Exploring the Interplay of Algorithms and Data Structures
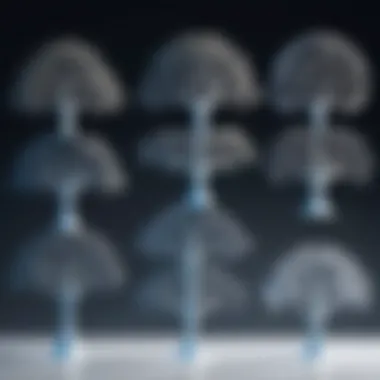
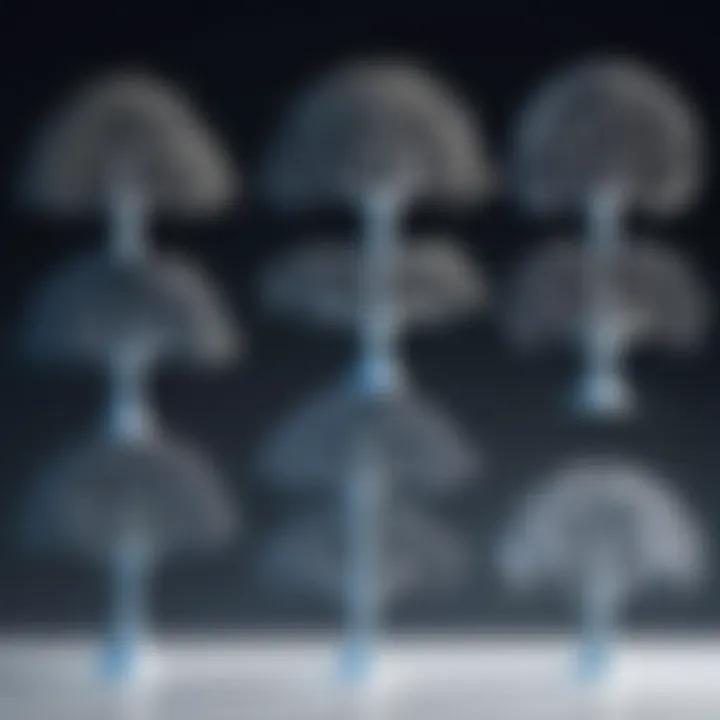
Intro
In the realm of computer science, algorithms and data structures form the backbone of efficient programming and problem-solving. Understanding their interconnectedness can significantly enhance one's ability to design and optimize software solutions. An algorithm acts as a clear set of instructions for performing tasks, while data structures provide a way to store and organize data.
This article explores these relationships, covering the definitions of these critical concepts, alongside their implementations in various technologies. From analyzing algorithmic efficiency to discussing data organization and massive datasets' management, we aim to equip readers with both a theoretical and practical foundation in these essential areas.
Understanding Storage, Security, or Networking Concepts
Prologue to the basics of storage, security, or networking
In modern computing, grasping the fundamentals of storage, security, and networking proves vital. Storage refers to the mechanisms and devices used to retain data. Security is about organizing defenses against unauthorized access or disruption to information, while networking describes how devices communicate and transfer data.
Key terminology and definitions in the field
To navigate through these areas effectively, here are relevant terms that one should understand:
- Data Storage: Conversion of information into a format that can be saved.
- Network Protocols: Rules governing data exchanges in networks.
- Encryption: Transforming data into a secure format to prevent unauthorized access.
Overview of important concepts and technologies
Technologies such as cloud storage have transformed how we manage piles of data, offering accessibility and flexibility. Security technologies like firewalls play a crucial role in preventing breaches. In networking, concepts like bandwidth and latency shape how well users experience or retrieve data, allowing for efficient data transfer.
Best Practices and Tips for Storage, Security, or Networking
Tips for optimizing storage solutions
- Choose appropriate storage solutions based on required access speed and data permanence.
- Implement redundant data storage systems to prevent information loss.
- Regularly check storage analytics for improved efficiency.
