Understanding HTTP REST APIs: A Comprehensive Guide
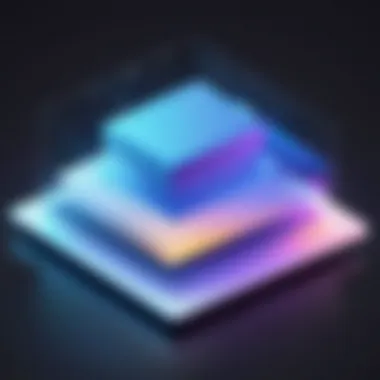
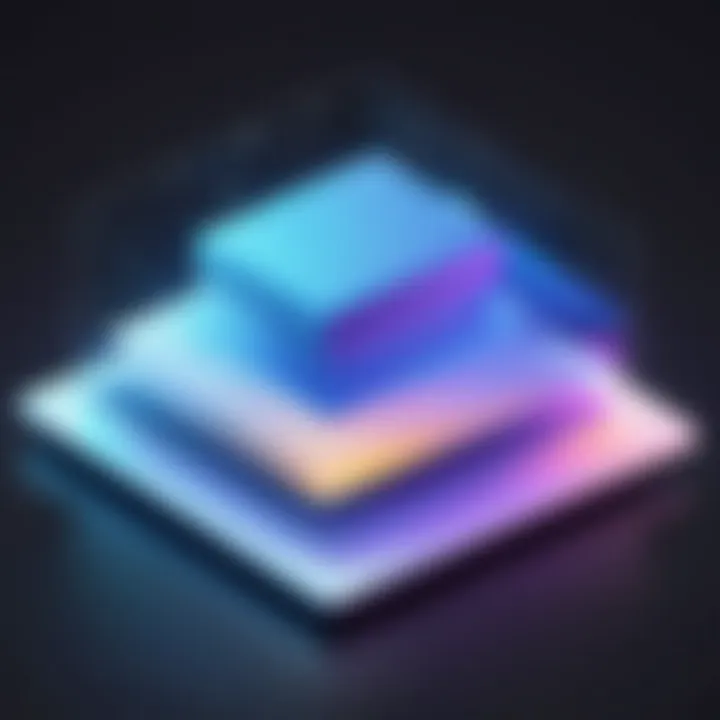
Intro
In the modern landscape of software development, understanding the principles behind HTTP REST APIs serves as a cornerstone for effective communication between various systems. Application Programming Interfaces (APIs) enable different software applications to interact seamlessly. While this might seem quite technical, the core components can be grasped with clarity and precision. This section provides a foundational understanding of the core concepts of HTTP REST APIs, setting the stage for further exploration.
REST, which stands for Representational State Transfer, is an architectural style that simplifies the development of networked applications. Designed to promote scalability and performance, it employs a stateless communication protocol—primarily HTTP. This allows different systems and platforms to communicate without maintaining an ongoing state between requests, leading to enhanced flexibility and lower resource consumption.
In grasping the essential principles of this model, it is crucial to define the terminology specific to REST APIs. Terms like "resource," "endpoint," and "CRUD operations" are foundational.
- Resource refers to a data entity or piece of information that can be accessed via the API.
- Endpoint is the URL through which the resource can be interacted with.
- CRUD operations encompass the basic operations—Create, Read, Update, and Delete—that can be performed on a resource.
With an understanding of these terms, we can start delving deeper into the technicalities of how HTTP methods function within RESTful services.
REST APIs leverage standard HTTP methods, which include GET, POST, PUT, and DELETE. Each of these methods corresponds to a specific action that can be performed on the resources. For example, GET retrieves information, POST creates a new resource, PUT updates an existing resource, and DELETE removes a resource from the server. Recognizing these methods is vital for effective API interactions.
The design principles of REST focus on resource identification, stateless operations, and the application of standard HTTP methods for efficient interactions.
Understanding these foundational concepts is essential not only for software developers but also for cybersecurity professionals who ensure the security and efficiency of these systems. As we progress through this guide, we will expand on these concepts, exploring practical applications and best practices, ultimately equipping readers with a comprehensive knowledge of HTTP REST APIs.
