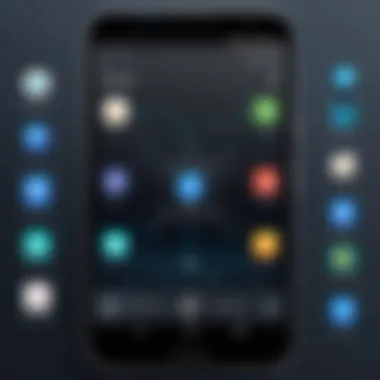
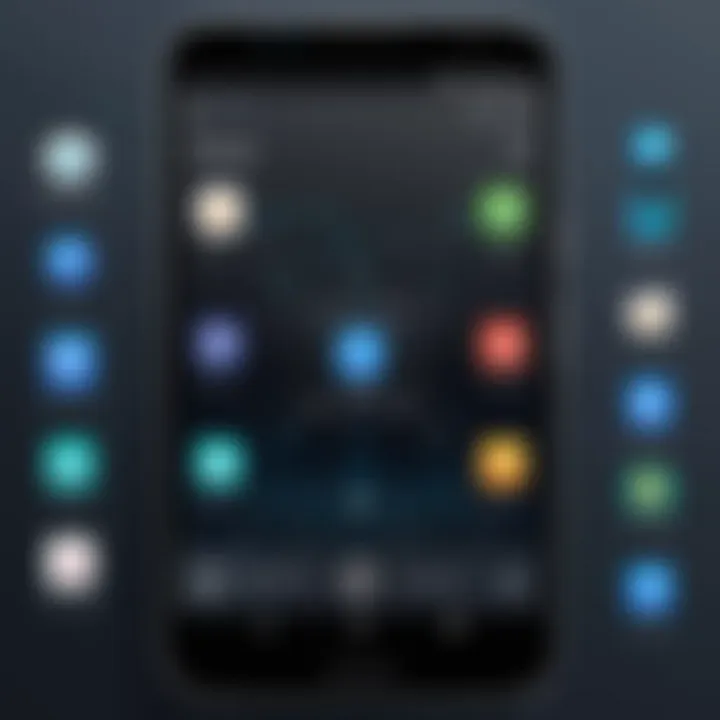
Using Material Design Guidelines
Material Design emerges from the need for a unified experience across various platforms and devices. This set of design principles encourages the creation of fresh, intuitive interfaces that resonate with users. Established by Google, Material Design outlines best practices in terms of color, typography, and space, promoting a cohesive look.
Implementing Material Design involves:
- Robust Color Palette: Choose a primary color with complementary secondary colors. This establishes brand identity while ensuring readability.
- Typography: Utilize easy-to-read fonts with a hierarchy that guides the user’s focus. Larger, bold fonts can denote titles or important information, while smaller fonts can be reserved for less critical text.
- Grid-Based Layouts: Consistency is key. Adhering to grid systems simplifies the placement of elements while fostering familiarity among users.
- Motion and Animation: Thoughtful animations can guide user attention and enhance interactivity without overwhelming.
Incorporating Material Design guidelines benefits developers by providing a blueprint for simplicity and clarity, which are paramount in today’s fast-paced app environment. Users appreciate familiar interfaces that make tasks straightforward.
"Good design is not just about aesthetics; it’s about solving problems and enhancing the user experience."
Ultimately, the marriage of thoughtful layout considerations and the Material Design framework can elevate an Android app from functional to exceptional. A user-friendly design not only leads to happy customers but also fosters loyalty and retention, giving developers a solid foundation to build upon.
Data Storage Options
In the realm of Android app development, understanding data storage options is akin to having a solid foundation on which to build a house. The way an application stores information influences performance, user experience, and even security. With an increasing amount of data generated by mobile applications, knowing when to leverage each storage solution can be significant for developers.
When you dive into data storage, you’ll find that it’s not just a technical necessity; it’s about enhancing user engagement through effective data management. Android provides various methods to store data, each catering to different scenarios and requirements, from small bits of user preferences to complex datasets needing structured queries. The choice of data storage can impact the app’s speed, security, and the ease with which updates and modifications can be made.