Security best practices and measures
- Employ strong authentication methods to guard entries against unauthorized users.
- Regularly update software to protect against newly discovered vulnerabilities.
- Educate employees about phishing attacks and other prevalent threats.
Networking strategies for improved performance
- Optimize the network layout to minimize latency and congestion.
- Use content delivery networks (CDN) for rapidly distributing content.
- Consider virtual private networks (VPN) for secure transmissions between networks.
Industry Trends and Updates
Latest trends in storage technologies
Current storage infrastructures lean towards decentralized and cloud-based systems. More companies are shifting to hybrid models to balance performance and cost. Tech such as non-volatile memory express (NVMe) enhances storage speed.
Cybersecurity threats and solutions
Close monitoring of malware strategies helps tech firms combat continuous threats. AI-driven security solutions increasingly provide real-time surveillance and user behavior insights, making systems more responsive than ever.
Networking innovations and developments
The movement towards 5G networking promises astounding speed and efficiency. It fundamentally advances IoT capabilities, enabling innumerable devices to interact effectively and instantaneously.
Case Studies and Success Stories
Real-life examples of successful storage implementations
Companies like Dropbox and Google Drive have scaled their services by ensuring efficient storage solutions and redundancy. They deploy recognized protocols to minimize downtime.
Cybersecurity incidents and lessons learned
The Equifax data breach exposed fundamental flaws in cybersecurity strategies. Rigorous updates might have prevented breaches and raised overall security practices in responding organizations.
Networking case studies showcasing effective strategies
Companies using SD-WAN technologies have successfully optimized their networks, ensuring efficiency across multiple locations and significantly reducing costs.
Reviews and Comparison of Tools and Products
In-depth reviews of storage software and hardware
Examining tools like AWS S3 storage solutions provides insights on how scalable cloud systems can benefit modern companies managing large data.
Comparison of cybersecurity tools and solutions
Analyzing industry-leading cybersecurity products, including Symantec and McAfee, offers a view into their capabilities and user experiences, shedding light on pros and cons of each.
Evaluation of networking equipment and services
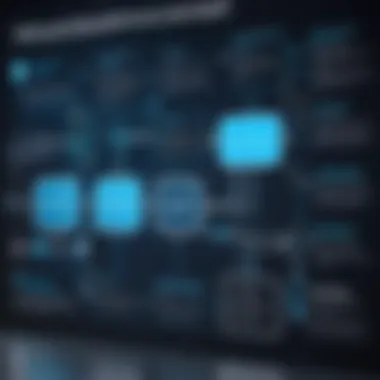
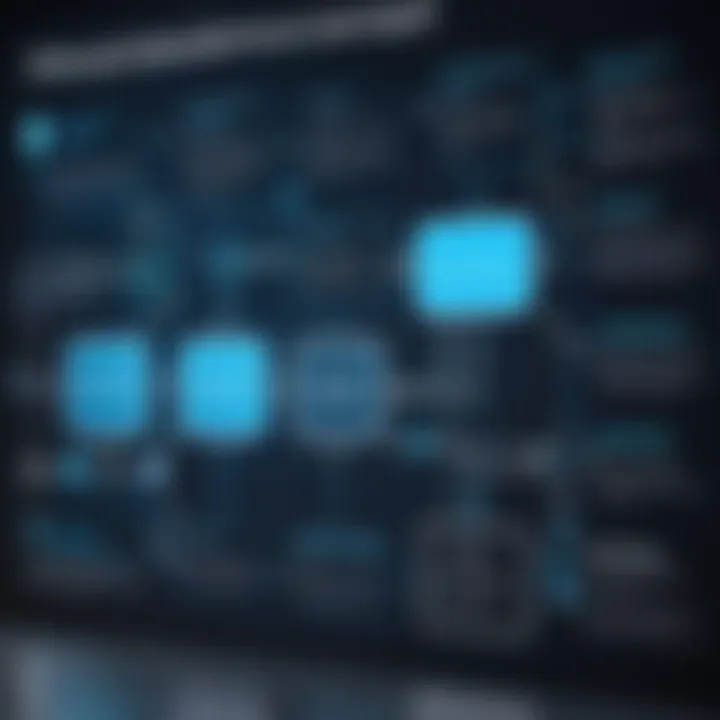
Cisco devices are often evaluated against competitors, with thorough assessments of performance, security features, and durability, helping businesses make informed decisions.