Foreword to HTTP REST APIs
HTTP REST APIs are crucial in the realm of modern web development. These interfaces define how different software applications communicate over the internet. Understanding their function and structure allows developers to create and maintain adaptable systems used by countless applications.
REST stands for Representational State Transfer. It is an architectural style that facilitates easy interactions between clients and servers over HTTP. RESTful APIs have gained prominence due to their simplicity and efficiency. These interfaces support various data formats and operations, enhancing versatility in application integration.
Key elements of REST API include resources, which are identified by URIs, and standard HTTP methods like GET, POST, PUT, PATCH, and DELETE. Each method serves a specific purpose, reflecting the different types of operations that clients can perform on the resources.
Adopting RESTful APIs brings several benefits:
- Scalability: Systems can grow in complexity without losing performance.
- Statelessness: Each request from a client contains all necessary information, which simplifies the interaction.
- Layered System: The architecture allows for intermediary layers that can handle security, caching, or load balancing without affecting client-server interaction.
Understanding these principles not only facilitates easier development but aids troubleshooting and optimization of web services.
Defining REST
REST is not just a set of guidelines; it is a philosophy that underpins the design of web services. It emphasizes a stateless communication model where each request is treated independently. This approach eliminates the burden of retaining session state on the server, thus enhancing performance. REST relies heavily on standard HTTP methods, which conform to the principles of CRUD (Create, Read, Update, Delete). Its implementation can vastly improve data interchange efficiency between systems.
Evolution of APIs
The evolution of APIs is a story of innovation and adaptation. Earlier APIs were primarily SOAP (Simple Object Access Protocol), which required complex XML messaging. While SOAP offers strong security features, it can be cumbersome compared to REST, which popped up as a simpler alternative. With the advancement of web technologies and the growing demand for lightweight solutions, RESTful APIs emerged as a response to these needs.
The development of REST APIs has transformed how applications interact. Their adoption has opened doors for rapid development cycles and collaborative integrations across diverse platforms. As businesses shift towards mobile and cloud services, REST APIs remain at the forefront, facilitating streamlined connectivity and improved user experiences.
The rise of REST APIs marks a significant transition in the design and utilization of web services, promoting seamless interactions between various software systems.
Core Principles of REST
Understanding the core principles of REST is key for anyone delving into the world of APIs. These principles shape the design, implementation, and scalability of RESTful services. They provide a robust framework that ensures efficient communication between client and server. Each principle plays a vital role in establishing a system that is easy to use, maintain, and extend. Such clarity is crucial, especially for IT professionals and students who need to grasp these concepts to develop effective applications.
Statelessness
One of the fundamental principles of REST is statelessness. In a stateless architecture, each request from a client to the server must contain all the necessary information for the server to understand and process the request. This means that the server does not store any session information about the client. Each interaction is independent, which simplifies the server design. Statelessness promotes reliability and scalability. Since servers do not manage state, they can be easily replaced or scaled without affecting the overall system.
"Statelessness allows APIs to be more flexible and recover from failures quickly."
Client-Server Architecture
REST is based on a client-server architecture that separates the user interface concerns from the data storage concerns. This separation allows the client and server to evolve independently. Clients are responsible for the interface and user experience, while servers manage data and business logic. This design leads to a more modular system. Changes can be made on either side without impacting the other. In practice, this means developers can innovate and deploy new features without having to overhaul the entire system.
Cacheability
Cacheability is a principle that enhances performance and reduces latency. When responses are cacheable, clients can store responses for reuse, thus minimizing repetitive requests to servers. This can lead to significant improvements in load times and server efficiency. However, for cacheability to work effectively, responses must include explicit Cache-Control headers that indicate whether and how the response can be cached. This allows clients to make intelligent decisions about what data to store and for how long, balancing performance with the need for fresh data.