Shared Preferences: Simple Key-Value Storage
Shared Preferences is a straightforward mechanism that enables developers to store basic data in key-value pairs. Think of it as a place to stash small items—configurations, settings, or user preferences—where quick and easy access is paramount. This form of storage is particularly valuable for app settings, such as user themes or preferences, where the data remains lightweight and does not require complex querying.
For instance, if you have an app that allows users to select their language of choice, you would utilize Shared Preferences to save that selection. The next time the user opens the app, the preference is retrieved, providing a seamless experience.
Here's a sample code snippet for saving a preference:
SQLite Databases for Structured Data
On the flip side, when dealing with structured data that requires an intricate and organized system, SQLite databases come into play. SQLite is a powerful and lightweight relational database management system that allows you to manage complex data structures efficiently. Unlike Shared Preferences, which is suitable for small data, SQLite shines with larger datasets and intricate queries.
For example, if you were to create a notes app, SQLite would allow you to save multiple entries, perform CRUD (Create, Read, Update, Delete) operations, and handle complex queries efficiently. This capability is especially pertinent when handling relationships between data, such as linking notes to different categories or tags.
Using SQLite may not be as simple as Shared Preferences, but it significantly increases your ability to manage data effectively.
Using Room for Database Management
To bridge the gap between simplicity and the capabilities of SQLite, Room offers an abstraction layer over SQLite, making it easier to work with databases. With Room, you can define your data entities in a more straightforward manner, eliminating much of the boilerplate code associated with traditional SQLite handling. It enforces SQLite best practices and offers compile-time checks of SQL queries.
Room is particularly beneficial when working with LiveData and ViewModel architectures, enhancing the responsiveness of your UI by allowing it to observe database changes based on user interactions.
Consider this example of a simple entity in Room:
Combining these approaches can cater to various use cases—if an app requires a lightweight solution for user preferences, Shared Preferences might be best. Conversely, larger data sets would benefit from SQLite, and for those who find the need for a more comprehensive management system, Room stands as a worthy choice. With the right data storage options, developers not only enhance app performance but also optimize the overall user experience.
Networking and APIs
Networking and APIs are at the heart of modern mobile application development. With the explosion of data-driven apps being used daily, understanding how to communicate with remote servers, databases, or other services is key. The essence of a dynamic Android application lies in its ability to retrieve and send data in real-time, and this is made possible through effective network communication and API integration.
APIs (Application Programming Interfaces) allow your app to interact with external services and databases. Imagine a weather app that gathers real-time data from a weather service; the API is what enables this seamless interaction. When you grasp networking concepts and how to implement APIs in your apps, you empower them to function more like living entities, continuously pulling in new information based on current demands.
Here are some of the specific elements that highlight the necessity of mastering networking and APIs:
- Data Retrieval: Essential for apps that rely on real-time information, such as social media, news, and fintech apps. Without APIs, these apps would offer static content.
- User Interaction: Many apps require user inputs to send data back to servers. Understanding how to facilitate communication directly affects the user experience.
- Third-party Integration: APIs allow integrating third-party services like cloud storage, payment processing, or geolocation, enhancing your app's functionality.
When working with networks and APIs, there are a few considerations to keep in mind:
- Mobil data usage can impact performance and user satisfaction.
- Error handling for network requests is crucial to maintain app stability.
- Knowledge of asynchronous programming is required to ensure smooth user experience while waiting for network responses.
"APIs represent a bridge between the code you write and the external services or data your app needs."
Mastering networking in Android thus enables you to develop robust applications that meet the challenges of modern computing, ensuring they remain relevant and useful.
Making API Calls with Retrofit
Retrofit, a powerful networking library developed by Square, has gained traction among Android developers for a reason. It's straightforward, efficient, and flexible, making the process of working with REST APIs much simpler.
What makes Retrofit appealing?
- Ease of Use: You can define API endpoints, parameters, and headers seamlessly using annotations.
- Support for JSON: It includes built-in support for converting JSON to Java objects, reducing the need for boilerplate code.
- Asynchronous calls: Retrofit excels in managing background tasks without blocking the main thread.
Here’s a basic example of how you might set up a Retrofit call:
Using Volley for Network Operations
If you're looking for a library that can manage network operations and cache responses more intelligently, Volley might be the right fit. Developed by Google, it is optimized for handling asynchronous networking tasks and handling several requests simultaneously without running into performance bottlenecks.