Understanding algorithms and the related data structures provides the foundation for everything from basic Programming to complex software development.
Prelims to Data Structures and Algorithms
Understanding the intricate relationship between data structures and algorithms is vital for professionals in the field of computer science. These two components form the backbone of programming and software development. This section seeks to elaborate on the essential aspects of data structures and algorithms, revealing their significance and why they are essential for effective coding and problem-solving.
Defining Key Terms
Definitions serve as foundational elements for understanding any subject. In this context, a data structure is organized data that allows computers to manage and store things systematically. Common examples include arrays, linked lists, stacks, queues, and trees, among others. On the other hand, an algorithm is a finite sequence of well-defined instructions to solve a problem or perform a specific task. The term often relates to the method used for manipulating data stored within a data structure.
The Importance of Data Structures
Data structures are critical as they enable developers to efficiently manage large amounts of data. Properly chosen data structures help in optimizing space and access times.
- Consider the following purposes of data structures:
- To store data effectively.
- To retrieve data quickly.
- To facilitate operations necessary for solving specific problems.
The implications of using appropriate data structures can be evident in areas such as speed and performance, essential especially in large-scale applications where efficiency is paramount. Both the choice and structure underpinning how data is stored and retrieved significantly impact the overall performance of applications.
The Role of Algorithms in Programming
Algorithms play an instrumental role in the computing landscape. They not only dictate how a data structure operates but also determine how quickly and efficiently a program runs.
Some key points about algorithms include:
- Guidance: Algorithms instruct computers on how to process data, serving as blueprints for action.
- Variability: Different algorithms can lead to different outputs for the same input data depending on the situation they are applied.
- Time Complexity Considerations: The performance of various algorithms can differ widely, underscoring the importance of selecting the right one for a given task.
In summary, a deep understanding of the relationship between algorithms and data structures enables professionals to devise solutions to complex coding challenges and enhances programming proficiency.
Types of Data Structures
Understanding the types of data structures is crucial in computer science and software development. Each data structure has its unique features, which dictate its use case and efficiency. Knowing the strengths and weaknesses of different structures helps in making informed decisions about their implementation, enhancing algorithm performance, and optimizing resource management.
Linear Data Structures
Arrays
Arrays are among the simplest and most widely used data structures. The most significant aspect of arrays is that they store a collection of items in a contiguous memory space. This allows constant-time access to elements via their index, boosting execution speed especially in retrieval operations. The fixed size of arrays makes them beneficial for applications where the number of elements is known beforehand.
However, arrays also present challenges. When needing to add or remove elements, one often has to shift several entries. This results in linear time complexity for operations that are dynamic in nature. Understanding howto manipulate arrays is essential for any software development.
Linked Lists
Linked lists provide a dynamic approach for storing data. Unlike arrays, the size of a linked list can adjust during program execution. This is achieved by connecting each item to the next through pointers, allowing for efficient insertions and deletions. Linked lists are considered a good choice when dealing with data whose size is unpredictable or when frequent modifications are typical.
However, the main downside of linked lists is that they require additional memory for their pointers. Furthermore, access time is generally slower compared to arrays since it involves traversing nodes. Still, linked lists are an integral topic for any computer science professional.
Stacks
Stacks function on a Last In, First Out (LIFO) principle. This structure is inherently simple, allowing only the addition or removal of elements from the top. They work well in scenarios involving recursion, backtracking, or reverse processes such as undo operations in applications. Their simplicity makes understanding flow control possible, serving as a foundation for algorithms.