Layered System
The layered system principle entails that a client cannot ordinarily tell whether it is connected directly to the end server or an intermediary. This can include load balancers, proxies, or gateways. The layered approach provides flexibility and enhances system scalability. For instance, it allows for the introduction of new layers to manage load distribution without altering the client’s interaction with the server. Security layers can also be implemented easily, adding another dimension to the architecture’s robustness.
Understanding these core principles is essential for anyone working with REST APIs. They form the backbone of the architectural style and dictate how APIs should be built to optimize performance, scalability, and user experience. By adhering to these principles, developers can create APIs that are not only functional but also capable of evolving to meet future needs.
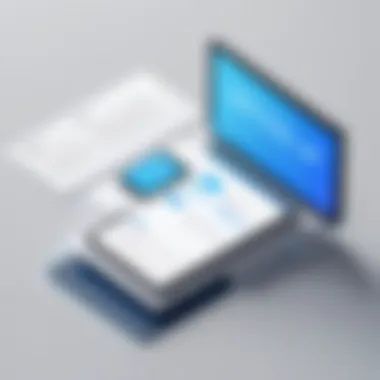
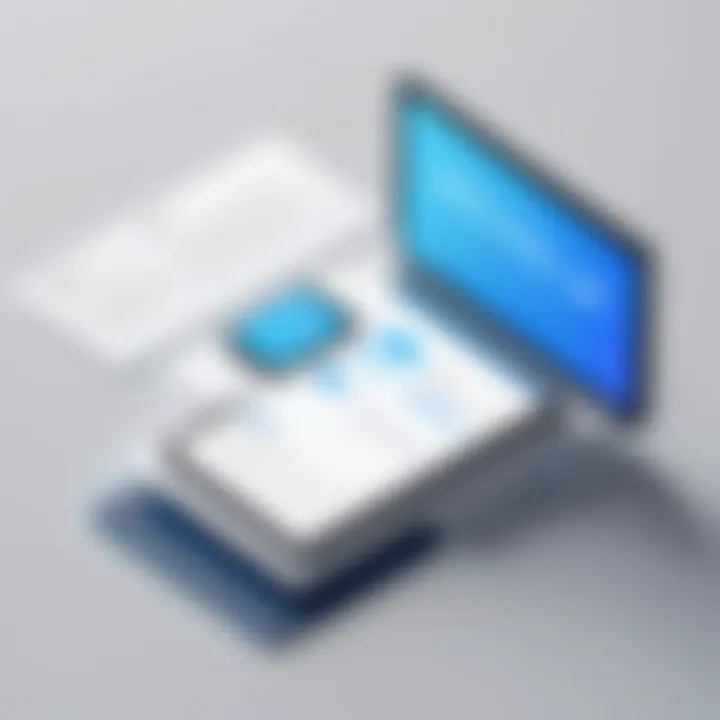
HTTP Methods in REST APIs
Understanding HTTP methods is crucial for working with REST APIs. These methods define the operations that can be performed on resources. Each HTTP method has a specific purpose and holds significance in how the RESTful architecture works. Employing the right HTTP method allows for efficient communication between clients and servers, enables standardization in API design, and improves the overall user experience. Consequently, a solid grasp of these methods can greatly enhance application development and integration capabilities.
Overview of HTTP Methods
HTTP methods specified in the REST architectural style include GET, POST, PUT, PATCH, and DELETE. These methods form the foundation for client-server interactions in a RESTful API. Each method maps to a particular action, allowing developers to perform CRUD (Create, Read, Update, Delete) operations. Let's take brief look at each:
- GET: Retrieve data from the server.
- POST: Send new data to the server.
- PUT: Update existing data.
- PATCH: Apply partial modifications to data.
- DELETE: Remove data from the server.
These methods create a clear and concise way to manage resources, making the structure of requests intuitive and straightforward.
GET, POST, PUT, PATCH, DELETE
GET: This method is typically used to retrieve information from a server. For example, a client may request user details with a GET request. It is essential that GET requests are safe and idempotent, meaning they should not alter the server state and can be made multiple times without affecting the outcome.
