Unraveling the Expanse of Structured Programming Languages: A Comprehensive Exploration

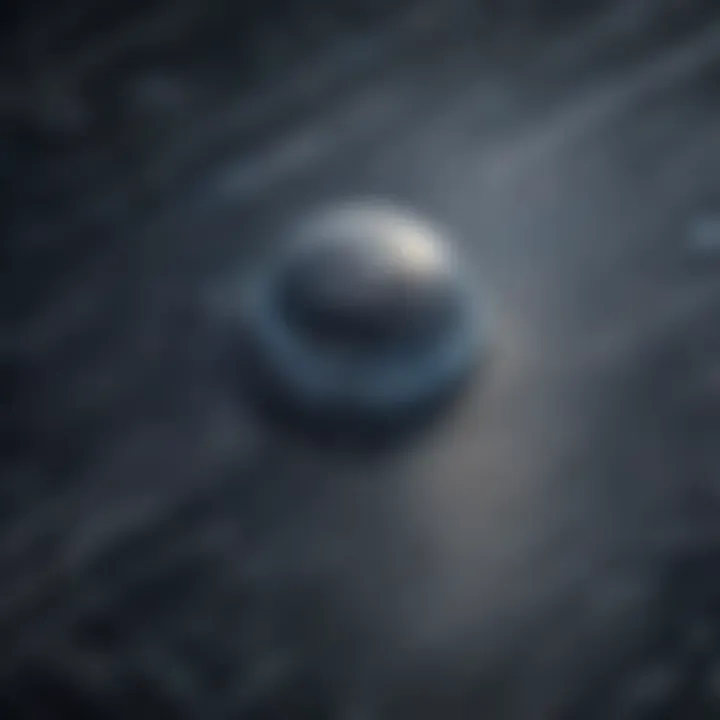
Synthesizing the Information Presented Throughout
By acquainting oneself with the foundational concepts of storage, security, and networking in the context of structured programming languages, developers gain a holistic understanding of the interconnected elements that shape modern software development. Incorporating best practices, industry trends, case studies, and reviews facilitates the integration of structured programming languages with optimal storage, robust security measures, and high-performance networking strategies. Synthesizing the information presented throughout the article equips IT professionals, cybersecurity experts, and students with a comprehensive guide to navigating the dynamic landscape of structured programming languages, empowering them to create secure, efficient, and innovative software solutions.
Introduction to Structured Programming
Structured programming is a foundational concept in software development that emphasizes organizing code into logical and reusable structures. In this article, we delve deep into the realm of structured programming languages, exploring their significance, characteristics, and role in modern software development. This section serves as a crucial primer for readers, highlighting the fundamental principles that underpin structured programming and setting the stage for a comprehensive exploration of this topic. By understanding the core tenets of structured programming, readers will gain insights into how these languages streamline the development process, improve code readability, and enhance overall program efficiency.
Evolution of Programming Paradigms
Procedural Programming
Procedural programming is a fundamental programming paradigm that follows a linear top-down approach to problem-solving. In the context of structured programming, procedural languages play a vital role in breaking down complex tasks into manageable procedures. The key characteristic of procedural programming lies in its systematic execution of instructions in a predetermined sequence, promoting code reusability and modularity. While procedural programming offers simplicity and ease of understanding, drawbacks such as lack of data encapsulation may limit its scalability in larger applications.
Object-Oriented Programming
Object-Oriented Programming (OOP) revolutionized the way developers conceptualize and design software systems. By focusing on objects and their interactions, OOP enhances code organization, fosters code reuse through inheritance and polymorphism, and promotes the encapsulation of data within objects. The key characteristic of OOP lies in its modeling of real-world entities as objects, leading to more intuitive and efficient code structuring. Despite its advantages in modeling complex systems, OOP may introduce overhead in terms of performance and memory usage in certain scenarios.