On the downside, stacks have their limitations since they only provide access to the most recent entry, making random access impossible. This could hinder performance in situations requiring frequent data retrievals from different points in the stack.
Queues
Queues operate on a First In, First Out (FIFO) principle. They help manage tasks in a manner that respects the order of entry. This can be vital in scenarios requiring resource management and load distribution, such as print jobs or task scheduling. Queues promote better efficiency in real-time systems where each process needs to be attended to in the sequence it was assigned.
However, as with stacks, the linear access pattern limits performance in certain situations, particularly where data needs to be accessed out of order. Queues require space management strategies to ensure that they maintain the needed number of entries without overflow.
Non-Linear Data Structures
Trees
Trees are influential in organizing data hierarchically, with nodes representing connections between values. A key feature of trees is their ability to facilitate swift searches, especially in binary trees. Tree structures are particularly beneficial in implementing tasks such as parsing expressions and managing directories.
One limitation is that unbalanced trees can degenerate, leading to longer paths and increased search times. Balanced trees, such as AVL and Red-Black trees, mitigate this effect but involve additional management complexities that must be navigated.
Graphs
Graphs can represent intricate relationships through nodes joined by edges. This versatility allows for modeling many real-world problems, such as social networks and web connections. The diversity of graph types supports various traversal strategies, making them broad ranging in applications.
Nonetheless, the representation of graphs can be cumbersome, requiring significant memory and processing efforts for very large datasets. Algorithms like Depth-First Search or Breadth-First Search can then be indispensable for graph traversal.
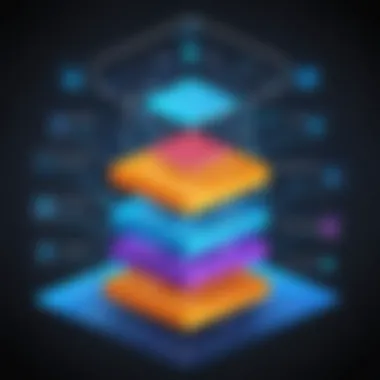
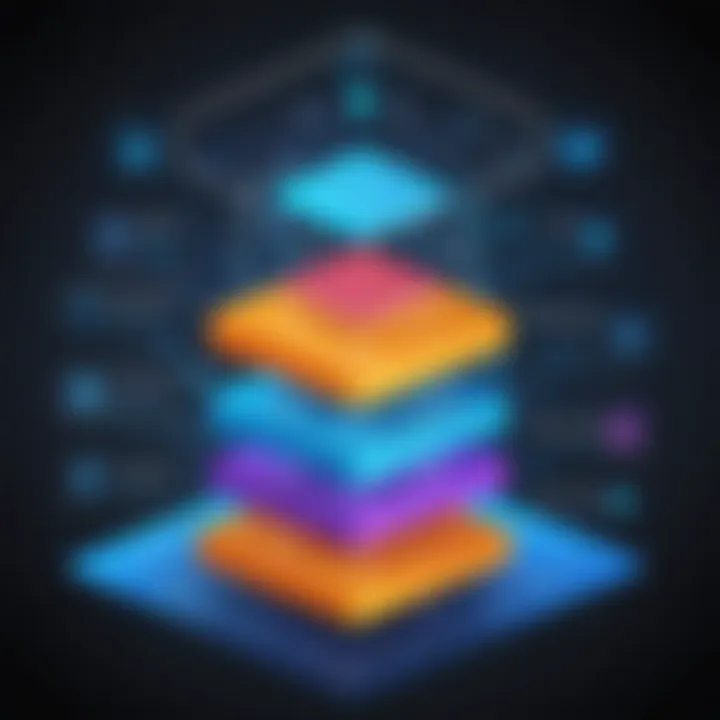
Hash Tables
Hash tables excel in enabling fast data retrieval based on unique keys. Their primary characteristic is the hashing function that transforms keys into indexes, dramatically reducing access time in average cases. This is particularly effective in systems needing efficient storage, such as caching or database indexing.