POST: This method is appropriate for creating new resources. When a client uses POST, it sends data to the server, which processes the request and may create a new resource in response. It is not idempotent, as sending the same request multiple times may create additional resources.
PUT: PUT is used for updating existing resources entirely. When a client sends a PUT request, it must include the complete representation of the resource. This method is idempotent, so multiple identical requests will yield the same result, ensuring the resource's state remains consistent.
PATCH: Unlike PUT, PATCH is used for partial updates. It allows a client to send only the data that needs changing without affecting the whole resource. This method is also considered idempotent, but care must be taken to ensure that the request format is correct to avoid unintended modifications.
DELETE: This method is straightforward; it instructs the server to remove a specific resource. Like GET and PUT, DELETE requests are idempotent; thus, invoking them multiple times will produce the same result.
The ability to effectively utilize these methods is imperative for developers engaging with REST APIs. By adhering to these principles, developers can create more reliable and efficient applications.
Data Formats in REST APIs
Data formats play a crucial role in the functionality and interoperability of REST APIs. The choice of data format affects how data is processed, transmitted, and understood by both the client and server. The two most commonly used formats in REST APIs are JSON (JavaScript Object Notation) and XML (eXtensible Markup Language). Each format offers distinct advantages and limitations, which need to be considered based on the specific requirements of an application.
JSON vs
JSON is favored for its simplicity and ease of use. It is lightweight, making it fast to parse. This efficiency in data transfer can be beneficial for applications that handle large amounts of data. Furthermore, JSON's syntax is derived from JavaScript, which makes it particularly appealing for web applications. With its ability to represent complex data structures using less verbosity compared to XML, developers often prefer JSON for RESTful services.
On the other hand, XML provides more robust features like support for namespaces and validation through schemas. It can accommodate complex data relationships and hierarchies. However, XML tends to be more verbose, which can lead to increased data transfer times. The choice between JSON and XML often hinges on the specific demands of the project, the existing technology stack, and performance considerations.
"The right data format enhances not just the API's performance but also its ease of use and integration with client applications."
Choosing the Right Format
When deciding between JSON and XML, there are several factors to consider:
- Complexity of Data: If the data structure is simple and requires minimal layering, JSON is typically suitable. For more complex or hierarchical data, XML might be needed.
- Interoperability: JSON works seamlessly with modern web browsers and is more widely used in frameworks like React and Angular. XML may be necessary if integration with legacy systems is a concern.
- Data Size: The size of the data being transmitted can influence the choice of format. Smaller payloads improve performance and speed. JSON often leads to smaller file sizes than XML.
- Ease of Use: If ease of use and speed of development are priorities, JSON provides a more straightforward approach due to its clear syntax.
Authentication and Security
Authentication and security in REST APIs are critical aspects that necessitate thorough understanding and implementation. Given the sensitive nature of data transmitted via APIs, ensuring that only authorized users can access specific resources is paramount. Organizations face significant risks if authentication is not adequately managed. A breach can lead to loss of data integrity, unauthorized access to sensitive information, and potentially severe reputational damage.
Several methods exist to implement authentication within REST APIs. These methods are not only about securing access; they also influence the overall user experience and application performance. Therefore, careful consideration must be given to the appropriate choice of authentication mechanism based on the context and requirements of the application.
"Robust authentication strategies are the first line of defense against unauthorized access to APIs."
OAuth 2.
OAuth 2.0 represents a widely adopted framework for authentication in REST APIs. It facilitates token-based access, allowing users to grant third-party applications limited access to their resources without sharing credentials. This protocol enhances security by utilizing access tokens instead of traditional username and password pairs.
The architecture behind OAuth 2.0 involves four main roles: the resource owner, the resource server, the client, and the authorization server. When a user seeks to access protected data, they are redirected to the authorization server, where their identity is verified and consent is obtained. Upon successful authentication, an access token is issued to the client. The client can then use this token to make API requests on behalf of the user. It is crucial that the access token is kept secure and is only transmitted over secure channels.
API Keys
API keys are another common method of authentication. They provide a unique identifier that allows access to an API. Typically, API keys are generated by the service provider and issued to developers upon registration. The key must be included in API requests, often as a query parameter or in the header of the request.