Key advantages of using Volley include:
- Automatic Request Queuing: Volley manages a queue of requests, ensuring that while one is processing, others can wait in line.
- Caching Mechanism: This feature minimizes network calls by caching responses, making subsequent requests for the same data much faster.
- Easier Error Handling: It simplifies handling connectivity issues or application errors with easy-to-implement functionalities.
Here's a simple way to set up a Vollet request:
Getting familiar with these libraries will significantly improve your capability to build robust applications that can tap into the world of data and services, thereby enriching user experience and adding layers of functionality.
Testing Android Applications
Testing Android applications is an essential part of the development cycle. It goes beyond merely finding bugs; it helps ensure that apps function consistently and meet user expectations. Strong testing practices can make or break an application. When developers prioritize testing, they not only save time and resources but enhance user satisfaction and trust in the product.
The significance of testing lies in its ability to catch issues before they reach the end-user. Imagine trying to navigate an app filled with glitches or crashes—frustrating, right? Effective testing prevents such scenarios by identifying problems at various stages of the development process.
Moreover, the benefits of thorough testing positively impact the application’s reputation. A well-tested app is less likely to receive negative feedback or low ratings on platforms like the Google Play Store. Instead, users tend to recommend those apps to their peers, thus boosting download rates. Ultimately, rigorous testing contributes to building a strong brand image.
Unit Testing Frameworks and Tools
Unit testing is a method where individual components of software are tested independently. In the context of Android development, unit tests verify that the smallest parts of an application—like methods and classes—perform accurately. By creating unit tests, developers can ensure that changes made during development won’t inadvertently cause previous features to fail.
Several frameworks are at a developer's disposal for unit testing in Android:
- JUnit: A widely-used framework that provides a simple, effective way to run tests on Java code. It's integrated seamlessly within Android Studio.
- Mockito: A mocking framework allowing advanced testing techniques by simulating objects that an application will interact with.
- Robolectric: This framework allows developers to run Android tests directly in the JVM, making the testing process quicker without needing an emulator.
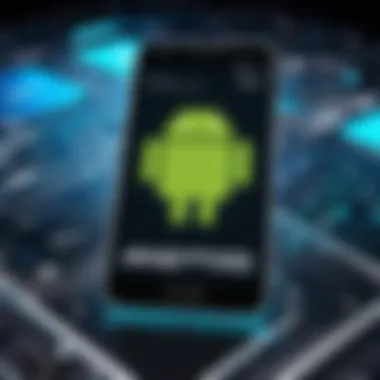
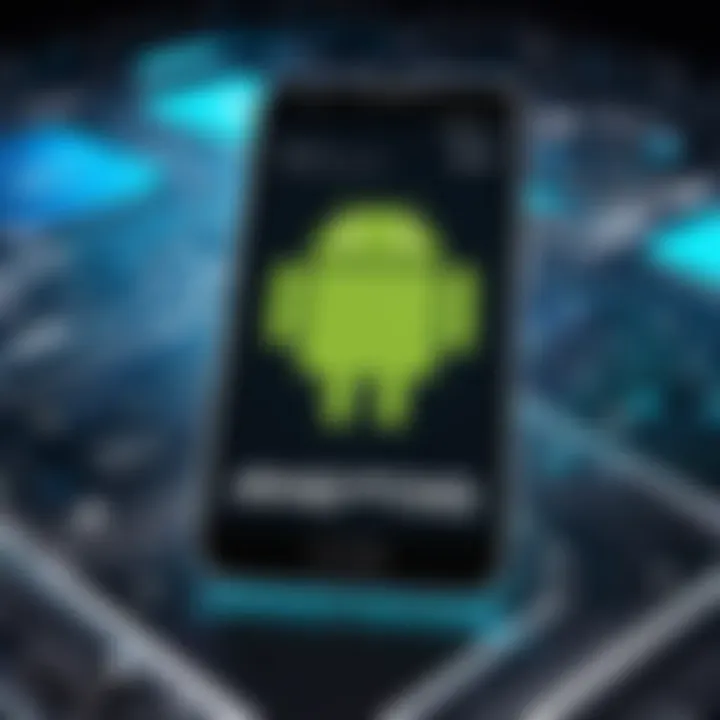
With these tools, developers can automate their testing workflows, reducing manual effort and increasing efficiency. Better organized tests improve maintainability, which is key in adapting apps to new features or updates.