The downside, however, is that collisions can occur when multiple keys generate the same index. Handling such conflicts requires strategy, often increasing complexity in implementation. Yet, their fast performance remains unmatched in many scenarios, making hash tables a critical study topic for developers.
Fundamental Algorithms
Fundamental algorithms are essential components of computer science that govern how solutions to problems are constructed using data. Their importance stems from the fact they provide a structured way to process and analyze data, significantly impacting the efficiency of applications and systems. Efficient algorithms can enhance system performance and optimize resource usage. Thus, an understanding of fundamental algorithms is vital for anyone engaged in programming or system design.
Sorting Algorithms
Quick Sort
Quick Sort is a highly efficient and popular sorting algorithm due to its average-case runtime performance, estimated at O(n log n). The most notable characteristic of Quick Sort is its use of the divide-and-conquer methodology, which breaks the array into smaller sub-arrays. Each element is compared, resulting in data that is sorted more efficiently than many alternative algorithms.
Quartz is beneficial for large datasets and is a common choice in scenarios where various performance metrics need to be prioritized, such as speed and memory usage. A unique feature of Quick Sort is that it performs in-place sorting, meaning it requires little additional memory space, which is crucial for optimizing resource consumption.
However, it does have disadvantages. In the worst-case scenario, Quick Sort experiences a time complexity of O(nยฒ) if pivot chosen leads to poor partitioning. Care in choosing pivot can enhance performance. Even with its drawbacks, Quick Sort remains a vital part of efficient data sorting operations.
Merge Sort
Merge Sort retains a significance in computer science thanks to its reliable sorting mechanism with an average-case complexity of O(n log n). It operates through merging sorted sub-arrays together, ensuring a stable sort which can be very important in certain situations. This algorithm is particularly advantageous when dealing with linked lists, because it does not require random access.
The unique advantage of Merge Sort lies in its stability, which preserves the original order of equal elements. However, from a spatial consideration, Merge Sort is not optimal as it requires extra space equivalent to the size of the input being sorted, which can present challenges in constrained memory environments. Yet, its ability to handle large data reliably makes it a choice to consider in many applications.
Bubble Sort
Bubble Sort is often never used for large scales but familiar because it's one of the simplest sorting algorithms to comprehend. It operates through repeated traversals of the list, swapping adjacent items when they are in the incorrect order, thus resulting in a final sorted array. The best-case scenario for its efficiency lands at O(n).
Bubble Sort has a clear advantage of easy implementation, often incorporated into introductory programming tasks to ease beginners into algorithmic thinking. Nonetheless, its efficiency is notably lesser, noted for lethargy with large datasets where performance subsists mainly in O(nยฒ) time complexity in the average and worst-case scenarios. For any practical use where performance is critical, other more efficient algorithms should be regarded rather than Utility of Bubble Sort.
Searching Algorithms
Binary Search
Binary Search boasts a powerful role due to its efficiency when seeking a target value within a sorted dataset. Its key characteristic involves repeatedly dividing the search interval in half which leads to a time complexity of O(log n). As the dataset size increases, the measuring increases made possible by its fundamental structure support quick conclusions.
With Binary Search's application, efficiency remains critical in large databases where speed is paramount. A downside, though, arises if the data is not sorted beforehand, resulting in inefficiency. Some studies even claim it steadies other search methods this advantage solidifies its reputation as a standard in search algorithms.
Depth-First Search
Depth-First Search (DFS) provides foundational behavior for traversing and exploring tree-like or graph-like structures. One primary trained characteristic of DFS includes utilizing a stack to keep track of it progresses deeper into the structure before backtracking when peering into various paths. Its time complexity sits at O(V + E), where 'V' reflects the specific vertices and 'E' must correlate.
DFS is preferred in many situations due to its ability to capture every possible path until they found cessation or a solution possibly relevant appears, often discovering artificial intelligence, puzzles, or path-finding solutions. However, poor memory management could cost resources without a conservative tree buildup so careful provision should realize limitations is helpful especially when utilizing recursive approach.