While API keys are relatively simple to implement, they do come with several limitations. They do not support user-based permissions and lack advanced security features compared to protocols like OAuth 2.0. API keys can easily be exposed if they are hardcoded in client-side code. Therefore, it is essential to monitor API usage and implement usage limits to mitigate potential abuse.
SSL/TLS Implementation
The implementation of SSL (Secure Sockets Layer) or TLS (Transport Layer Security) is essential for ensuring the security of data in transit. This layer of encryption prevents eavesdropping and tampering with the data and the requests sent between the client and server.

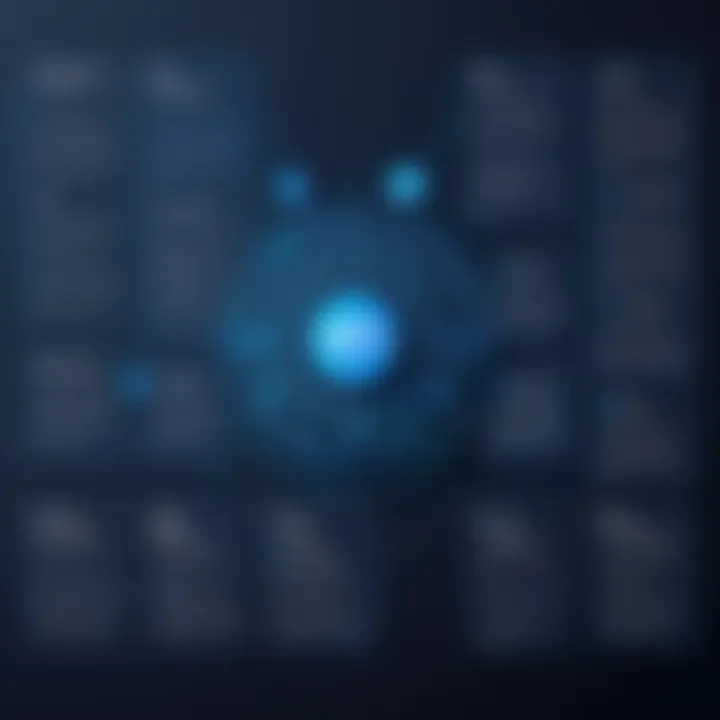
In REST APIs, utilizing SSL/TLS not only safeguards sensitive information like authentication credentials but also enhances the trustworthiness of the API. Users are more likely to interact with an API that demonstrates commitment to security through encrypted connections. Failure to implement SSL/TLS can lead to vulnerabilities where attackers can intercept data and potentially manipulate requests.
Error Handling in REST APIs
In the realm of web services, error handling stands as a critical aspect of HTTP REST APIs. It encapsulates the methods developers use to manage problems arising during client-server interactions. Proper error handling not only improves user experience but also aids in maintaining a robust application architecture. Moreover, effective strategies for error management can minimize downtime and ensure that users receive timely and informative feedback when an issue occurs.
Common HTTP Status Codes
Understanding HTTP status codes is vital for effective error handling. These codes provide insight into the outcome of a client's request. They are standardized and universally recognized, enabling clear communication between the server and client. The most commonly used status codes include:
- 200 OK: The request succeeded, and the server returned the requested data.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 401 Unauthorized: The request requires user authentication.
- 403 Forbidden: The server understands the request but refuses to authorize it.
- 404 Not Found: The server cannot find the requested resource.
- 500 Internal Server Error: The server encountered an unexpected condition that prevented it from fulfilling the request.
These codes are integral in determining the next steps for both the client and server. For example, a status may prompt the client to check the resource identifier for errors, while a may necessitate investigation into server logs.
Creating Informative Error Responses
Informative error responses enhance the user's ability to understand and resolve issues. Instead of returning generic error messages, developers should provide details pertinent to the error encountered. This can include:
- A clear description of what went wrong.
- Suggested actions the user might take to rectify the problem.
- Links to documentation or support resources for further assistance.