Functional Programming
Functional programming approaches software development from a mathematical perspective, treating computation as the evaluation of mathematical functions. Functional languages emphasize immutability, higher-order functions, and declarative programming style. The key characteristic of functional programming is its focus on pure functions that avoid side effects, enabling better code modularity and testability. While functional programming excels in handling complex data transformations, its steep learning curve and limited support for mutable state can pose challenges for developers working in imperative programming environments.
Fundamentals of Structured Programming
Sequential Control Structures
Sequential control structures dictate the flow of a program from one statement to the next, following a linear execution path. These structures define the order in which instructions are executed, allowing programmers to implement algorithms step-by-step. The key characteristic of sequential control structures is their deterministic nature, ensuring predictability in program flow. While sequential control structures promote code clarity and logic simplification, they may become restrictive when dealing with non-linear program flows or asynchronous operations.
Selection Control Structures
Selection control structures enable programmers to make decisions within their code based on certain conditions. By incorporating conditional statements such as if-else and switch-case, developers can control the execution path of a program dynamically. The key characteristic of selection control structures is their ability to introduce branching logic, facilitating adaptive program behavior based on runtime conditions. While selection control structures enhance code flexibility and decision-making capabilities, excessive nesting of conditional statements can lead to code complexity and reduced maintainability.
Iteration Control Structures
Iteration control structures, also known as loops, enable repetitive execution of code segments until certain conditions are met. Through constructs like for, while, and do-while loops, developers can streamline the processing of collections, implement iterative algorithms, and automate recurring tasks. The key characteristic of iteration control structures is their ability to iterate over data sets or perform iterative computations efficiently. While iteration control structures enhance code reuse and optimize algorithm efficiency, poorly designed loops can result in performance bottlenecks and potential infinite loops.
Importance of Program Structure
Enhanced Readability
Enhanced readability is a core aspect of structured programming that focuses on making code more understandable and accessible to developers. By adopting clear naming conventions, organizing code into logical blocks, and incorporating meaningful comments, programmers can enhance the readability of their programs. The key characteristic of enhanced readability is its impact on reducing cognitive load, promoting code comprehension, and easing collaborative development efforts. While enhanced readability improves code maintainability and debugging efficiency, excessive comments or overly verbose code may hinder readability and introduce information redundancy.
Improved Maintainability
Improved maintainability encompasses the ease with which developers can update, modify, and extend existing code without introducing errors or disruptions. Through modular design, adherence to coding standards, and documentation practices, programmers can enhance the maintainability of their software projects. The key characteristic of improved maintainability is its contribution to long-term code sustainability, lowering the risk of software decay and technical debt accumulation. While improved maintainability simplifies code maintenance and fosters agile development practices, neglecting proper documentation or overlooking design optimizations can hinder the scalability and evolution of software projects.