UI Testing with Espresso
UI testing is another critical aspect, where the focus is on the graphical interface of an application. Espresso is one of the core frameworks used for UI testing in Android, designed to make it easy to write reliable user interface tests. With Espresso, developers can simulate user interactions on the app’s interface, ensuring that buttons work, screens load correctly, and data displays as expected.
Utilizing Espresso is straightforward due to its concise syntax. It allows developers to
- Write concise tests: Scene descriptors enable tests to look clean and clear.
- Synchronize actions with the app state: Espresso’s intelligent synchronization ensures that tests execute smoothly, only when the app is ready.
Implementing UI tests with Espresso involves simple setups. Basic examples might look like this:
Here, a button click is simulated, and it checks whether a text view updates accordingly. This simplistic yet powerful technique enables robust verification of user interaction.
Remember: The goal of testing is not to please the development team, but to enhance end-user experience.
Publishing an Android App
The path from coding to deployment is like sailing from familiar shores into unpredictable waters. Publishing an Android app is an essential step that transforms a developer’s hard work into a real-world resource for users. Getting to this stage means your app has met the necessary quality and functionality standards, but the voyage is far from over once you hit the "publish" button.
Understanding the nuances involved in publishing is critical. This phase is where the rubber meets the road, and a few key elements must be kept in mind:
- User Accessibility: Making your app available on the Google Play Store ensures that a vast audience can access it. With billions of Android devices in the world, the potential for user engagement is enormous.
- Visibility and Marketing Benefits: Even the best application won’t gain traction without proper exposure. The Google Play Store provides a platform with built-in marketing strategies to help your app reach its target users.
- Updates and Maintenance: Publishing isn’t just a one-off event. It sets the stage for future updates, allowing you to improve your app based on user feedback and emerging technology trends.
In this section, we deconstruct the essential steps associated with preparing for release and publishing on the Google Play Store.
Preparing for Release
Preparation is the backbone of a seamless launch. If the groundwork is not laid meticulously, all that effort could crumble like a house of cards, leaving both developers and users unsatisfied. Here are the crucial steps you'll want to follow while preparing for your app's release:
- Optimizing Your App: Before releasing, ensure that your app is optimized for performance. This includes minimizing battery usage and memory consumption, which can lead to a smoother user experience.
- Versioning Your App: Proper versioning matters. When you upload your app, clearly define your app's version using numbers that reflect changes. This helps users and you track updates easily.
- Testing Across Multiple Devices: Not all Android devices are created equal. Tests should be run on different screen sizes and operating systems to catch potential issues early.
- Creating a Release Build: Ensure you’re working with the correct configuration for the release version, including signing your app with a release key, which is critical for app security.
- Preparing Legal Material: Compliance with the legal aspects must not be overlooked. This includes crafting privacy policies and user agreements that comply with local legislation.
As you navigate these considerations, be mindful that preparation isn’t just about the technical side. The user experience also depends on effective marketing and support strategies.
Publishing on Google Play Store
Now that the groundwork is set, it’s time to embark on the final phase — publishing your app to the Google Play Store. This process may appear straightforward, yet there are intricacies to grasp.
"Publishing isn’t merely about upload and hope; it’s about positioning your app for long-term success."
Here’s an outline of the steps you need to take:
- Create a Developer Account: The first step is to register for a Google Play Developer account, which requires a one-time registration fee. This account gives you the credentials needed to publish.
- Preparing the Store Listing: When creating your app’s store listing, you must provide documentation like descriptions, screenshots, and a promotional video, if possible. These elements significantly enhance your app’s attractiveness.
- Setting Distribution Settings: Decisions on device compatibility, countries or regions, and pricing settings for your app must be made during this phase. Planning your distribution wisely can boost your app’s reach.
- Submission and Review: After submitting your app, it goes through a review process by Google, which checks for compliance with their guidelines. Be prepared for possible feedback or required changes.
- Post-Publication Feedback and Updates: After successful publishing, keep a keen eye on user reviews and ratings. Addressing concerns and integrating their feedback in future updates is essential for maintaining your app’s success.