For instance, instead of the standard “An error occurred”, a response can be structured as:
This approach not only exemplifies transparency but also builds user trust in the system's reliability.
Key Point: Effective error handling minimizes frustration and enhances user satisfaction. It fosters a positive relationship with users by ensuring they have the information they need to overcome obstacles efficiently.
Versioning REST APIs
Versioning REST APIs is a crucial aspect that influences the evolution and usability of web services. As applications and user requirements change, so do the APIs that support them. When an API undergoes changes, it can impact existing clients that rely on it. This results in potential disruptions and loss of functionality. Thus, implementing a systematic versioning approach is necessary to ensure seamless user experiences and continued service reliability.
Importance of Versioning
The importance of versioning cannot be overstated. First, it allows developers to manage changes without breaking existing functionality. By versioning the API, teams can introduce new features or changes while keeping previous versions active. This flexibility is vital for organizations that have multiple clients, each potentially utilizing different API features.
Another critical aspect is compatibility. Over time, changes to an API are almost inevitable. These changes might include enhancements, deprecations, or bug fixes. If versioning is ignored, clients can face issues, breaking their integratoin processes. This can lead to frustration and loss of trust in the API provider. Building stable relationships with clients necessitates that their needs are met even as the service evolves.
Lastly, versioning promotes clearer documentation. By distinguishing API versions, developers and users can easily navigate which functionalities are available in each version. A clear versioning strategy provides a marketing advantage, highlighting an organization’s commitment to quality and user satisfaction.
Strategies for Versioning
There are several strategies for versioning APIs. The most common methods include:
- URI Versioning: This method integrates the version directly within the API endpoint. For instance, . This approach is straightforward, making it easy for clients to understand which version they are using.
- Query Parameter Versioning: In this method, the version number is added as a query string. For example, . This offers flexibility, enabling clients to specify the version of the API to call without altering the URI structure.
- Header Versioning: With header versioning, clients indicate version preferences through HTTP headers. For example, clients can send a request with a custom header like . This method keeps URIs clean and allows more control over API requests.
When choosing a strategy, consider factors such as client needs, ecosystem complexity, and potential future changes. Each method has its strengths and weaknesses, impacting ease of use and client transparency.
Remember: Without a clear versioning plan, organizations risk alienating their user base and damaging their reputation.
For further reading on REST APIs and their significance in modern web development, visit Wikipedia or Britannica.
Best Practices for API Design
In the rapidly evolving world of technology, well-designed APIs play a critical role in successful software development. Best practices in API design are essential as they ensure usability, consistency, and reliability. Focusing on these practices provides a foundation for developers and companies to build, maintain, and enhance their APIs effectively.
Good API design not only enhances the developer experience but also influences productivity across teams. It eases the integration process with third-party services, minimizes errors, and creates a better user experience. As REST APIs become more prevalent, understanding best practices is becoming increasingly important for any tech professional.
Resource Naming Conventions
Naming conventions for resources in REST APIs are crucial for clear communication and understanding. Clear and logical naming improves the API’s usability, enabling developers to quickly identify the purpose and behavior of each resource.
When defining resource names, consider the following guidelines:
- Use nouns, not verbs: Resources should be represented by nouns. For example, use instead of .
- Be consistent: Consistency in naming conventions across all resources prevents confusion. Choose a format, such as plural nouns, and stick to it.
- Avoid using special characters and spaces: Unintended results may arise from incorrectly formatted URLs. Instead, use hyphens or underscores to separate words if necessary.