Facilitated Debugging
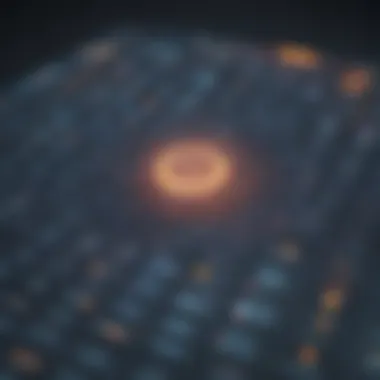
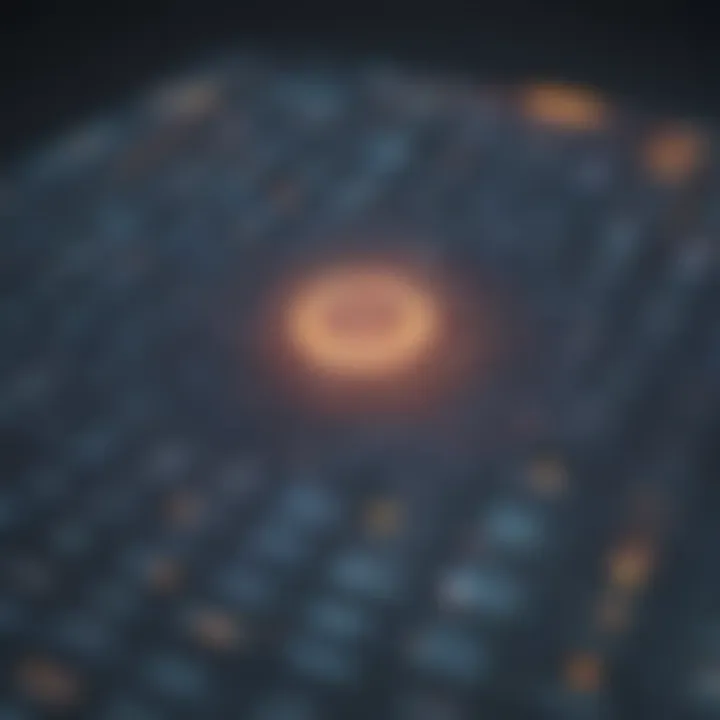
Facilitated debugging refers to the process of identifying and resolving software defects efficiently and effectively. By incorporating error handling mechanisms, logging utilities, and debugging tools, developers can streamline the debugging process and diagnose issues in their code. The key characteristic of facilitated debugging is its role in enhancing code reliability, accelerating bug resolution, and improving software quality. While facilitated debugging reduces time-to-resolution for code issues and promotes a proactive approach to software quality assurance, over-reliance on debugging tools or neglecting root cause analysis can mask underlying issues and lead to persistent bugs.
Key Concepts in Structured Programming
Structured programming is a fundamental framework in software development that emphasizes organization, readability, and maintainability. This section delves into the core principles that underpin structured programming languages, highlighting their importance in modern coding practices. By ingraining key concepts like modularity, reusability, and top-down design, developers can streamline the development process and enhance the quality of their code.
Modularity and Reusability
Modularity is a cornerstone principle in structured programming, advocating for the decomposition of code into discrete, interchangeable modules. These modules, often in the form of functions or subroutines, facilitate code reuse and maintenance, promoting efficiency and clarity in program structure. By compartmentalizing code logic, developers can isolate specific functionalities, fostering reusability across projects and minimizing redundancy.
FunctionsSubroutines
Functions and subroutines encapsulate specific sets of operations within a program, promoting code reuse and enhancing readability. The hallmark of functionssubroutines lies in their ability to break down complex tasks into manageable units, fostering a modular design approach. Through the encapsulation of logic, functionssubroutines promote reusability, reduce code duplication, and enhance the overall maintainability of software systems.
Abstraction
Abstraction involves hiding complex implementation details behind a simplified interface, allowing developers to focus on the essential aspects of a system. By abstracting intricate logic into easily understandable constructs, programmers can enhance code readability and maintainability, facilitating system comprehension and modification. This principle enables developers to tackle complex problems by emphasizing essential concepts and concealing superfluous complexities.
Encapsulation
Encapsulation involves bundling data and associated functionalities into coherent units, promoting data security and code reliability. By encapsulating data within defined structures, such as classes or modules, developers shield internal details from external access, reducing code coupling and enhancing system robustness. Encapsulation fosters a clear separation of concerns, promoting code integrity and mitigating the risk of unintended data manipulation.
Data Structures and Algorithms
Data structures and algorithms form the bedrock of structured programming, enabling efficient data organization and manipulation. This section explores essential data structures such as arrays, linked lists, and sorting techniques, elucidating their significance in software development. By leveraging these foundational concepts, developers can optimize resource utilization, improve algorithmic efficiency, and implement scalable solutions.
Arrays
Arrays represent contiguous collections of similar elements, providing a mechanism for systematic data storage and retrieval. The key characteristic of arrays lies in their indexed access, allowing for efficient element manipulation and traversal. While arrays offer fast element access and predictable performance, they are constrained by fixed sizes and lack dynamic resizing capabilities.