The journey from a mere idea to a fully-functioning application on the Google Play Store involves careful planning and execution. Each step not only enhances the quality and usability of your app but also builds a solid foundation for its future in a constantly evolving marketplace.
Security Measures in Android Development
In the world of mobile applications, security has become a paramount concern. As these apps hold a treasure trove of personal information and sensitive data, a firm grasp on security measures is key to protecting not just the users, but also the developers and the integrity of their creations. Security in Android development isn’t merely a checkbox—it's a continuous process that weaves through every stage of development. In this section, we will explore the critical elements of Android security, including permissions and sensitive data handling, while detailing their significance.
Security is indispensable not only for compliance with regulations but also to maintain trust with users. A well-secured app encourages more downloads and fosters a positive image for the developer. With the rise of cyber threats, staying ahead in terms of security has become an essential part of the development lifecycle.
A developer's responsibility goes beyond coding; it involves safeguarding the user experience as well.
Understanding Android Permissions
Permissions in Android are gates that regulate access to sensitive device features and user data. When an app requests permissions, it’s essentially asking for a ticket to interact with functionalities like the camera, location services, or contacts. Each permission type falls into either a normal or dangerous category.
- Normal permissions are granted automatically, as they don't pose significant risks. An example includes the permission to set an alarm.
- Dangerous permissions, on the other hand, require user consent, as they can affect a user's privacy or the device's security. Examples are permissions to access the microphone or read contacts.
Understanding permissions is vital not just for compliance but also for enhancing user experience. When an application requests permissions responsibly, it can mitigate user frustration and avoid potential uninstallations stemming from concerns over privacy invasion. Develeopers should always provide clear explanations about why certain permissions are necessary, which can be achieved through user-friendly dialogues.
However, there’s more than just formalities to consider. Implementing the principle of least privilege is crucial—only request permissions that are absolutely necessary for the app's functionality. This ensures that the application does not become a blanket that absorbs every user’s data unnecessarily.
Securing Sensitive Data
Once permissions are granted, the focus shifts to safeguarding sensitive data. This situation brings to mind the old adage—“A chain is only as strong as its weakest link.” In the context of app development, even a minor oversight can lead to data leaks or catastrophic breaches. Here are some strategies to secure sensitive information:
- Encryption: Encrypting data both at rest and in transit is crucial. For instance, sensitive user inputs like passwords should never be stored in plain text. Tools like AES (Advanced Encryption Standard) can be employed to enforce strong encryption standards.
- Proper Data Handling: Developers should avoid hardcoding sensitive information, such as API keys or passwords, directly into the application code. Instead, utilize alternatives like secure storage systems or environment variables.
- Use Secure Communications: Ensure that all data-fetching operations within your app communicate over HTTPS. This not only helps to safeguard data from eavesdroppers but also builds trust among users.
- Regular Security Audits: Periodic assessments of the app and code help in identifying potential vulnerabilities that might arise due to new operating system updates or frameworks.
By weaving these security measures into the fabric of Android applications, developers not only protect their users but also build robust applications that stand resilient against cyber threats. Security is not an afterthought—it's built through foresight and diligence. In a world where digital threats are ever-evolving, it's smart to stay proactive in securing your applications.
Future Trends in Android Development
Android development continuously evolves, adapting to technological advancements and user needs. Understanding these changes is essential for both budding developers and seasoned pros, as it shapes the strategies for creating engaging applications. This segment explores the emerging trends that are likely to influence the Android landscape and why keeping abreast of them is crucial.
Emerging Technologies in Mobile Development
The mobile development sphere is undergoing a seismic shift thanks to new technologies that are both innovative and practical. Here are some critical elements transforming the landscape:
- 5G Technology: The advent of 5G networks is a game-changer. It allows for faster data downloads, lower latency, and improved user experiences. Apps can now handle rich media content such as augmented reality and high-definition video streaming smoothly.
- Augmented Reality (AR) and Virtual Reality (VR): Devices are increasingly integrating AR and VR capabilities. Individuals can build experiences that blend the digital and physical worlds, enhancing storytelling, gaming, and educational applications. Imagine apps that allow users to preview furniture in their living rooms before purchasing!