Breadth-First Search
Breadth-First Search (BFS) serves a distinct morphological character where width iterations allow the approach towards exploring nearby nodes before sequencing deeper. Encounter expectations such as recovering the shortest paths within graph iterations direct as it searches through volumetric reasons prefer execution stack use and occupy greater interim memory relative initial locational scope. The visualized effectiveness stands in value often related yet merits greedier gathering alongside memory systems with its distinction primary O(V + E).
Like its cousin DFS, it learns expected tasks valuably fostering efficiency and solving-related problems suitable109. Running usability notices on cyclical aspect minimally can present clashes. Avoid titan overload concepts, assess technique control memory factor account as management strategy safeguards choices lies in performance ุชููู ั ะพัะพัะตะผ.
Algorithm Efficiency
Understanding algorithm efficiency is critical in today's complex programming environment. As data size and application requirements grow, optimizing algorithms can significantly improve performance. Efficiency not only influences execution speed but also afects resource utilization. Industry strategies increasingly depend on the ability to analyze and refine algorithm performance, making this topic vital.
Big O Notation
Big O Notation is a language used to describe an algorithm's efficiency in terms of time or space as the input size increases. This notation is essential for comparing algorithms and determining their scalability.
- Time Complexity: Time complexity expresses how the execution time of an algorithm escalates relative to the input size. Common Big O classifications include:
- Space Complexity: This aspect refers to the amount of memory an algorithm consumes relative to the size of the input. Like time complexity, evaluating space complexity also employs Big O notation.
- O(1) - Constant Time
- O(log n) - Logarithmic Time
- O(n) - Linear Time
- O(n log n) - Linearithmic Time
- O(n^2) - Quadratic Time
Being familiar with these classifications aids developers. Knowing whether an algorithm is more efficient can inform crucial decisions in implementation.
Analyzing Time Complexity
Time complexity analysis connects directly to Big O notation. When developers analyze algorithms, the focus is on identifying the upper limit on growth rate concerning time.
- Best Case: The least amount of time an algorithm will take.
- Worst Case: The maximum time taken for any input.
- Average Case: A blend of the best and average performance given a random input distribution.
A thorough understanding of these cases helps developers predict how an algorithm will behave under various scenarios, ensuring better design in applications.
Space Complexity Considerations
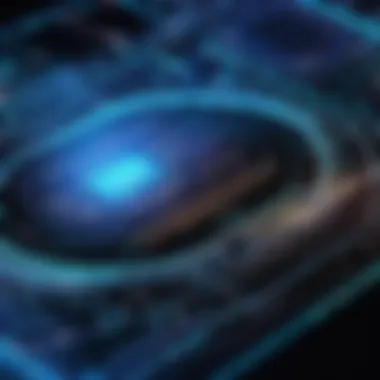
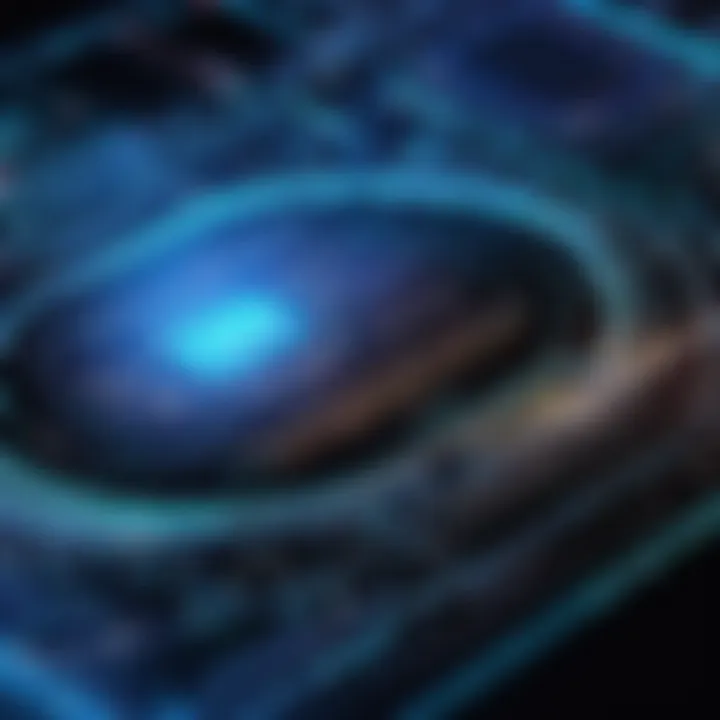
Developing efficient algorithms means more than focusing on time. Space complexity involves balancing algorithm efficiency with memory usage. A well-optimized algorithm should seek a solution that conserves memory without sacrificing speed.