Linked Lists
Linked lists consist of nodes that are dynamically linked together, forming flexible data structures with variable sizes. The distinctive feature of linked lists is their dynamic memory allocation, enabling efficient insertions and deletions at arbitrary positions. Although linked lists offer flexibility and dynamic memory management, they incur additional overhead in terms of memory consumption and traversal complexity.
Sorting Techniques
Sorting techniques encompass algorithms that arrange elements in a specified order, optimizing data retrieval and search operations. Popular sorting algorithms include bubble sort, merge sort, and quicksort, each offering unique trade-offs in terms of time complexity and space efficiency. By implementing efficient sorting algorithms, developers can enhance the performance of data-intensive applications and streamline information processing.
Top-down Design Approach
The top-down design approach advocates for problem decomposition from general goals to specific subtasks, fostering a systematic problem-solving methodology. By breaking down complex problems into manageable components, developers can hierarchically structure software systems, promoting clarity and maintainability. This methodology emphasizes stepwise refinement, iterative development, and hierarchical organization to ensure optimal system design.
Breaking Down Problems
Breaking down problems involves dissecting overarching objectives into smaller, achievable tasks, facilitating focused problem-solving strategies. The key characteristic of problem decomposition lies in its ability to simplify complex issues into manageable segments, fostering systematic problem analysis and solution identification. By deconstructing large-scale problems into digestible units, developers can incrementally address challenges and iteratively refine solutions.
Hierarchical Structure
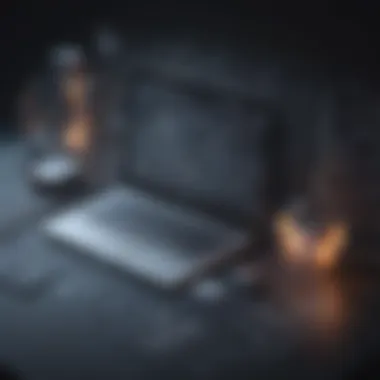
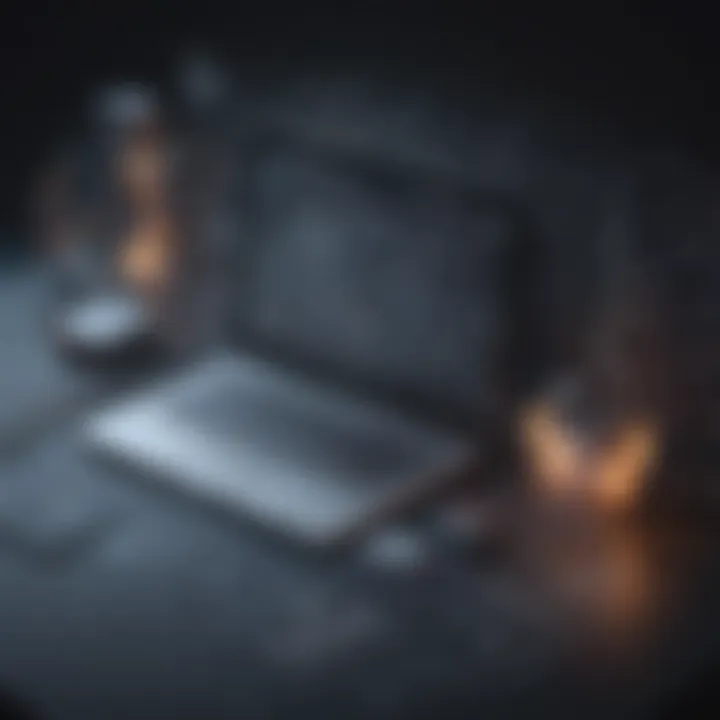
Hierarchical structure entails organizing system components into layered levels of abstraction, promoting modular design and scalability. The primary feature of hierarchical structure is its nested arrangement, where higher-level modules encapsulate lower-level functionalities. This hierarchical organization facilitates system comprehension, promotes code reusability, and offers a structured approach to software development.