- Internet of Things (IoT): This technology facilitates smart device connectivity. Android applications can now interact with diverse sensors, home appliances, and wearables, fostering a seamless user experience and a centralized ecosystem in smart homes and cities.
- Blockchain: Framed mostly around security and transparency, blockchain technology can provide enhanced security features for apps handling sensitive user information or transactions, adding a layer of trust for users.
Integrating these elements not only enhances the functionality of applications but also enriches the user experience, making them more intuitive and responsive to everyday needs.
The Role of Artificial Intelligence
Artificial Intelligence is no longer just a buzzword; it's becoming a cornerstone of modern Android development. The integration of AI technologies into apps is not merely a trend but a necessity for developers aiming to provide exceptional user experiences.
- Personalized Experiences: AI can analyze user behavior, allowing developers to create tailored experiences. For example, recommendation systems in mobile shopping apps, like those in Amazon, use AI to suggest products based on past searches and purchases.
- Voice Recognition: With tools like Google Assistant and voice commands gaining popularity, apps are increasingly adopting voice recognition technologies. This makes navigating interfaces more user-friendly, especially for those who may find traditional input methods inconvenient or challenging.
- Chatbots and Automated Customer Support: Embedding AI-driven chatbots improves customer service by providing instant responses to user queries, thus offering support around the clock. This is evident in many apps today, which handle various customer concerns without human intervention, freeing staff to tackle more complex issues.
- Enhanced Security: AI can also bolster security measures in apps. By detecting unusual patterns and behaviors, AI algorithms can provide real-time alerts, contributing to a tighter security net against potential threats.
In summary, as Android developers, staying informed on these trends and technologies isn't just good practice—it's essential for crafting the next generation of mobile applications that resonate with users and stand the test of time.
Community and Resources
In the vast landscape of Android development, community engagement plays a pivotal role. It not only fosters collaboration but also helps in sharing knowledge and resources, making it easier for developers—both budding and seasoned—to navigate challenges and broaden their horizons. As Android remains a dynamic platform, keeping pace with the latest trends and technologies can be daunting. Here, community and resources become invaluable, offering support, guidance, and a continuous flow of updates, tools, and best practices.
Contributing to Open-Source Projects
One of the most rewarding ways to engage with the Android development community is through open-source projects. Contributing to these projects serves as a learning experience, granting insight into industry standards and practices while improving one's coding skills. Developers can pick and choose projects that resonate with their interests or align with skill growth, be it Kotlin libraries or Java frameworks.
Participating in open-source fosters a collaborative spirit. Developers exchange ideas, troubleshoot issues together, and even enhance existing codebases. As a result, they gain real-world experience in version control systems like Git and learn how to work effectively in teams—a crucial skill in the tech industry.
In addition, contributing to these initiatives allows developers to showcase their work. A portfolio filled with contributions to significant projects often speaks louder than a resume. This visibility not only puts developers on the radar of potential employers but also builds credibility within the community.
Accounts like GitHub host countless repositories ready for contributions. Immerse yourself in the conversations around these projects in forums or on sites such as reddit.com to find opportunities that match your skills and ambitions.
Key Online Resources and Forums
The digital world is replete with resources for Android development. Tapping into these resources can significantly accelerate your learning curve and enhance your development capabilities. Here's a breakdown of some vital platforms and forums that every Android developer should explore:
- Stack Overflow: A go-to for troubleshooting, it has a plethora of technical questions and answers on Android-related queries. The community is quite active, making it a great place to get swift responses.
- Android Developers Website: This is the official hub for Android development. It offers documentation, guidelines, and resources that are essential for any budding developer.
- GitHub: Apart from hosting projects, it has an active community where developers can find collaboration opportunities, share knowledge, and contribute to open-source.
- Reddit: Subreddits like r/androiddev allow developers to connect, share experiences, and discuss the latest trends or challenges in Android development.
- Facebook Groups: There are numerous Android development groups that serve as forums for discussion, networking, and event sharing. Engaging in these groups opens doors for interaction with industry professionals and prospective collaborators.
Utilizing these resources can enhance your development journey, providing not just technical guidance but also emotional support through shared experiences. As you embrace this collective wealth of knowledge, you position yourself on a steady path toward becoming a proficient Android developer.