- Common space complexities include:
- O(1): Requires a fixed amount of space regardless of input size.
- O(n): Space grows linearly with input size.
Questions arise about whether to favor time over space, or vice versa. This decision strongly depends on the situation.
"Efficient use of space often leads to manageable systems, allowing for scalability while minimizing costs."
In summary, analyzing algorithm efficiency is essential not just for performance, but also for ensuring applications can handle growth without excessive resource consumption. An algorithm's time and space complexity need regular revisiting, especially as technologies advance. Effective calculations mean improved programming solutions in the long run.
Advanced Data Structures
Advanced data structures are vital for efficiently managing complex data handling needs in computer science. They improve the speed and effectiveness of algorithms and enhance overall application performance. Their role is profound, facilitating complex data organization, search operations, and manipulations that are perfomed regularly in software development. For instance, using advanced data structures ensures that algorithms can execute more efficiently by optimizing both time and space complexities. These elements are particularly crucial in systems where speed and resource management are paramount.
Balanced Trees
AVL Trees
AVL trees are a type of self-balancing binary search tree. Their name comes from the initials of their inventors, Georgy Adelson-Velsky and Evgenii Landis. The main idea behind AVL trees is to maintain a height balance factor of each node, which guarantees that the depth of the tree is always low. A critical aspect of AVL trees is that after any modification, such as insertion or deletion, they automatically rebalancing themselves through rotations. This ensures O(log n) time complexity for basic operations like insertion, deletion, and look-up. This key characteristic makes AVL trees a popular choice when frequent insertions and deletions occur in limited timeframes. Their performance can degrade, however, during rebalancing, making it suitable primarily for environments that require a predictable and balanced structure, yet demanding the insertion rate is moderate.
Red-Black Trees
Red-black trees are another type of self-balancing binary search tree yielding good performance in dynamic settings. A distinguishing characteristic of red-black trees is that each node carries an attribute indicating whether it's red or black. This assists in keeping the tree balanced during operations. The unique feature of red-black trees lies in the color-based balancing rules that help ensure that no path in the tree is more than twice as long as any other path. This gives red-black trees consistently O(log n) time complexity across various operations. They are favorable in contexts where frequent balance maintenance is more viable, particularly in environments with numerous iterations. Nonetheless, their complexity tends to be higher than that of AVL trees, which could hinder performance in applications where tree height is significantly more pivotal than balance constraints.
Tries and Suffix Trees
Tries and suffix trees are specialized data structures optimized for managing large sets of strings. Tries are tree-like structures where each node represents a common prefix shared by some strings. They allow speedier searches, closely related to the length of the input strings rather than the number of entries. This characteristic is particularly beneficial when dealing with extensive dictionaries or implementing autocomplete features in applications. Here, a unique advantage is realized in reduced redundancy storing and retrieval involving repeated prefixes.
Suffix trees, on the other hand, are most effective in various string processing problems, including searches for substrings or patterns in given texts. Such trees allow for quickly finding complex patterns with minimal traversals. A noted feature is providing efficient sub-string searches in linear or low polynomial time, which significantly boosts performance when analyzing genetic sequences or web p.Cursor traversal through large datasets becomes smoother with them, greatly easing querying operations in various systems.