Stepwise Refinement
Stepwise refinement involves iteratively elaborating on high-level abstractions, gradually refining system details to achieve implementation precision. The essence of stepwise refinement is its incremental nature, where developers progressively add detail and functionality to system components. This iterative refinement process ensures comprehensive system design, methodical implementation, and iterative testing to validate system functionality and performance.
Advantages of Structured Programming Languages
Structured programming languages offer a myriad of benefits that significantly enhance the efficiency and effectiveness of software development. One key aspect is the emphasis on code maintainability, which plays a pivotal role in reducing errors, easing debugging processes, and enhancing program scalability. Code maintainability ensures that programs are easier to manage, modify, and update over time. It promotes the creation of well-structured and readable code, leading to better software quality and durability.
Code Maintainability
Easy Debugging
Easy debugging is a crucial component of code maintainability in structured programming. It involves the ability to identify and rectify errors in the code swiftly and accurately. By facilitating the detection of bugs and problems during the development process, easy debugging improves the overall robustness and reliability of the software. This aspect is essential in ensuring that the program functions correctly and meets the specified requirements. Although debugging can be time-consuming, the ease of locating issues promptly enhances the development cycle's efficiency.
Enhanced Reusability
Enhanced reusability is another significant advantage of code maintainability. It allows developers to reuse existing code components efficiently across multiple projects or within the same program. By creating reusable modules and functions, developers can streamline the development process, save time, and enhance productivity. This practice not only reduces redundancy in code but also improves consistency and standardization, leading to more maintainable and scalable software solutions.
Scalability
Scalability in code maintainability relates to the ability of a program to handle increased workloads and growing data volumes without compromising performance. A well-maintained codebase provides the flexibility to scale the software system effortlessly to accommodate changing requirements or user demands. Scalability is crucial for addressing future expansion needs, ensuring that the program can grow seamlessly without significant code refactoring or performance bottlenecks. By incorporating scalable design principles, developers can future-proof their applications and adapt to evolving technology landscapes effectively.
Challenges and Limitations in Structured Programming
Structured programming languages bring tremendous benefits to the software development landscape; however, they are not devoid of challenges and limitations. Understanding these obstacles is crucial for developers and programmers aiming to leverage the full potential of structured programming. By delving into the challenges and limitations in structured programming, individuals can enhance their problem-solving skills and optimize their coding practices.
Complexity Management
Overhead in Designing
In the realm of structured programming, Overhead in Designing plays a pivotal role in shaping the efficiency and effectiveness of code implementation. This aspect focuses on the additional complexities and demands placed on developers during the design phase. The key characteristic of Overhead in Designing lies in the extensive planning and analysis required before the actual coding process begins. While it can seem burdensome, this meticulous approach ensures that the final product meets the desired specifications and functionalities, enhancing the overall quality of the program. However, excessive overhead in designing may lead to extended development timelines and increased resource allocation, posing potential drawbacks to project completion.
Nested Structures
Nested Structures in structured programming involve the incorporation of multiple levels of abstraction within the code. This arrangement allows for intricate hierarchies and relationships among different elements within the program. The key characteristic of Nested Structures is their ability to encapsulate and organize complex data structures, enhancing code readability and maintenance. By nesting structures, developers can partition large datasets into manageable units, streamlining data access and manipulation. Despite these advantages, over-reliance on nested structures may lead to overly convoluted code and hinder code comprehension, making debugging and troubleshooting more challenging.
Maintaining Consistency
Maintaining Consistency is a fundamental aspect of structured programming that focuses on preserving uniformity and coherence throughout the codebase. The key characteristic of Maintaining Consistency lies in upholding standardized coding practices, naming conventions, and design principles across a project. By ensuring consistency in coding styles and implementations, developers can facilitate collaboration, minimize errors, and promote code reusability. However, strict adherence to consistency may constrain creative problem-solving approaches and innovation, potentially inhibiting the exploration of alternative solutions.