An example of a well-structured naming convention could be:
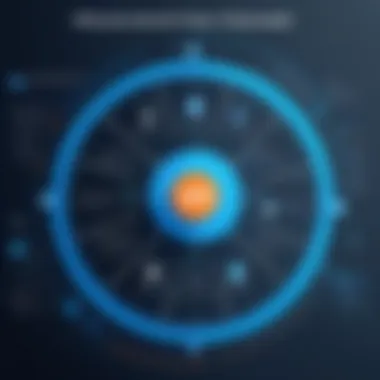
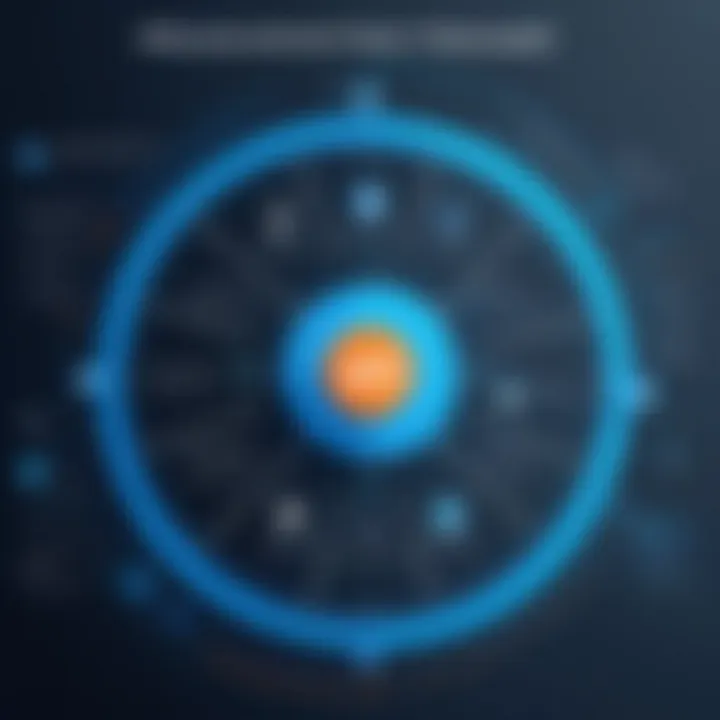
These endpoints clearly define actions associated with the resource, allowing for predictable interactions.
Documentation and User Guides
Well-documented APIs are a necessity for successful implementation. Effective documentation serves as a bridge between the API and its users. It makes it easier for developers to understand how to interact with the API services.
Documentation should include:
- Overview of the API and its capabilities: Clearly outline what the API does. This creates a starting point for new users.
- Usage examples: Practical examples help clarify how to use different endpoints. Including both request and response examples enhances comprehension.
- Error Handling Information: Provide details about common error responses users may encounter, with explanations and suggested solutions.
Using platforms like Swagger can help create dynamic API documentation, allowing users to test API endpoints directly within the documentation.
In essence, investing time and resources into API design best practices promotes stronger collaboration and better functionality for developers across all platforms.
Real-World Applications of REST APIs
Understanding the real-world applications of REST APIs is critical to grasping their value in modern software architecture. REST APIs are the backbone of communication in web services, enabling seamless interaction between different applications and systems. They play a crucial role in various industries, from finance to healthcare, and have transformed how developers build and integrate software solutions. This section explores specific examples and insights related to these applications, showcasing their importance and impact.
Case Studies
Examining case studies provides concrete illustrations of how REST APIs drive innovation and efficiency. One notable example is Stripe, a payment processing platform that has leveraged REST APIs to simplify online transactions. By allowing developers to integrate payment processing into their applications effortlessly, Stripe showcases how APIs can enhance user experience and streamline business processes.
Another important case is Spotify. The music streaming giant uses REST APIs to deliver personalized playlists and recommendations. By exposing their music catalog via APIs, Spotify empowers developers to create immersive applications that integrate with its services, reaching a wider audience.
Moreover, Airbnb utilizes REST APIs to facilitate seamless interaction between hosts, guests, and payment systems. Each API endpoint corresponds to specific functions like booking a home or managing listings, enabling efficient communication and user interaction.
These cases illustrate that REST APIs not only improve functionality but also lead to better customer satisfaction and reduced operational costs.
Industry Insights
Several industry insights highlight the ongoing relevance and adaptability of REST APIs. As businesses increasingly rely on integrations, the need for RESTful services has surged. According to a survey, more than 65% of developers regard REST as their primary API architecture choice. The simplicity and scalability of REST APIs make them suitable for new applications and large-scale enterprise solutions alike.