Applications of Algorithms and Data Structures
The realm of algorithms and data structures presents an array of vital applications across various fields in technology and beyond. Understanding these applications is crucial for IT professionals and students alike. They represent not only the foundation of effective programming but also the pillar upon which efficient system design stands. Algorithms dictate the procedures we use to process procedures, especially when paired with appropriate data organization tools offered by various data structures. The convergence of these components leads to enhanced performance and innovation in software development, risk management, and overall data handling.
Use in Software Development
Software development necessitates well-structured algorithms and data structures to create efficient applications. The proper use of algorithms results in refined working methods, which improve computing resources, speed up data processing, and lead to more resilient code. Here, it is essential to select suitable data structures according to problem specifications. For instance, linked lists can be effective for applications requiring high-frequency inserts and deletes, whereas arrays function productively for indexed access to data.
Some key points include:
- Performance Optimization: A well-implemented algorithm can reduce execution time and save cost on computing resources.
- Maintainability: Efficient structure and algorithms contribute to more understandable and maintainable codebase configurations.
- Error Reduction: Carefully crafted algorithms minimize the weight of data manipulation tasks and subsequently decrease the odds of trapping erroneous situations.
Additionally, software frameworks utilize rich collections of algorithms and data structures to ascertain the best operational outcome for specific tasks. Choosing the right system construction ensures versatility and scalability as software systems grow in complexity.
Applications in Machine Learning
Machine Learning (ML) heavily relies on algorithms and data structures for effective function. Various data movements, manipulations, and storage benefit from this synergy. Algorithms are the mathematical foundations driving learning processes. In contrast, advanced data structures efficiently store immense data volumes processing crucial to identify patterns, correlations, and structures present within large datasets.
In the ML realm, consider:
- Feature Extraction: Using algorithms tailored for extracting data features helps refine the inputs leading to better insights.
- Model Optimization: Advanced algorithms can structure data more effectively โ this, in turn, means optimization in training machine learning models and less redundancy.
- Processing Big Data: Effective data structures address considerable complexity inherent in big data, locating trends and info to process quicker and validate faster outcomes.
The interplay between algorithms and data structures creates impactful tools for managing myriad data types. Tighter efficiency becomes attainable, allowing for adaptability across factual requirement shifts.
The efficacy of a program is fundamentally defined by its underlying algorithms and how harmoniously they operate with its data structures.
End and Future Trends
The relationship between algorithms and data structures is not static; it evolves continuously. Its implications for computer science are far-reaching, impacting software design, user experience, and solution efficiency. As the field progresses, understanding the conclusion and future trends is critical for those engaged in information technology or software development. This section outlines the essence of retrospection on current projects, as well as preparing for the complexities of future advancements.
Summary of Insights
The exploration of algorithms and data structures revealed several essential insights:
- Interdependence: Algorithms rely on well-structured data. Their performance deteriorates without suitable data organization.
- Efficiency: Understanding algorithms' complexities helps in creating faster and resource-efficient applications.
- Real-World Application: From machine learning to cybersecurity, the applications of data structures are diverse and critical for system performance.
โThe efficiency of data organization can define success in real-time computational tasks.โ
By comprehending these fundamentals, IT professionals can adapt and innovate in a fast-paced environment.
Emerging Trends in Algorithms
Several significant trends in algorithms are emerging as technology marches forward:
- Artificial Intelligence: More algorithms are designed with AI capabilities to adapt and learn, allowing them to solve complex problems more efficiently.
- Quantum Algorithms: These promise to revolutionize processing capabilities, particularly in large-scale data analysis and encryption.
- Distributed Algorithms: As cloud computing and distributed systems grow, the emphasis on algorithms that efficiently process data across networks increases.
- Create Adaptive Algorithms: Algorithms that learn from data patterns provide better outputs and adaptability in real-time decision making.
In each of these trends, the underlying data structures play a role. Their organization remains crucial for effective algorithmic performance as demands increases.
By staying informed on these developments, professionals can ensure their methods remain relevant and prioritize the optimized use of both algorithms and data structures.