Performance Trade-offs
Execution Speed
Execution Speed in structured programming determines the rate at which code instructions are processed and executed by the system. This aspect directly impacts the overall performance and responsiveness of the software application. The key characteristic of Execution Speed is its role in optimizing code efficiency and reducing latency. By streamlining algorithms and minimizing computational overhead, developers can enhance the speed of program execution, ensuring swift response times for end-users. However, prioritizing execution speed over other considerations may lead to code optimization trade-offs, compromising code readability and maintainability.
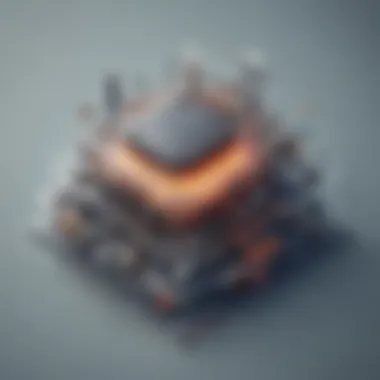
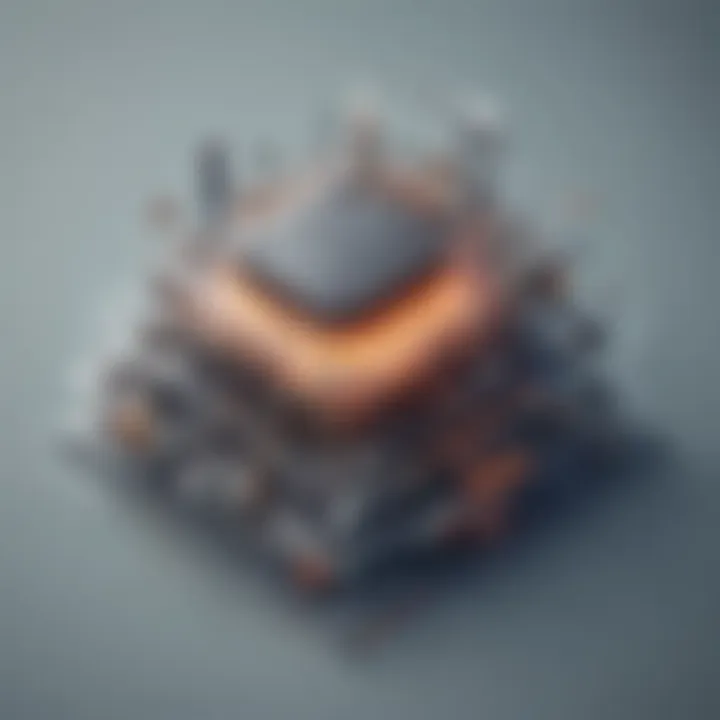
Resource Consumption
Resource Consumption in structured programming refers to the utilization of system resources such as CPU, memory, and storage during program execution. The key characteristic of Resource Consumption is its impact on system performance and scalability. By managing resource allocation effectively, developers can optimize program efficiency, prevent resource bottlenecks, and improve overall system stability. However, inefficient resource utilization may result in memory leaks, performance degradation, and system crashes, underscoring the importance of optimizing resource consumption in structured programming.
Memory Utilization
Memory Utilization is a critical factor in structured programming that influences the efficient management and allocation of memory resources within a program. The key characteristic of Memory Utilization is its effect on program stability, data access speeds, and overall system responsiveness. By optimizing memory utilization, developers can mitigate memory leaks, reduce memory fragmentation, and enhance program performance. However, improper memory management can lead to memory overflow, segmentation faults, and unpredictable program behavior, necessitating careful consideration and optimization strategies to maximize memory efficiency.
Future Trends in Structured Programming Languages
In the intricate realm of software development, staying abreast of Future Trends in Structured Programming Languages is paramount. As technology evolves, the landscape of programming paradigms undergoes dynamic shifts. Understanding these emerging trends is crucial for IT professionals, cybersecurity experts, and students alike. The fusion of structured programming with Artificial Intelligence, IoT Development, and Blockchain Implementation is reshaping the way software is designed and executed.