Furthermore, REST APIs are being increasingly employed in mobile app development. As mobile technology advances, REST APIs provide the necessary framework for connecting mobile applications to backend services. The lightweight nature of RESTful services facilitates faster loading times, which is crucial for mobile user retention and satisfaction.
In addition, the rise of microservices architecture has amplified the significance of REST APIs. Organizations are segmenting their applications into smaller, independent services that communicate via REST APIs, leading to improved maintainability and deployment speeds. This separation allows companies to adapt to changing business needs faster.
"REST APIs have revolutionized how businesses operate and deliver services, proving essential for growth in the technology landscape."
In summary, the real-world applications of REST APIs offer a wealth of knowledge about their effectiveness and necessity in today's digital frameworks. Case studies of companies like Stripe, Spotify, and Airbnb underline the practical benefits, while industry insights signal a bright future for REST API usage across various sectors.
Future Trends in REST APIs
As the technological landscape continues to evolve, the trends surrounding REST APIs undergo significant transformations. These shifts hold important implications for developers and organizations. Understanding these future directions is crucial for anyone involved in web development, data exchange, or software architecture.
GraphQL vs REST
One of the most talked-about trends is the rise of GraphQL as an alternative to REST. Created by Facebook in 2012, GraphQL offers a different approach to API design. With GraphQL, clients can request exactly the data they need and nothing more. This contrasts with REST, where responses often include excessive data, leading to inefficiencies.
GraphQL reduces the number of requests made to the server. Instead of multiple endpoints to fetch related data, a single request can provide all needed information. This flexibility can enhance performance, especially for mobile applications that require limited data usage.
However, it is important to note some cautious considerations when adopting GraphQL. The learning curve can be steep, and managing security in GraphQL needs more focus. REST continues to offer simplicity and robust security practices through established standards.
Serverless Architectures
Another significant trend is the growth of serverless architectures. This approach allows developers to build and run applications without managing server infrastructure. Popular platforms such as AWS Lambda and Azure Functions enable this paradigm by providing event-driven execution of code.
Using serverless architecture with REST APIs can lead to better resource management. Developers can scale their applications automatically in response to varying traffic demands. This not only reduces costs but also simplifies deployment.
However, it introduces challenges as well. Debugging and monitoring can be more complex in a serverless context. Moreover, vendor lock-in can become a concern as applications grow.
The End
In an era where digital transformation drives the advancement of businesses and technologies, understanding HTTP REST APIs holds critical importance. This conclusion synthesizes the topics discussed throughout the article and underscores the vital aspects of REST APIs that IT professionals, cybersecurity experts, and students must grasp.
Recap of Key Points
REST APIs represent a significant architectural style for web services. Some key points covered in the article include:
- Core Principles of REST: REST relies on a set of principles, including statelessness, cacheability, and a layered system. Each principle helps in creating scalable and efficient APIs.
- HTTP Methods: Different HTTP methods, notably GET, POST, PUT, PATCH, and DELETE, are integral for interacting with resources. Understanding these methods is essential for seamless API operations.
- Error Handling: Knowing the common HTTP status codes and the importance of informative error responses ensures that APIs function smoothly and that errors are communicated effectively to the users or developers.
- Authentication and Security: The implementation of OAuth 2.0, API keys, and SSL/TLS helps in protecting sensitive data and ensuring secure communications between clients and servers.
- Versioning and Best Practices: Strategies for versioning APIs and adhering to best practices in API design can greatly enhance usability and system maintainability.
- Real-World Applications and Future Trends: Practical applications case studies illustrated the significance of REST APIs, along with future trends like GraphQL and serverless architectures shaping the API landscape.
Final Thoughts
The intricate framework of REST APIs demands not only technical knowledge but also strategic thinking. As REST continues to evolve, staying informed about best practices is vital. This knowledge enables developers to build robust interfaces that meet dynamic business needs and adhere to user experience standards. Furthermore, understanding the emerging trends will empower professionals to leverage these technologies effectively in their projects.