Integration with Emerging Technologies
Artificial Intelligence
Artificial Intelligence (AI) stands at the forefront of technological advancements, embedding intelligence into programs. Its capacity for learning and decision-making sets it apart from traditional computing methods. In the context of structured programming languages, AI brings automation, predictive analytics, and cognitive capabilities, optimizing processes. Despite its benefits, AI's complexity and resource demands pose challenges in integration, requiring streamlined algorithms and efficient data processing.
IoT Development
IoT Development revolves around interconnected devices sharing data seamlessly, fostering the concept of a digital ecosystem. This integration extends the capabilities of structured programming languages, enabling real-time communication and advanced functionalities. The scalability and diversity of IoT applications demand robust security measures and efficient data handling, presenting opportunities for structured programming to enhance reliability and performance.
Blockchain Implementation
Blockchain, synonymous with secure decentralized transactions, is revolutionizing data integrity and trust mechanisms. Its incorruptible ledger system promotes transparency and security, aligning with the principles of structured programming. By incorporating blockchain into software development, immutability, and cryptographic protections fortify system integrity. However, the resource-intensive nature of blockchain operations necessitates optimized algorithms for seamless integration within structured programming paradigms.
Enhancements in Compilation Techniques
Just-In-Time Compilation
Just-In-Time Compilation optimizes program execution by converting code into machine language during runtime. This real-time translation enhances performance and reduces memory overhead, ideal for resource-constrained environments. Structured programming languages benefit from JIT compilation's adaptive optimization strategies, offering faster deployment and efficient memory management. Despite its advantages, JIT compilation's initial overhead and debugging complexities require meticulous monitoring and refinement.
Optimization Strategies
Optimization Strategies streamline program efficiency and resource utilization through algorithmic enhancements. These strategies fine-tune code execution, minimizing bottlenecks, and improving response times. Incorporating optimization techniques in structured programming enhances code maintenance and scalability, crucial for large-scale software projects. Balancing performance gains with complexity demands a strategic approach to algorithm design and implementation.
Static Code Analysis
Static Code Analysis scrutinizes code for potential errors, security vulnerabilities, and compliance with coding standards. As a fundamental aspect of structured programming, static analysis fortifies code quality and identifies coding inconsistencies early in the development cycle. Automatic detection of bugs and optimization opportunities streamlines the debugging process, enhancing software reliability. Despite its efficacy, static code analysis demands well-designed coding practices and continuous adherence to coding guidelines.
Evolution of Programming Paradigms
Concurrent Programming Models
Concurrent Programming Models facilitate parallel execution of tasks, harnessing multi-core processing capabilities. In the realm of structured programming languages, concurrency enables efficient resource utilization and improved system responsiveness. Implementing concurrent models enhances program performance and scalability, vital for modern software applications. However, managing shared resources and synchronizing concurrent processes necessitate meticulous planning and synchronization mechanisms.
Distributed Systems
Distributed Systems decentralize data processing across networked nodes, enabling scalable and fault-tolerant applications. Structured programming methodologies align with distributed systems by promoting modular designs and efficient communication protocols. The resilience and performance scalability of distributed computing benefit from structured programming principles, ensuring robust functionality and data integrity. Mitigating latency and ensuring data consistency present challenges that structured programming addresses through optimized system architectures.
Event-Driven Architectures
Event-Driven Architectures orchestrate system behavior based on triggered events, enhancing responsiveness and adaptability. Within the structured programming landscape, event-driven design fosters modular and decoupled systems, facilitating flexibility and extensibility. Implementing event-driven paradigms optimizes resource allocation and improves system resilience, crucial for dynamic software environments. However, managing event propagation and ensuring event consistency require careful event handling mechanisms and robust error-handling strategies.